Hi Guys, Welcome to Proto Coders Point, In this Flutter Tutorial we will create a app where user can select his desired theme either dark theme in flutter or light theme in flutter.
VIDEO TUTORIAL
Flutter Dynamic theme change using getX
Step 1 : Create a new Flutter project
Start your favorite IDE, In my case i am making user of ANDROID STUDIO to build Flutter project, you may use as per your choice.
Create a new Flutter project
IDE -> Files -> New > New Flutter Project -> give name -> give package name and finish
Step 2: Add GetX & Get Storage dependencies
Then, once your flutter project is been created, you need to add 2 required dependencies i.e. GetX & Get Storage in pubspec.yaml
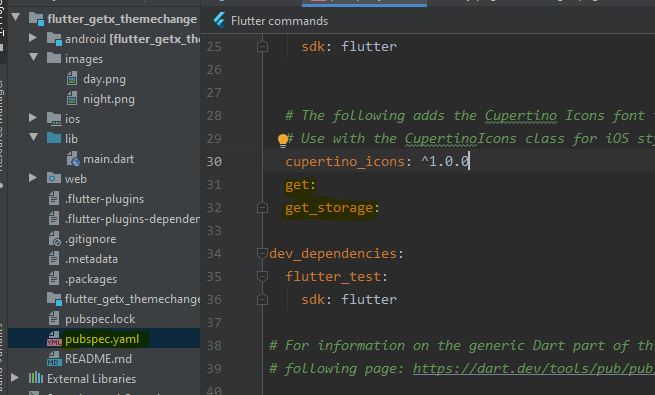
Step 3 : Adding images in Flutter project
create a package/folder in your flutter project structure, Right click on project -> New -> Directory (give name) and add image files in that folder.
After creating the directory you need to specify the path of the directory you have created in pubspec.yaml file so that your flutter project can access the images.
You can see in below screenshot, i have created folder by name images which has 2 images in it, and then in pubspec.yaml file i have gave the image path in flutter.
Step 4: The Code
If you face problem in understand below code, learn basic of Getx and Get Storage (link is below)
void main() async{ await GetStorage.init(); // before building the app you need to initialize GetStorage // so that we have access to use app data runApp(MyApp()); }
Then, Create a instance of GetStorage class
final appdata = GetStorage(); // instance of GetStorage
Now, using instance create a key-value pair
appdata.writeIfNull('darkmode', false);
as you see above, ‘darkmode’ is a key and false is value stored in it.
bool isDarkMode = appdata.read('darkmode'); // get data from get storage and store in a boolean variable
This isDarkMode will store either true or false, If true then it means, user have previously turned on dark mode before closing the app.
and depending on isDarkMode, we will set the theme of the app either dark mode or light mode.
A Switch Widget to turn on/off dark mode dynamically in flutter
Switch( value: isDarkMode , onChanged: (value) => appdata.write('darkmode', value), )
Complete Flutter code main.dart
This complete code will be posted on github repository, you may download it from there (link below)
import 'package:flutter/material.dart'; import 'package:get_storage/get_storage.dart'; import 'package:get/get.dart'; void main() async{ await GetStorage.init(); // before building the app you need to initialize GetStorage runApp(MyApp()); } class MyApp extends StatelessWidget { final appdata = GetStorage(); // instance of GetStorage @override Widget build(BuildContext context) { appdata.writeIfNull('darkmode', false); return SimpleBuilder( builder: (_) { bool isDarkMode = appdata.read('darkmode'); return GetMaterialApp( theme: isDarkMode ? ThemeData.dark() : ThemeData.light(), home: Scaffold( appBar: AppBar(title: Text("Getx Dynamic theme change"),), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Image.asset(isDarkMode ? 'images/night.png' :'images/day.png' ,width: 100,height: 100,), Switch( value: isDarkMode , onChanged: (value) => appdata.write('darkmode', value), ) ], ), ), ), ); }, ); } }
To make it easy i am making user of Getx & Get Storage package https://pub.dev/packages/get_storage/
Watch this video to learn basic of Get Storage and Getx Basic : https://www.youtube.com/watch?v=L7Zs4yuStsw&t=1s
Keep user logged in into the app :
clone project on github https://github.com/RajatPalankar8/flutter_dynamic_theme_change.git
Comment below for any queries
😀 Follow and support me:
🐦 Twitter: https://twitter.com/rajatpalankar
💬 Facebook: https://www.facebook.com/protocoderspoint/
💸 Instagram: https://www.instagram.com/protocoderspoint/
Be sure to ask for help in the comments if you need any. Suggestions for future Flutter tutorials are also very welcome! 🙂 For mobile application development keep learning freely from proto coders point Visit: https://protocoderspoint.com/