A dart is a programming language that support all the concepts of object oriented programming such as:
- Classes
- Objects
- Inheritance
- Polymorphism
- Interface
- Abstract class
The main goal of oops concepts in dart is to reduce programming complexity & can execute many tasks at once.
Let’s have a brief introduction of dart oops concepts one by one
1. Classes
Classes are a user defined datatype that describe how an object should behave.
A dart class is a blueprint by which we can create an object to access properties members of a class.
A class in dart can contain:
- fields.
- Methods (<gettter>/<setter>).
- Constructors.
- User Defined Methods/functions.
Syntax of dart class:
class class_name{ <field> <getter/setter> methods or functions <constructor. }
Example below
2. Object
A object is an Instance of a class, so as you know, A Class is a blueprint from which object are created.
A dart object is a logical entity & Physical entity.
Object in dart can be easily understood by taking real-life object such as : Pen, Table, Car, Table etc.
In Dart Object of a class is created by below syntax:
Syntax:
Class_name object_name = Class_name();
Note: In dart there is no need to use new keywork to create a object.
For Easy understanding, class & object in dart let’s take a example of a car.
A Car has a brandName, model_no, color, price, topSpeed, Therefore we have various brands of cars & each car will have a individual properties like name, color and all…
Therefore here each car is an object that is created by using a class.
Dart Class & Object Example – OOPS
Dart Car Program
class Car{ // data members String? brandName; String? modelNo; String? color; int? speed; int? price; //constructor Car(String brandName,String modelNo,String color,int speed,int price){ this.brandName = brandName; this.modelNo = modelNo; this.color = color; this.speed = speed; this.price =price; } } void main(){ print("Hello World"); //creating call object by passing value using parameter constructor Car audi = Car('Audi','A6','RED',300,5000000); // using object we can access class properties using dot . operator print('Name: ${audi.brandName}'); print('Model No: ${audi.modelNo}'); print('Car Color: ${audi.color}'); print('Car Speed: ${audi.speed}'); print('Car Price ${audi.price}'); }
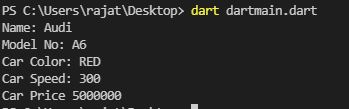
Dart program to calculate age using data of birth
We will create a class, that will calculate & find the exact age of a person using data of birth.
Here is below dart class & object program, we have 3 properties: datatype, constructor, & a method.
- dataType: Used to hold the data in variable eg: String webSite = ‘Proto Coders Point‘;
- constructor: we have parametrized constructor that except 2 parameter (name & date of birth).
- method: That calculate age using date_of_birth(dob) & print the age on console.
Dart code below:
void main(){ print("Hello World"); //create a object by passing value to constructor of class. AgeFinderClass myClassObject = AgeFinderClass('Rajat Palankar', DateTime(19XX, X, X)); // pass your dob here : (1999,5,6) //calling a method in class using object myClassObject.calculate(); } // dart class class AgeFinderClass { String name; DateTime? date_of_birth; // Constructor AgeFinderClass(this.name, this.date_of_birth); // Method. void calculate() { print('Name: $name'); var date_of_birth = this.date_of_birth; if (date_of_birth != null) { int years = DateTime.now().difference(date_of_birth).inDays ~/ 365; print('Your Age is: $years'); } } }
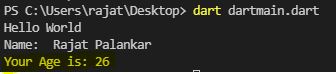
3. Inheritance in dart
Inheritance is one of the pillar of object oriented. Inheritance in dart, is very important concepts of OOPS, By using dart Inheritance, we can derive/acquire all the method & behaviour of a parent class.
Eg: Real Life Example
You dad, build house, earn money, has a car, bike & a business, Therefore you have all the rights to use all of his properties.
Inheritance represent the IS-A relationship also been called as parent-child relationship.
Why do we use Inheritance in dart
It’s been used to re-use the method & field of a parent class.
- Code Reusability
- Method Overriding (Polymorphism)
Syntax of dart class inheritance
class child-class-name extends parent_class_name{ //method & field }
Single Inheritance Example
In below example we have 2 class ‘Animal’ & ‘Dog’.
Dog acquire all the properties of Animal class by extending it,
Therefore, now Dog can use Animal class eat() method.
class Animal{ void eat(){ print('Eating....'); } } class Dog extends Animal{ void dark(){ print('Barking...'); } } void main(){ Dog d = Dog(); // here d is object of Dog Class d.eat(); // dog can use Animal class method d.dark(); }

4. Polymorphism in dart
In Dart or any OOPS concept a polymorphism is a feature that perform different action in different situation.
Polymorphism definition says:- A ability of an object to take on many forms, An example of polymorphism is when a parent class refer to child class object.
Real Life example
Let’s take an example to understand polymorphism. A person have many characteristics & perform different roles in his life, He can be a dad, husband, a employee or a business man, so as you saw, The same person plays different roles in different situation. This is the best & easiest way to understand what is polymorphism in dart.
Here poly means many, & morph means forms.
Dart polymorphism example
void main() { // parent class object creation Animal ma ; // using parent object, re-initialize subsclass object ma = Dog(); // class method print('Dog making sound: ${ma.makeSound()}'); ma = Cat(); print("Cat :- "+ma.makeSound()); ma = Bird(); print("Crow Making sound :- "+ma.makeSound()); } class Animal{ makeSound(){ return "Animal Sound is not Defined"; } } class Dog extends Animal{ @override String makeSound(){ return "bow wow"; } } class Cat extends Animal{ //no sound method declared // so it will take sound property from parent class "Animal" } class Bird extends Animal{ @override String makeSound(){ return "AWW! AWW!"; } }
In above code, we have a super class i.e Animal which has method makeSound(), Then we have a sub class i.e Dog, Cat, Bird. They have their own sound i.e method implemented to make respective animal sound.
In above code, I have defined sound method for Dog & Bird but not Cat, Therefore when cats makeSound() method is class, it will refer it parent method i.e. superclass Animal & makeSound() “sound is not defined”.
Basic Dart Programs to learn dart
Java OOPS Concepts – easy understanding of oops concepts