Hi Guys, Welcome to Proto Coders Point.
In this Flutter Hive tutorial we learn how to implement flutter dark mode switch and store the user selected theme using HIVE database, So that whenever user revisit the app again the selected theme i.e dark theme or light theme should be loaded.
Learn basic of HIVE NOSQL database
Hive NoSQL Database Basic Video Tutorial
Hive database to save selected dark / light theme switch
Video Tutorial
Step 1: Create a Flutter Project
Start your favorite IDE, In my case i am making user of ANDROID STUDIO to build Flutter project, you may use as per your choice.
Create a new Flutter project IDE -> Files -> New > New Flutter Project -> give name -> give package name and finish
Step 2: Add Hive & Hive_Flutter Dependencies
In your project, open pubspec.yaml file and under dependencies section, add this 2 dependencies hive & hive_flutter
dependencies: hive: hive_flutter:
Step 3: Import Hive & hive_flutter
import 'package:hive/hive.dart';
import 'package:hive_flutter/hive_flutter.dart';
The Code Explanation
Initialize hive flutter
void main() async { await Hive.initFlutter(); await Hive.openBox('themedata'); runApp(MyApp()); }
In above snippet code, In main() method i.e. before loading your flutter UI content we are initializing flutter hive and then opening the box by using Hive.openBox so the later we can easily use Hive.box() and avoid async code
Using ValueListenableBuilder
We need to refresh the app when user make a switch in flutter theme(dark or light), So the efficient way to refresh is when there is change in HIVE database. Therefore to listen to the changes will make use of ValueListenableBuilder and box.listenable().
We need to refresh MaterialApp themeMode to either ThemeMode.dark else ThemeMode.light
class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return ValueListenableBuilder( valueListenable: Hive.box('themedata').listenable(), builder: (context,box,widget){ var darkMode = box.get('darkmode',defaultValue:false); return MaterialApp( themeMode: darkMode ? ThemeMode.dark : ThemeMode.light, darkTheme: ThemeData.dark(), home: Scaffold( body: Center( child: Switch( value: darkMode, onChanged: (value){ box.put('darkmode',value); }, ), ), floatingActionButton: FloatingActionButton.extended( onPressed: () {}, label: Text('Change Theme')), ), ); } ); } }
In above code we have a flutter switch, by using which a user can switch dark mode or to light mode theme, On Switch Changed() we are storing the switch data i.e. (true or false) in Hive box.
Here true mean : flutter dark theme
false mean: flutter light theme
and by using ValueListenableBuilder valueListenable properties we are listening to any changes in HIVE BOX, and if any change is listened then refresh the builder property, in our case we are MaterialApp Widget that get refreshed when changed happens in Hive data base box.
Complete Source Code – Hive database to save selected dark / light theme switch
main.dart
import 'package:flutter/material.dart'; import 'package:hive/hive.dart'; import 'package:hive_flutter/hive_flutter.dart'; void main() async { await Hive.initFlutter(); await Hive.openBox('themedata'); runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return ValueListenableBuilder( valueListenable: Hive.box('themedata').listenable(), builder: (context,box,widget){ var darkMode = box.get('darkmode',defaultValue:false); return MaterialApp( themeMode: darkMode ? ThemeMode.dark : ThemeMode.light, darkTheme: ThemeData.dark(), home: Scaffold( body: Center( child: Switch( value: darkMode, onChanged: (value){ box.put('darkmode',value); }, ), ), floatingActionButton: FloatingActionButton.extended( onPressed: () {}, label: Text('Change Theme')), ), ); } ); } }
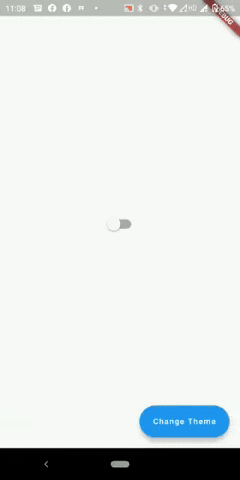