Hi Guys, Welcome to Proto Coders Point.
I have Already Made a tutorial on Flutter Default Bottom Navigation Bar Check here.
In this Tutorial, we will Implement Flutter Fancy Bottom Navigation Bar animation using android-studio to write flutter Dart codes.
Then, Check out the below Image that shows you how exactly does the flutter Fancy Bottom Navigation Bar looks like.
FANCY Flutter BOTTOM Navigation Bar AMINATION
Demo
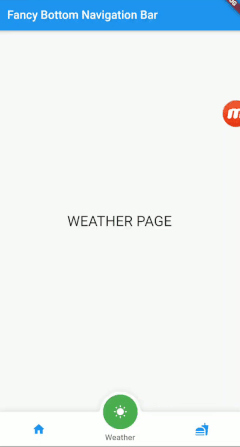
Instead, you’re switching out a over all group of content within a single page to give the appearance that the page is changing.Here is a illustration to visually describe what we’re doing. Not that the overall “scaffolding”, as Flutter calls it, stays the same and the contents within the page change.
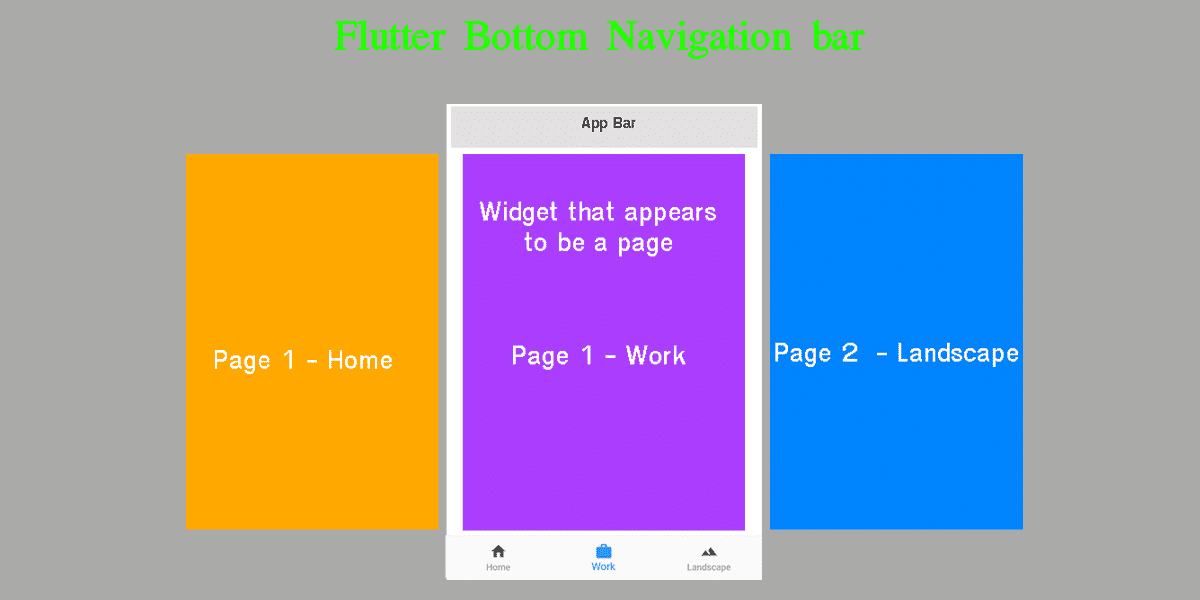
Creating Flutter Project in android Studio
As usual you need to create a new Flutter Project to implement the above.
File >New > New Flutter Project
First We need to start by changing the MyApp
widget in main.dart to be a Stateful widget. We need to do this because we will be storing which tab the user is currently on within our state.
Not to Worry in our project we are simple calling StateFullWidget class from StatelessWidget
It might seem to be little confusing for beginner learn of flutter but no need to worry the complete code will given below in the end of this post.
Adding fancy_bottom_navigation dependencies into your project
Here on the right side of your android studio you will see your flutter project
Open the File called pubspec.yaml
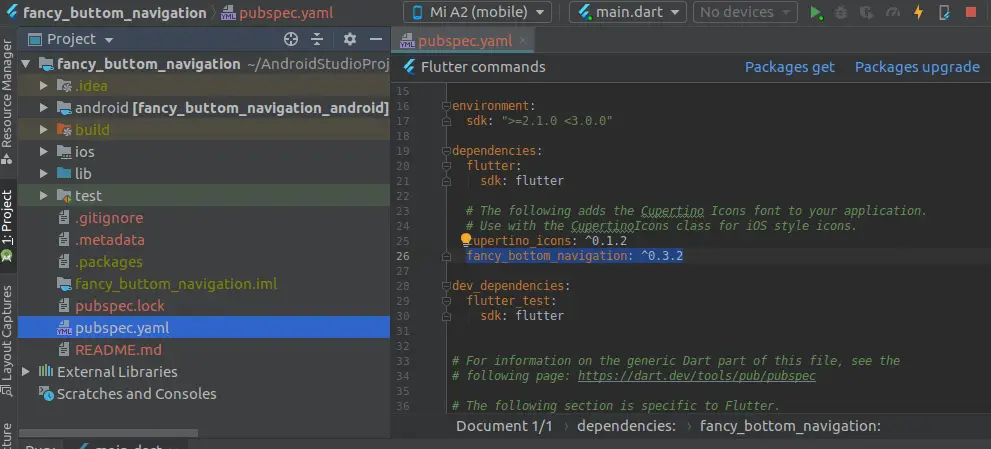
and copy paste below dependencies as show in the above image.
Dependencies
fancy_bottom_navigation: ^0.3.2
Importing Fancy Bottom Navigation Bar in main.dart file
Then, once you have added required dependencies in pubspec.yaml you need to import.
import 'package:fancy_bottom_navigation/fancy_bottom_navigation.dart';
Now, Add the above import statement into main.dart file on the top.
Creating 3 dart Pages into the project
As we are making use of Bottom Navigation bar with 3 Tabs, we need to create 3 different dart pages.
That changes when user clicked of particular bottom tabs
In our project we have Home(), Weather(),and food() page.
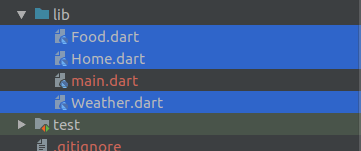
Right click on lib directory > New > Dart File
Then, all the required files are been added in to the project
Adding codes in dart file
Home()
import 'package:flutter/material.dart'; class Home extends StatelessWidget { @override Widget build(BuildContext context) { return Center( child: Container( child: Text( "HOME PAGE", style: TextStyle(fontSize: 25.0), ), padding: EdgeInsets.all(10.0), ), ); } }
Weather()
import 'package:flutter/material.dart'; class Weather extends StatelessWidget { @override Widget build(BuildContext context) { return Center( child: Container( child: Text( "WEATHER PAGE", style: TextStyle(fontSize: 25.0), ), padding: EdgeInsets.all(10.0), ), ); } }
Food()
import 'package:flutter/material.dart'; class Food extends StatelessWidget { @override Widget build(BuildContext context) { return Center( child: Container( child: Text( "FOOD PAGE", style: TextStyle(fontSize: 25.0), ), padding: EdgeInsets.all(10.0), ), ); } }
All the above page contain simple Text which is child of Container() widget in turn Container is the child if Center() widget,with simple show a text at the center of screen.
Introduction to Basic usage :
bottomNavigationBar: FancyBottomNavigation( tabs: [ TabData(iconData: Icons.home, title: "Home"), TabData(iconData: Icons.search, title: "Search"), TabData(iconData: Icons.shopping_cart, title: "Basket") ], onTabChangedListener: (position) { setState(() { currentPage = position; }); }, )
TabData
A TabData Contains a iconData -> which is used to show a icon on the tab with some title.
onTabChangeListener this function will simple keep track of which tab is currently open. and listens to which tab this click.
Optional Usage for customizing the Bottom Navigation Bar Tabs
initialSelection -> Defaults to 0
circleColor -> Defaults to null,
activeIconColor -> Defaults to null,
inactiveIconColor -> Defaults to null,
textColor -> Defaults to null,
barBackgroundColor -> Defaults to null, derives from
key -> Defaults to null<br/>
Theme Customizing

To learn more about this Flutter widget Library refer Official site
Complete Code of Flutter Fancy Bottom Navigation Bar
main.dart
Just copy paste the below lines of flutter code under main.dart file which will show you flutter fancy bottom Navigation bar at the bottom of the screen through which you can easily navigate to different pages being at the same page.
import 'package:flutter/material.dart'; import 'package:fancy_bottom_navigation/fancy_bottom_navigation.dart'; import 'Home.dart'; import 'Weather.dart'; import 'Food.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: HomePage()); } } class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { int currentPage = 0; final _pageOptions = [Home(), Weather(), Food()]; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Fancy Bottom Navigation Bar"), ), body: _pageOptions[currentPage], bottomNavigationBar: FancyBottomNavigation( circleColor: Colors.green, tabs: [ TabData(iconData: Icons.home, title: "Home"), TabData(iconData: Icons.wb_sunny, title: "Weather"), TabData(iconData: Icons.fastfood, title: "Food's") ], onTabChangedListener: (int position) { setState(() { currentPage = position; }); }, ), ); } }
Here, currentPage is set to 0 and pageOption is a array of different pages available
and body of flutter app is set to current page like pageOption[currentPage] with simple loads Home page when we run the app first
By making use of onTabChangedListener we are changing currentPage value to tab the tab value which is been clicked as that tabed page gets loaded on the screen.
Ok the main think is here i.e
The flutter widget package library have some Limitation
So total tabs that can be implemented using this widget is ranged from 2-4 tabs.
Comments are closed.