Hi Guys, Welcome to Proto Coders Point, In this tutorial, we will create our own API server using DENO JS scripting language and we will also create a flutter app that will fetch image data from our Deno API server script and display the Images in flutter deno application by making use of Flutter Builder and ListView Builder.
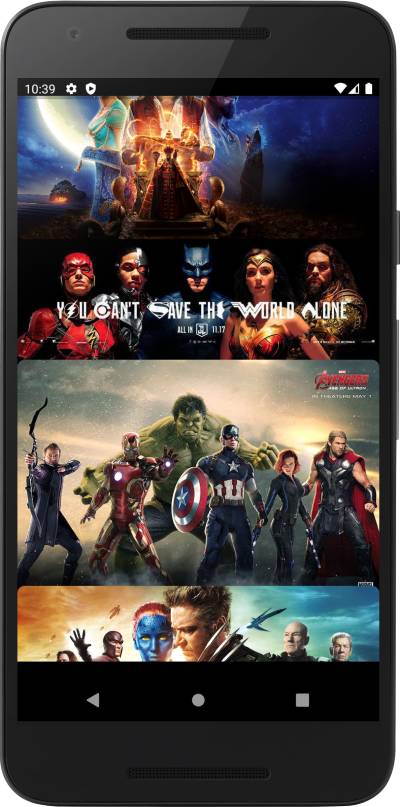
Brief Introduction on what is DENO ?
Deno is new Implementation of Node.js, We can call it as alternative of Node.js, The Creator of Deno and Node.js is the same “Ryan Dahl“, He it the original Creator of Node.js.
A Deno is a runtime JavaScript and TypeScript that is built on Version 8 of JavaScript engine and Deno is writter in Rust Programming language.
Creating API server using Deno Script | Deno js tutorial
Video Tutorial to create deno API
Step 1: Editor VSCode or Atom
I prefer to make use of vscode editor or Atom editor to write back-end scripts.
In my case i am using vscode editor.
Step 2: Create New project / New Folder
File > Open Folder (new folder) > “deno http server”
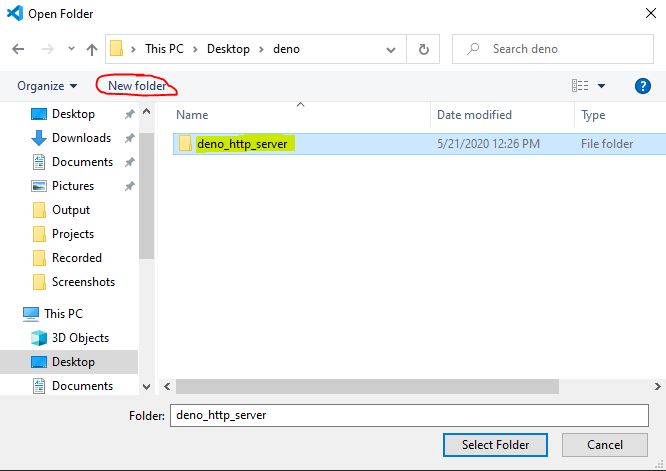
Then, Select Folder.
Step 3: Creating .ts files under deno http server folder
In vscode go to Files > New File here create 2 files and name it as index.ts and ImageData.ts
Step 4: index.ts deno script code and ImageData.ts
Then, In index.ts file copy paste the below lines of deno code
index.ts
// we need denotrain module to create routes and application import { Application, Router } from "https://deno.land/x/denotrain@v0.5.0/mod.ts"; import { imagedata } from "./imagesdata.ts"; const app = new Application({hostname:"192.168.0.7"}); // replace with you localhost IP Address const routes = new Router(); // lets create router to the api server app.use("/api",routes); routes.get("/imagedata",(ctx)=>{ return { // lets pass imagesdata interface here "imagedata":imagedata } }); app.get("/",(ctx)=>{ return "Hello World"; }); //this is the root of the server await app.run(); // then finally run the app press ctrl + ` to open terminal
ImageData.ts
interface Images{ url:String; } export const imagedata : Array<Images> = [ { "url":"https://images.wallpapersden.com/image/download/aladdin-2019-movie-banner-8k_65035_2560x1440.jpg", }, { "url":"https://thumbor.forbes.com/thumbor/960x0/https%3A%2F%2Fblogs-images.forbes.com%2Fscottmendelson%2Ffiles%2F2017%2F07%2FJustice-League-SDCC-Banner.jpg", }, { "url":"https://c4.wallpaperflare.com/wallpaper/869/847/751/movies-hollywood-movies-wallpaper-preview.jpg", }, { "url":"https://www.pixel4k.com/wp-content/uploads/2018/09/x-men-days-of-future-past-banner_1536361949.jpg", }, { "url":"https://images.wallpapersden.com/image/download/aquaman-2018-movie-banner-textless_63348_4000x1828.jpg", }, ]
then, make sure you have saved both the files.
Step 5: Run the Deno Script to start the server
open terminal in your editor,
How to open terminal in editor?
press ctrl + ` to open terminal
Then run the deno js script using below command
deno run --allow-net index.ts
After running the command, your deno script API data we be serving at your hostname as show in below screenshot.
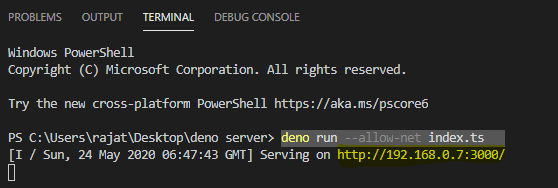
My Deno script Imagedata router url is http://192.168.0.7:3000/api/imagedata
Deno will server me json format of Image Urls that i will be using in my Flutter application to load those image URL.
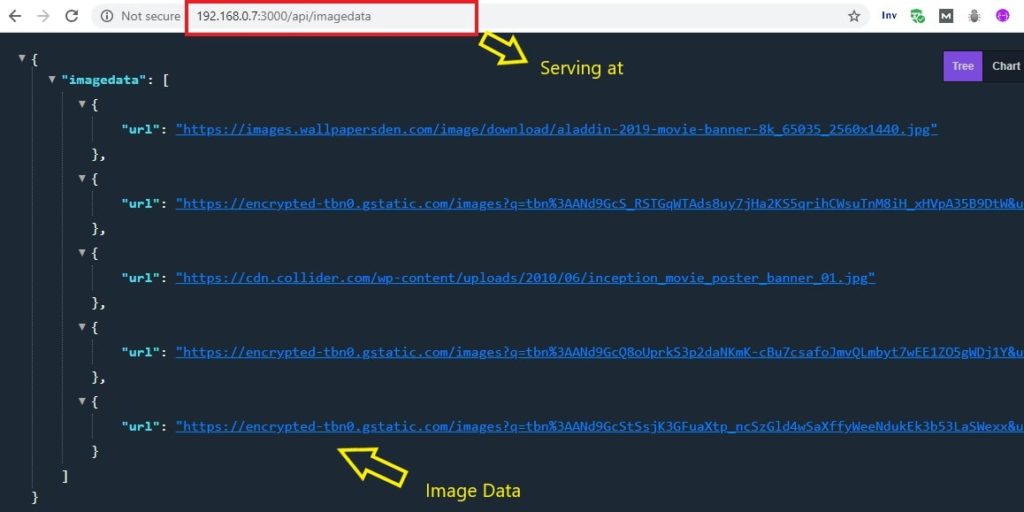
Now we are done with Creating our own API using Deno Script.
Creating Flutter Application to Display Image in ListView Builder | Flutter Deno Example
Flutter Deno Example Video Tutorial
Step 1: Create a new Flutter Project
I am making Android Studio as my IDE to build Flutter Application
create a new Flutter Project in android studio and name the project as “Flutter Deno Example” or anything as per your choice.
Step 2: Add flutter http dependencies
As we are we are making network call we need to use flutter http library to fetch data from our deno api server.
open pubspec.yaml file and add the flutter http library under dependencies section.
http: ^0.12.1 #kindly check official site for latest version
Step 3: Method to Fetch Image Data from Server
//function to fetch image data from deno script server fetchImageData() async{ final url = "http://192.168.0.7:3000/api/imagedata"; // replace with your IP url var res = await http.get(url); return jsonDecode(res.body)["imagedata"]; }
The above method is making use of http call to get data from deno script and return data in json decoded format.
Step 4 : Display image Data using Future builder and Listview widget
This is just a snipcode, Complete flutter code is given at the end.
FutureBuilder( future: fetchImageData(), builder: (context,snapshot){ if(snapshot.connectionState == ConnectionState.done) { if(snapshot.hasData) { return ListView.builder(itemBuilder: (context,index){ return ClipRRect( borderRadius: BorderRadius.circular(8.0), child: Image.network(snapshot.data[index]["url"],fit: BoxFit.cover,), ); },itemCount: snapshot.data.length,); } }else if (snapshot.connectionState == ConnectionState.none) { return Text("Something went Wrong"); } return CircularProgressIndicator(); }, ),
In above Flutter code we are making use of:
FutureBuilder : Making use of FutureBuilder because loading/fetching data from server may take some time. Here snapshot will hold all the data received from server.
Until data from server is completely loaded we will display CircularProgressIndicator() and once data is received we will show the data using ListView.builder that has Image widget to load url.
Complete Flutter Source Code | Flutter Deno Example
Copy paste below lines of Flutter Deno Example Source in main.dart file.
main.dart
import 'dart:convert'; import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:http/http.dart' as http; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', debugShowCheckedModeBanner: false, theme: ThemeData( primarySwatch: Colors.blue, visualDensity: VisualDensity.adaptivePlatformDensity, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Container( margin: EdgeInsets.symmetric(vertical: 20.0), height: 200.0, child: FutureBuilder( future: fetchImageData(), builder: (context,snapshot){ if(snapshot.connectionState == ConnectionState.done) { if(snapshot.hasData) { return ListView.builder(itemBuilder: (context,index){ return ClipRRect( borderRadius: BorderRadius.circular(8.0), child: Image.network(snapshot.data[index]["url"],fit: BoxFit.cover,), ); },itemCount: snapshot.data.length,); } }else if (snapshot.connectionState ==ConnectionState.none) { return Text("Something went Wrong"); } return CircularProgressIndicator(); }, ), ); } } //function to fetch image data from deno script server fetchImageData() async{ final url = "http://192.168.0.7:3000/api/imagedata"; var res = await http.get(url); return jsonDecode(res.body)["imagedata"]; }
Done now your Flutter applications is ready to fetch data from your own deno API script.
Recommended Article
how to install deno js – Deno Hello World Example