Hi Guys, Welcome to Proto Coders Point. In this flutter article let’s create a bottom navigation bar in flutter, A another way of creating awesome & beautiful bottom navigation bar by using salomon bar package.
Let’s get started
Add Salomon bottom bar as library
1. Install
To Install salomon navigation bar, add it in pubspec.yaml under dependencies section.
dependencies:
salomon_bottom_bar:
then hit pub get
button or run flutter pub get
.
2. Import salomon
To use it, any where within your flutter app you need to import salomon_bottom_bar.dart file.
import 'package:salomon_bottom_bar/salomon_bottom_bar.dart';
How to use salomon package in flutter for bottom navigation bar
Properties of salomon bottom bar
property | description |
items:[] | List of tab/ bottom bar in navigation. |
currentIndex: 0 | Active bar |
onTap:(position) | used to navigate to pages |
selectedItemColor: | Color of active tab/bar. |
unselectedItemColor: | Color of inActive tab/bar. |
duration: | The animation duration while switching tabs at buttom. |
curve: | active tab/bar curve shape. |
itemShape: | Salomon Bottom Bar Item Active tab shape. |
Syntax
bottomNavigationBar: SalomonBottomBar( currentIndex: _selectedTab, onTap: (position){ setState(() { _selectedTab = position; }); }, items: [ SalomonBottomBarItem( selectedColor: Colors.redAccent, title: const Text('Profile'), icon: const Icon(Icons.person) ), SalomonBottomBarItem( selectedColor: Colors.redAccent, title: const Text('Map'), icon: const Icon(Icons.map_outlined) ), ], ),
Complete Source Code
Flutter Salomon Bottom Navigation Bar
Output
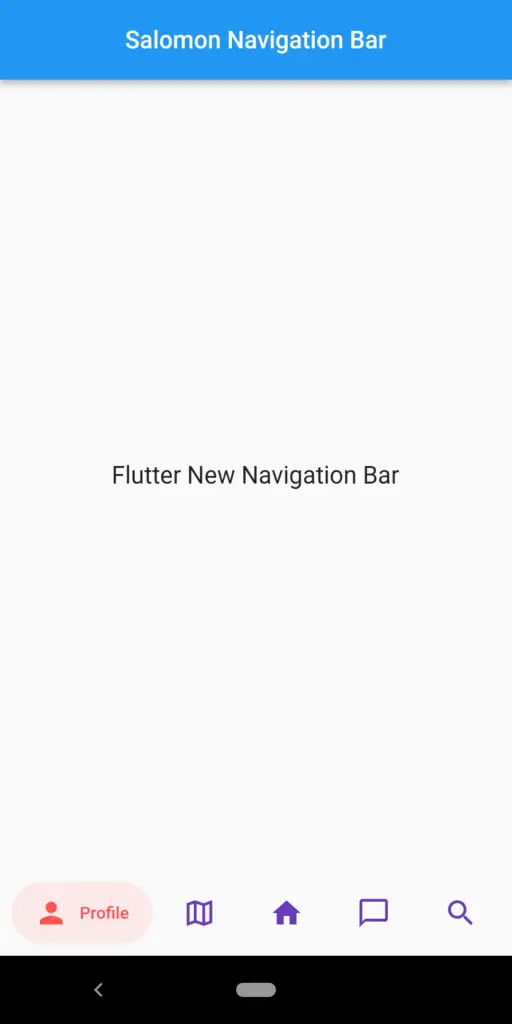
main.dart
import 'package:flutter/material.dart'; import 'package:salomon_bottom_bar/salomon_bottom_bar.dart'; void main() async { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), debugShowCheckedModeBanner: false, ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { var _selectedTab = 0; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text("Salomon Navigation Bar"), centerTitle: true, ), body: Center( child: Text('Flutter New Navigation Bar',style: TextStyle(fontSize: 20),), ), bottomNavigationBar: SalomonBottomBar( unselectedItemColor: Colors.deepPurple, currentIndex: _selectedTab, onTap: (position){ setState(() { _selectedTab = position; }); }, items: [ SalomonBottomBarItem( selectedColor: Colors.redAccent, title: const Text('Profile'), icon: const Icon(Icons.person) ), SalomonBottomBarItem( selectedColor: Colors.redAccent, title: const Text('Map'), icon: const Icon(Icons.map_outlined) ), SalomonBottomBarItem( selectedColor: Colors.redAccent, icon: const Icon(Icons.home), title: const Text("Home") ), SalomonBottomBarItem( selectedColor: Colors.redAccent, title: const Text('Chat'), icon: const Icon(Icons.chat_bubble_outline) ), SalomonBottomBarItem( selectedColor: Colors.redAccent, title: const Text('Search'), icon: const Icon(Icons.search) ) ], ), ); } }