This Web page represents a Form validation in a naughty way, Where the button moves right or left if the user has not filled the form and he tried to click on the button to submit.
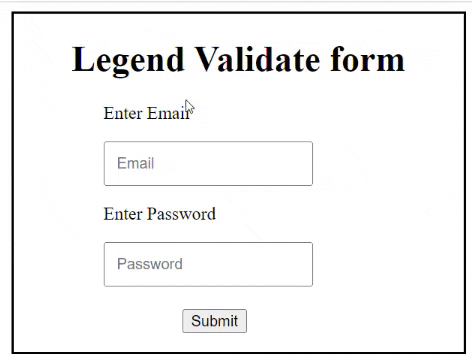
Source Code
<html> <head> <style> .outer { margin: auto; height: 300px; width: 400px; border: 2px solid black; position: relative } p { margin-left: 80px; } .in { margin-left: 80px; padding: 10px } #bt { margin-top: 20px; position: absolute; left: 150px; } #bt:hover { background: green; font-size: 13px; cursor: pointer; color: white; } </style> <script> function fa() { if (email.value == "" || pass.value == "") { f() document.getElementById("email").style.border = "2px solid red" document.getElementById("pass").style.border = "2px solid red" bt.value = "Pahila data dal bay" } else { document.getElementById("email").style.border = "2px solid green" document.getElementById("pass").style.border = "2px solid green" bt.value = "Ha thik ahe ata" bt.style.left = "120px"; } } flag = 1 function f() { if (flag == 1) { bt.style.left = "210px" flag = 2 } else if (flag == 2) { bt.style.left = "80px" flag = 1 } } </script> </head> <body> <div class="outer"> <h1 style="text-align:center">Legend Validate form</h1> <p>Enter Email</p> <input class="in" type="text" placeholder="Email" id="email" /> <p>Enter Password</p> <input class="in" type="password" placeholder="Password" id="pass" /> <br> <input type="submit" onmouseenter="fa()" onclick="alert('Form Sumbitted..!')" id="bt" /> </div> </body> </html>
Let’s break down the above code in parts and explain the code:
HTML Structure:
<head>
Section: In Head we have <style>
tag where we define CSS, and <script>
we have javascript code where we define function.
CSS Styling:
The CSS styles define the appearance of the form components.
.outer
: Represents the outer container with specific styling like margin, height, width, border, and relative positioning.p
: Applies styling to paragraphs within the form..in
: Applies styling to input elements within the form.#bt
: Applies styling to the submit button with specific positioning, margin, and hover effects.
JavaScript:
The <script>
tag includes JavaScript functions that add user event to the form like user hover over button to click it.
fa()
: This function is called when the mouse hover on the submit button (onmouseenter
). It checks if the email and password fields are empty. If either is empty, it calls the function f()
and changes the border color of the corresponding input fields to red. It also updates the button text.
f()
: This function is used to toggle the position of the submit button (#bt
) between two positions. It is invoked when the email and password fields are empty.
HTML Body:
Form Elements:
<div class="outer">
: Represents the outer container of the form.<h1>
: Displays a heading for the form.<p>
: Represents paragraphs for the email and password input fields.<input>
: Defines input fields for email and password with specific IDs (email
andpass
).<input type="submit">
: Represents the submit button with anonmouseenter
event calling thefa()
function and anonclick
event displaying an alert. The button has the IDbt
.
Summary:
In summary, This code is for fun and practicing HTML, CSS and JS for beginners by creating a form with a dynamic submit button that moves when form is empty and use tries to click on the button by hovering. The JavaScript functions are used for form validation, changing button positions, and updating styles based on user interactions. The form includes fields for email and password, and the submit button triggers JavaScript functions to validate the form and provide visual feedback to the user.