Hi Welcome to Proto Coders Point, In this tutorial, we’ll building a simple stylish Digital + analog clock using HTML, CSS, and JS. This clock features distinct hour, minute, and second hands will show a numerical indicators where will show current hours, minute & second as a hand of the clock, providing a visually appealing and functional timekeeping display as animation.
Have a look at below gif image how our clock will look
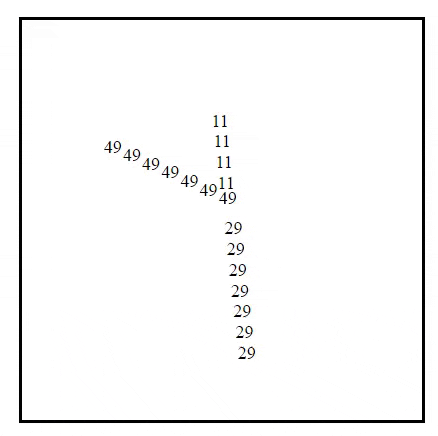
Source Code
<!DOCTYPE html> <html> <head> <title>Simple Clock with Lines</title> <script src="https://code.jquery.com/jquery-3.7.1.js" integrity="sha256-eKhayi8LEQwp4NKxN+CfCh+3qOVUtJn3QNZ0TciWLP4=" crossorigin="anonymous"></script> <style> #clock { position: relative; width: 400px; height: 400px; margin: 50px auto; font-size: 18px; border:solid } .hour-line, .minute-line, .second-line { position: absolute; transform-origin: 50% 100%; padding-bottom:15%; left:50%; } .hour-line { width: 6px; height: 60px; top: 90px; } .minute-line { width: 4px; height: 80px; top: 40px; } .second-line { width: 2px; height: 100px; top: 25px; } </style> </head> <body> <div id="clock"> <div class="hour-line" id="hourLine"> <div class="clock_number">00</div> <div class="clock_number">00</div> <div class="clock_number">00</div> <div class="clock_number">00</div> </div> <div class="minute-line" id="minuteLine"> <div class="clock_number">00</div> <div class="clock_number">00</div> <div class="clock_number">00</div> <div class="clock_number">00</div> <div class="clock_number">00</div> <div class="clock_number">00</div> <div class="clock_number">00</div> </div> <div class="second-line" id="secondLine"> <div class="clock_number">00</div> <div class="clock_number">00</div> <div class="clock_number">00</div> <div class="clock_number">00</div> <div class="clock_number">00</div> <div class="clock_number">00</div> <div class="clock_number">00</div> </div> </div> <script> function updateClock() { const now = new Date(); const hours = now.getHours() % 12 || 12; const minutes = now.getMinutes(); const seconds = now.getSeconds(); // Calculate angles for hour, minute, and second hands const hourAngle = (hours % 12) * 30 + minutes * 0.5; const minuteAngle = minutes * 6; const secondAngle = seconds * 6; // Rotate the lines based on the calculated angles $("#hourLine") .css("transform", `rotate(${hourAngle}deg)`) .children(".clock_number") .html(hours) .css("transform", `rotate(${-hourAngle}deg)`); $("#minuteLine") .css("transform", `rotate(${minuteAngle}deg)`) .children(".clock_number") .html(minutes) .css("transform", `rotate(${-minuteAngle}deg)`); $("#secondLine") .css("transform", `rotate(${secondAngle}deg)`) .children(".clock_number") .html(seconds) .css("transform", `rotate(${-secondAngle}deg)`); } // Update the clock every second setInterval(updateClock, 1000); // Initial call to display the clock immediately updateClock(); </script> </body> </html>
Above Source Code Explanation
HTML Structure
Let’s start with the HTML structure. The clock is contained within a <div>
element with the ID “clock” . Within this div, we have three child div’s which classes named a “hour-line,” “minute-line,” and “second-line,”. This 3 div.’s representing the hour, minute, and second hands of the clock, respectively. Additionally, within each line div, there are child div which classes names as “clock_number” to display numerical indicators.
CSS Styling
The CSS code is used to give styling and appearance to the clock design. The clock will have a square shape border, and each hand (hour, minute, and second) is represented by a digital number line with a specific dimensions and positions a the center of the square border. The transform-origin
property ensures that the rotation occurs around the base of each line.
JavaScript and jQuery
The JavaScript code is used to update the clock’s hands based on the current time. For this we have a function updateClock
that retrieves the current hour, minute, and second and perform calculation for each hand rotations in a proper angle for each hand. The angles we get is then applied using jQuery to rotate the lines. The numerical indicators are updated accordingly.
The setInterval
function is used to call the updateClock
function every second, ensuring that the clock hands are continuously updated in real-time.