Hi Guy’s Welcome to Proto Coders Point. In this article let’s revise data structures and algorithms for interviews, basically will revise data structure interview questions that will be surely asked in any coding interview.
Data Structure quick revision to crack interview
1. What is Data Structure?
Data Structure simple means organizing, retrieving or storing of data in a specialized format, Basically data’s can be arranged in many ways like mathematical or logical arrangement, so technic of storing and retrieving of data is called as Data Structure.
2. What is Algorithms?
A technic in solving a given problem in a sequential steps performed on data is called algorithm.
3. Types of Data Structures
Data Structure are classified into two types:
- Primitive.
- Non-Primitive.
Example of Primitive data structure: includes byte , short , int , long , float , double , boolean and char.
Example of Non- Primitive data structure such as Array, Linked list, stack, Classes.
4. Classification of Data Structure
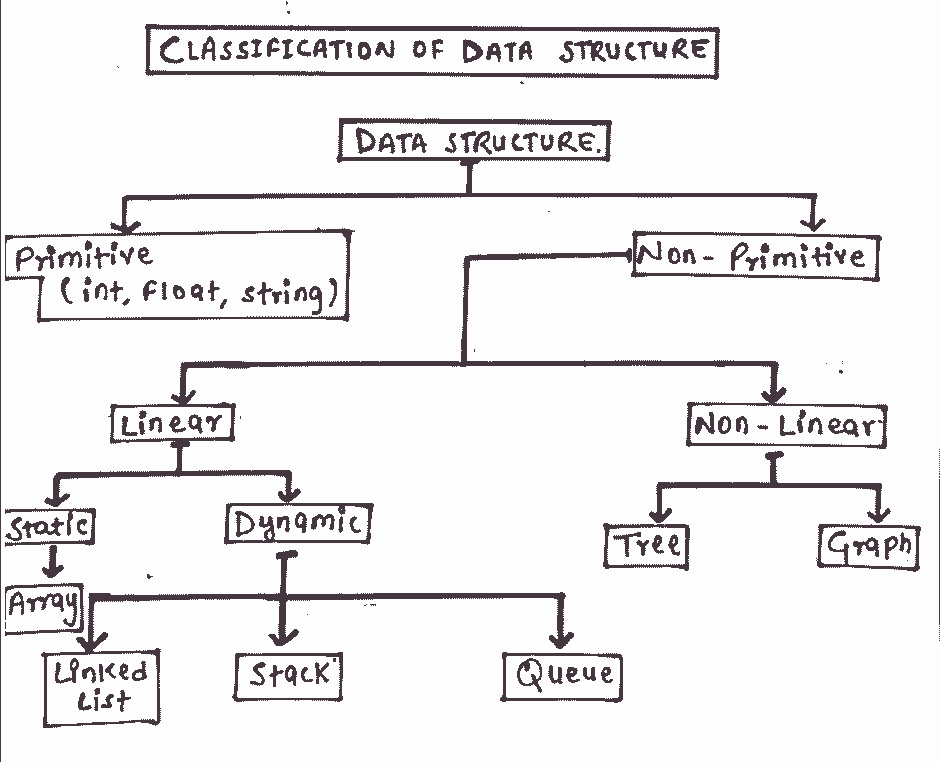
4. DS Array
Array is a type of linear data list, Using array we can store more then one data in a datatype using array. The data in array is been stored in a contiguous memory location.
Eg:
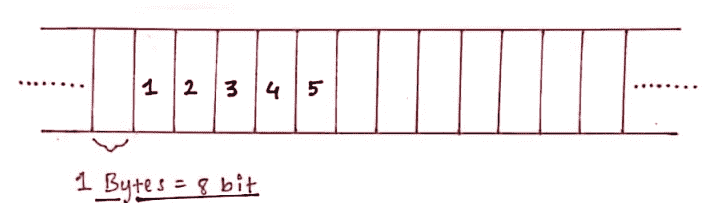
Types of array
One Dimensional Array: A Array with one subscript is called a 1D array.
Eg: int a[5];
Two Dimensional Array: A Array with two subscript is called a 2D array.
Eg: int a[3][3];
Multi Dimensional Array: A array with more then two subscript is called Multi Dimensional.
Eg: int a[5][4][2];
6. Data structures operations
There are for operations in data structure that plays major role:
Traversal :Accessing each record exactly once so that certain items in the record get processed.
Insertion : Adding data in the record.
Deletion: removing the data from the record.
Searching: Finding the location/position of particular item in the record with a given key value.
Sorting: Rearranging the data in accending or decending order.
Merging: combining the two or more data record as a single record.
6. Different of algorithm every developer should know
Searching algorithm
In DS, A search algorithms is a step-by-step procedure used to find the location if a data.
Types of Search algorithm in DS:-
1. Linear Search:
The linear search is also called as sequential search this is a method in data structure to search/find a element into the list in sequential order. Here each element is the list is check one by one until the match is been found.
complexity of linear search
C(n) = n/2.
2. Binary Search:
Here In Binary Search the element is been searched in the middle of the array list. This search technic is been implemented only for small list items.
Here first we need to check of the element are sorted or no, If not then first need to sort them in ascending order.
- First begin the search at middle of array.
- if the value search key is less then the item in the middle, then split it, and in next search only in first half.
- or
- if the value search key is greater then middle item, the split it, and search in second half.
- repeat it until you find the element.
complexity of binary search
C(n) = log2n
And many other searching method like:
Interpolation Search
Jump Search
Exponential Search