Hi Guys, Welcome to Proto Coders Point.
In the Dart tutorial (Article), We will learn a basic program every beginner programmer should know, i.e. How to convert string character to ASCII value in dart programming language(code unit).
Introducation:
What is ASCII value?
ASCII stands for “American Standart code for informaton exchange”. Is used for character encoding standard for electronic communication.
In simple words ASCII is a technique used to get ASCII number of a character.
Here is a ASCII value chart for you for reference.
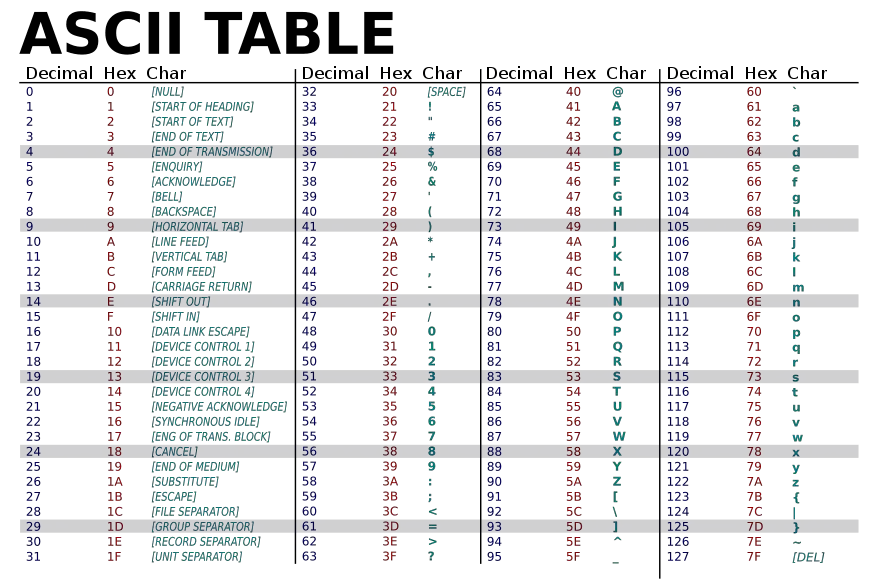
Eg: Suppose you want ascii value of character capital ‘R’, you can check above ascii chart we have R = 82, like wise for small letter ‘r’ = 114.
Ascii in dart – code unit in dart
In dart language, ascii is also know as code unit. In dart, each character is in UTF-16 form.
dart String class we have 2 methods to get code unit of a given character or also loop to string character to get all code units by each index of string value.
Dart codeUnitAt(index) & codeUnits methods
As i said above, String class in dart has 2 main method that can be used to convert string to ASCII in dart.
1. string.codeUnitAt(index)
The codeUnitAt method is used to get ascii/code unit of character in string using it’s index value.
int codeUnitAt(int index)
It accepts a integer parameter (i.e. index) and return a integer value of ASCII code.
As you all know that index value starts from ‘0’. i.e. 0 index is the first character of a string, 1 is second character of a string and so on. Have a look to example.
String name = "PROTO CODERS POINT";
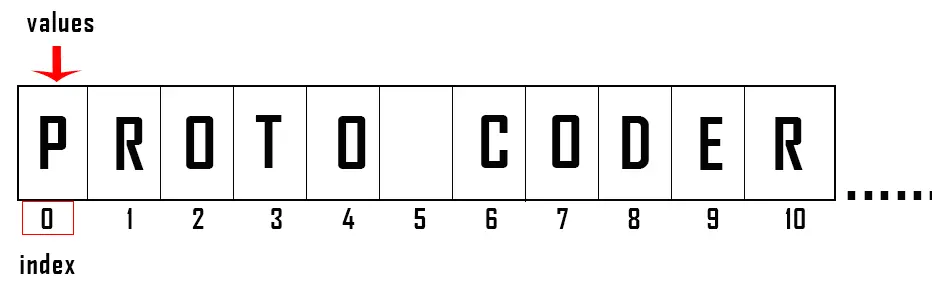
so if you want to convert index 0 position from string, then you can simple do it by:-
name.codeUnitAt(0); // here 0 is index value.
1. Dart code for converting character to ascii value
import 'dart:io'; void main() { String ch = 'R'; print(' ASCII value of ${ch[0]} is ${ch.codeUnitAt(0)}'); }
output
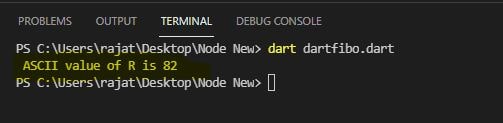
2. Looping through all character in string.
import 'dart:io'; void main() { String name = 'PROTO CODERS POINT'; //loop to each character in string for(int i = 0; i <name.length; i++){ print(' ASCII value of ${name[i]} is ${name.codeUnitAt(i)}'); } }
Output
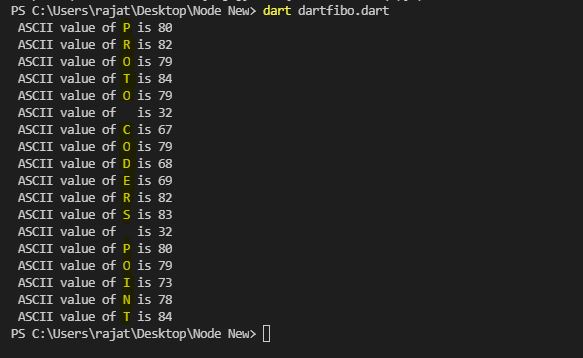
2. string.codeUnits
The string.codeUnits is used to get all the ascii value / code units of a given string & it returns code unit(ascii value) in list.
defined as:
List codeUnits
To get list of code unit(ascii value) of a given string simply pass the string to this method.
Eg: String name =''RAJAT";
print("ASCII list of given string ${name.codeUnits}.
import 'dart:io'; void main() { String name = 'PROTO CODERS POINT'; //returns list of code unit value. print('Code Unit list: ${name.codeUnits}'); }
output
