Hi Guys, Welcome to Proto Coders Point, In this Flutter Tutorial we will implement Flutter Splash Screen and showing a loading effect using Flutter Spinkit.
What is Splash Screen?
In any Mobile or Web Application a Splash Screen is the first screen that is visible to the user when it app is been launched… Usually Splash Screen are used to show company logo and then launch the main screen of the application after some time.
There are many way to show a flutter splash screen. In this Flutter Tutorial we gonna learn the simplest way to show the Splash Screen using Timer class.
DEMO
Video Tutorial
So let’s begin implementing this in Flutter project without wasting any time.
Brief about Flutter Spinkit Library
The Flutter Spinkit package library is a collection of pre – animated loading indicator in flutter app development.
This Spinkit package library is been pre-animated with loading effect you just need to use those class wherever required.
How to add Flutter Spinkit package library?
Step 1: Add Spinkit dependencies in Pubspex.yaml file
To make use of spinkit library to show loading animation effect you need to add the library in your project.
dependencies: flutter_spinkit: ^4.1.2 // add this line
Step 2: Import the dart class where required
Then you need to import dart code in main.dart file to use the Spinkit library.
import 'package:flutter_spinkit/flutter_spinkit.dart';
Step 3 : Use the Spinkit widgets
SpinKitRotatingCircle( color: Colors.white, size: 50.0, );
There are many type of spinkit widget.
Here are the list of all the spinkit loading widget that you can easily use to show loading effect. Experiment them one by one and select any one.
SpinKitRotatingCircle(color: Colors.white) SpinKitRotatingPlain(color: Colors.white) SpinKitChasingDots(color: Colors.white) SpinKitPumpingHeart(color: Colors.white) SpinKitPulse(color: Colors.white) SpinKitDoubleBounce(color: Colors.white) SpinKitWave(color: Colors.white, type: SpinKitWaveType.start) SpinKitWave(color: Colors.white, type: SpinKitWaveType.center) SpinKitWave(color: Colors.white, type: SpinKitWaveType.end) SpinKitThreeBounce(color: Colors.white) SpinKitWanderingCubes(color: Colors.white) SpinKitWanderingCubes(color: Colors.white, shape: BoxShape.circle) SpinKitCircle(color: Colors.white) SpinKitFadingFour(color: Colors.white) SpinKitFadingFour(color: Colors.white, shape: BoxShape.rectangle) SpinKitFadingCube(color: Colors.white) SpinKitCubeGrid(size: 51.0, color: Colors.white) SpinKitFoldingCube(color: Colors.white) SpinKitRing(color: Colors.white) SpinKitDualRing(color: Colors.white) SpinKitRipple(color: Colors.white) SpinKitFadingGrid(color: Colors.white) SpinKitFadingGrid(color: Colors.white, shape: BoxShape.rectangle) SpinKitHourGlass(color: Colors.white) SpinKitSpinningCircle(color: Colors.white) SpinKitSpinningCircle(color: Colors.white, shape: BoxShape.rectangle) SpinKitFadingCircle(color: Colors.white) SpinKitPouringHourglass(color: Colors.white)
Flutter Splash Screen Example with Loading Animation using Spinkit library
In this Flutter Tutorial we gonna make use of Timer() which loads page2 after 5 second.
To do so you need two Flutter dart pages, I have create 2 dart pages namely main.dart and HomePage.dart
Creating a dart page
To create a new dart file lib (right click) > New > Dart File
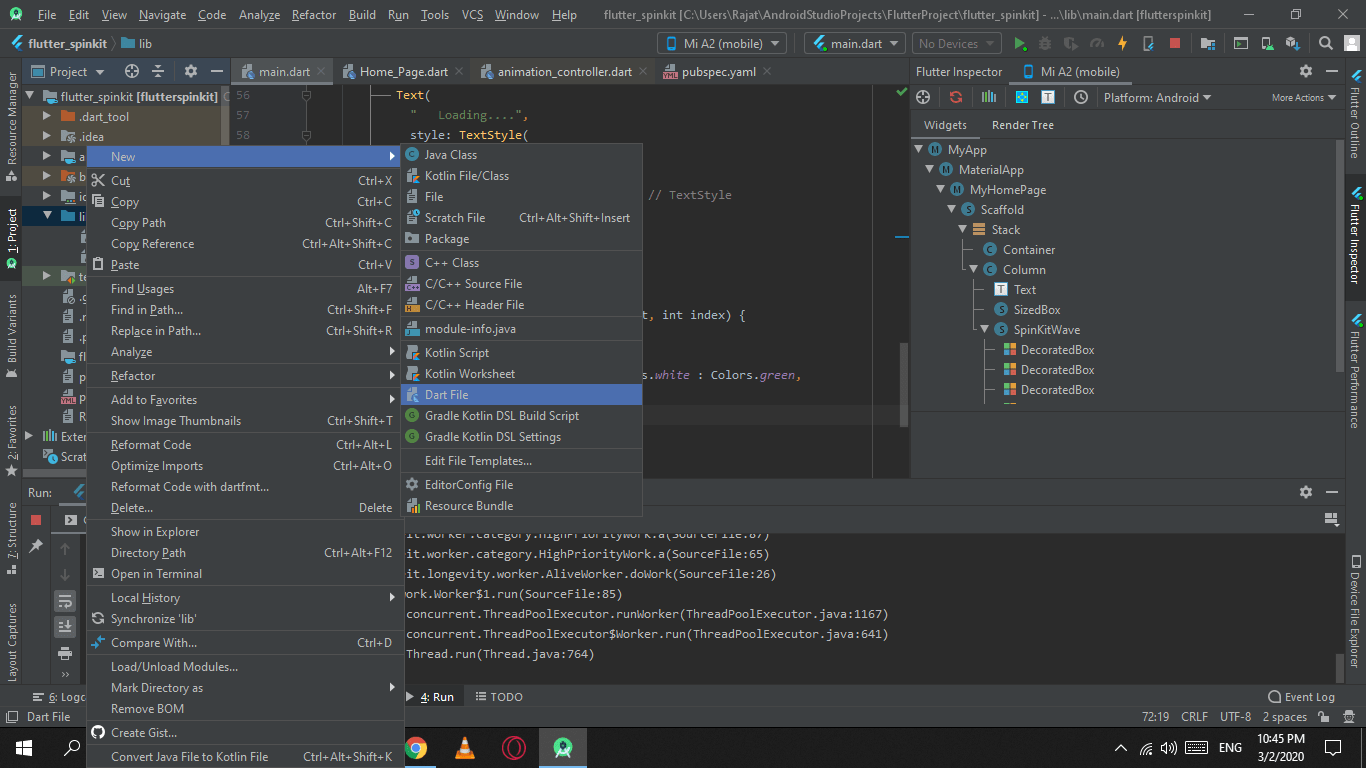
Then i have named it HomePage.dart
My Project Structure
Snippet Code of Timer() in main.dart file
@override void initState() { // TODO: implement initState super.initState(); Timer( Duration(seconds: 5), () => Navigator.push( context, MaterialPageRoute(builder: (context) => HomePage()), ), ); }
Here i will be waiting in main.dart file for 5 second to show Flutter loading Spinkit then after 5 second i will navigate from main.dart page —> HomePage.dart this will work like a Flutter Splash Screen.
Complete Code Flutter Splash Screen with Spinkit library.
main.dart
Copy paste the below lines of Flutter dart code in main.dart file
import 'dart:async'; import 'package:flutter/material.dart'; import 'package:flutter_spinkit/flutter_spinkit.dart'; import 'package:flutterspinkit/Home_Page.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override void initState() { // TODO: implement initState super.initState(); Timer( Duration(seconds: 5), () => Navigator.push( context, MaterialPageRoute(builder: (context) => HomePage()), ), ); } @override Widget build(BuildContext context) { return Scaffold( body: Stack( fit: StackFit.expand, children: <Widget>[ Container( decoration: BoxDecoration(color: Colors.redAccent), ), Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( " Loading....", style: TextStyle( color: Colors.white, fontSize: 20, fontWeight: FontWeight.bold), ), SizedBox( height: 25, ), SpinKitWave( itemBuilder: (BuildContext context, int index) { return DecoratedBox( decoration: BoxDecoration( color: index.isEven ? Colors.white : Colors.green, ), ); }, ), ], ), ], )); } }
The above code will simply show a loading indicator for 5 seconds and then load the new page.
HomePage.dart
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; class HomePage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Flutter Tutorial"), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( " This is an Example for Splash Screen and Loading Spin Kit Library") ], ), ), ); } }
This HomePage has simple text at the center of the screen.
[…] https://protocoderspoint.com/flutter-splash-screen-example-with-loading-animation-using-spinkit-libr… […]