Hi Guys welcome to Proto Coders Point, In the Tutorial, we will learn about the Flutter widget class i.e Rich Text.
Introduction to Flutter RichText widget
In Flutter, RichText is used to display multiply text styles.
Here, the text to be displayed is done using TextSpan objects.
The text which is displayed in the rich text widget should be styled explicitly. you can set a DefaultTextStyle using the current BuildContext to provide defaults. To learn more on how to style text in RichText Widget. see the documentation for TextStyle.
Implementation of Flutter Rich Text Example
ok, So we now know the basic of the above Flutter Text Widget. It’s time to implement the widget in our project. For that, we need to first create a new Flutter Project or you can continue with your existing one.
File > New > New Flutter Project
If you can’t find any option to create a flutter project that might be because you have not installed the flutter plugin into your android studio.
Check out this post to install flutter in android studio.
How to install flutter in android studio
Example of Flutter RichText with Code.
Code
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: HomePage(), ); } } class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Rich Text Demo"), ), body: Padding( padding: const EdgeInsets.all(8.0), child: RichText( text: TextSpan( text: 'The harder you work for something,', style: TextStyle(fontSize: 15.0, color: Colors.lightBlue), children: <TextSpan>[ TextSpan( text: ' The greater you\'ll feel when you achieve it', style: TextStyle( fontWeight: FontWeight.w600, fontSize: 20, color: Colors.red), ), ], ), ), ), ); } }
Result:
Here is another example of RichText Widget
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: HomePage(), ); } } class HomePage extends StatefulWidget { @override _HomePageState createState() => _HomePageState(); } class _HomePageState extends State<HomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Rich Text Demo"), ), body: Padding( padding: const EdgeInsets.all(10.0), child: Column( children: <Widget>[ Text( "Motivational Quotes", style: TextStyle(fontWeight: FontWeight.w800, fontSize: 25.0), ), RichText( text: TextSpan( text: 'The harder you work for something,', style: TextStyle(fontSize: 15.0, color: Colors.lightBlue), children: <TextSpan>[ TextSpan( text: ' The greater you\'ll feel when you achieve it', style: TextStyle( fontWeight: FontWeight.w600, fontSize: 20, color: Colors.red), ), ], ), ), Text( "Sentance 2", style: TextStyle(fontWeight: FontWeight.w900, fontSize: 30.0), ), RichText( text: TextSpan( text: 'Your limitation', style: TextStyle(fontSize: 15.0, color: Colors.lightBlue), children: <TextSpan>[ TextSpan( text: '— it\'s only your', style: TextStyle( fontWeight: FontWeight.w600, fontSize: 20, color: Colors.yellow[900]), ), TextSpan( text: ' IMAGINATION.', style: TextStyle( fontWeight: FontWeight.w500, color: Colors.green, fontSize: 25), ) ], ), ), ], ), )); } }
Output – Result
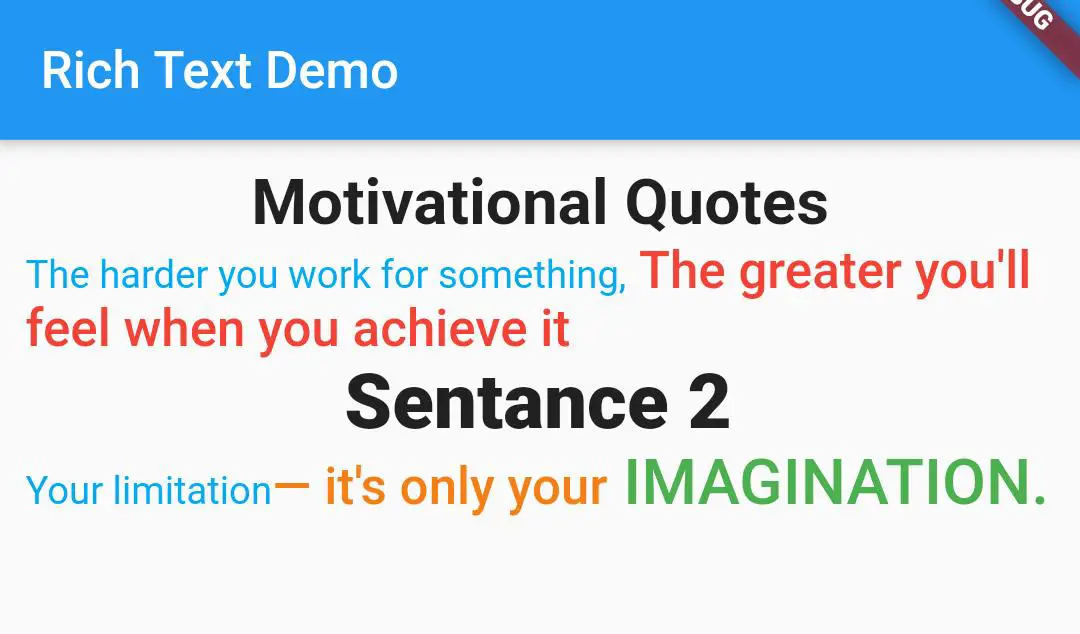
Flutter RichText Widget is very much helpful when you want to show Text View in different TextStyle.
as in the above Code or image, I have made the Text font too big in the same sentence this you can’t Achieve using a normal Text Widget.
By you RichText in a flutter, we can Design the UI to show text to users as we want for example Making the first letter to be bigger in the sentence.
To learn more about flutter Widget visit the official Flutter site here
[…] Flutter Rich Text Widget Example […]