Hi Guys, Welcome to Proto Coders Point, In this Flutter tutorial article, We will learn how to use GetX Storage package (an alternative of shared preferences) in Flutter Application Development to keep users logged In.
In this example we gonna make use of Get Storage library GetX, to keep logged in users data.
Video Tutorial
What is Get_Storage Package in flutter?
In Flutter get storage is a very fast, extra light weight & synchronous key-value pair package that will help you to store app data in memory such as, if user is signed in, if yes, then all the details about the user.
The GetX Storage is built completly built using dart programming language & we can easily integrate with GETX framework of flutter.
This Flutter package to store user data is fully tested & supported on most of the famous operating system like, Android, iOS, Web, Windows & much more OS.
Which kind of data get_storage can handle/store?
Using Getx Storage we can store data type such as String, int, double, Map, and a list of values/arrays.
Installation and Basic of Get Storage Library
Check out this,
https://protocoderspoint.com/flutter-getx-storage-alternative-of-sharedpreferences/
Flutter Sharedpreferences Alternative GetX Storage | keep user loggedIn?
So let’s begin, with this small task in flutter app, to keep user signedIn using GetX Storage package (An Alternative of SharedPreferences)
OffCourse you need to create a new flutter project in your favourite IDE in my case i am using Android Studio as my IDE to develop flutter apps.
Create a new Flutter project and add the required 2 dependencies ( GetX & Get Storage ).
Then, to do this task you need 3 dart pages i.e
- main.dart
- LoginPage.dart
- DashBoardPage.dart
main.dart : Here we will check if the user is logged in or no
If the user is not Logged In then we will Navigate user using GetX to LoginPage.
If user is been Logged In then we can navigate user to DashBoardPage.
LoginPage.dart: In this page/screen user can enter username & password to signIn to the app, SignedIn user data will be stored using Get Storage library in key, value pair. & from successfull signIN we will navigate the user to dashboard page.
Dashboardpage.dart: Here we can simple show a username of the signed/logged in user and there is a button so that user can logout from the app.
Creating dart files in project
As stated above we need 3 dart files, So for that in lib directory of your flutter project create them.
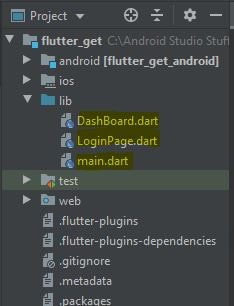
Codes – Login Form – Keep user logged in Using GetX Get Storage – Alternative of SharedPreferences
main.dart
In main page we are just checking if the user is been previously logged in or no
import 'package:flutter/material.dart'; import 'package:flutter_get/DashBoard.dart'; import 'package:flutter_get/LoginPage.dart'; import 'package:get/get.dart'; import 'package:get_storage/get_storage.dart'; void main() async{ await GetStorage.init(); runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return GetMaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: HomePage() ); } } class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { final userdate = GetStorage(); @override void initState() { // TODO: implement initState super.initState(); userdate.writeIfNull('isLogged', false); Future.delayed(Duration.zero,() async{ checkiflogged(); }); } @override Widget build(BuildContext context) { return Scaffold( body: SafeArea( child: Center( child: CircularProgressIndicator() ), ), ); } void checkiflogged() { userdate.read('isLogged') ? Get.offAll(DashBoard()) : Get.offAll(LoginPage()); } }
LoginPage.dart
UI Design
import 'package:flutter/material.dart'; import 'package:flutter_get/DashBoard.dart'; import 'package:get_storage/get_storage.dart'; import 'package:get/get.dart'; class LoginPage extends StatelessWidget { final username_controller = TextEditingController(); final password_controller = TextEditingController(); final userdata = GetStorage(); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(" Shared Preferences"), ), body: Center( child: Column( crossAxisAlignment: CrossAxisAlignment.center, mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( "Login Form", style: TextStyle(fontSize: 30, fontWeight: FontWeight.bold), ), Text( "To show Example of Shared Preferences Alternative using GetX Storage", style: TextStyle(fontSize: 20, fontWeight: FontWeight.bold), ), Padding( padding: const EdgeInsets.all(15.0), child: TextField( controller: username_controller, decoration: InputDecoration( border: OutlineInputBorder(), labelText: 'username', ), ), ), Padding( padding: const EdgeInsets.all(15.0), child: TextField( controller: password_controller, decoration: InputDecoration( border: OutlineInputBorder(), labelText: 'Password', ), ), ), RaisedButton( textColor: Colors.white, color: Colors.blue, onPressed: () { String username = username_controller.text; String password = password_controller.text; if (username != '' && password != '') { print('Successfull'); userdata.write('isLogged', true); userdata.write('username',username ); Get.offAll(DashBoard()); }else{ Get.snackbar("Error", "Please Enter Username & Password",snackPosition: SnackPosition.BOTTOM); } }, child: Text("Log-In"), ) ], ), ), ); } }
DashBoard.dart
import 'package:flutter/material.dart'; import 'package:flutter_get/LoginPage.dart'; import 'package:get_storage/get_storage.dart'; import 'package:get/get.dart'; class DashBoard extends StatelessWidget { final userdata = GetStorage(); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(" Shared Preferences"), ), body: Center( child: Column( crossAxisAlignment: CrossAxisAlignment.center, mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( "USER DATA", style: TextStyle(fontSize: 30, fontWeight: FontWeight.bold), ), Text( "NAME : ${userdata.read('username')}", style: TextStyle(fontSize: 20, fontWeight: FontWeight.w500), ), RaisedButton( onPressed: (){ userdata.write('isLogged', false); userdata.remove('username'); Get.offAll(LoginPage()); }, child: Text("LOGOUT"), ) ], ), ), ); } }
[…] 2: Add GetX & Get Storage […]