In JavaScript, Data can we stored in a form of object to store multiple values of complex data structure.
In JS, A Object can be created by {} curly braces, Here data are stored in key-value pair. Keys are always in String & value can be of any datatype.
JavaScript, Array & Object are the most frequently used to manage collection of data, it’s the easiest way to manage data while any event is been performed by users.
Convert array to collection(object) in JS
The best way to convert array to object in JavaScript
['a','b','c']
to
{
'0':'a',
'1':'b',
'2':'c'
}
1. JavaScript Example 1 – Convert array to object
const namesArr = ["Rajat","Proto", "Coders"]; function toObject(arr){ var ob = {}; for(var i =0;i<arr.length;i++){ ob[i] = arr[i]; } return ob; } var obj = toObject(namesArr); console.log(obj);
Output
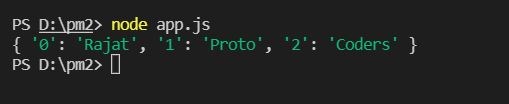
2. Array to Object using reduce method & functions
In today’s, JS we have a js method .reduce to iterate to array and remove empty holes from an array, so in below example will use array.reduce to convert array to object collections.
const namesArr = ["Rajat","Proto", "Coders"]; var obj = namesArr.reduce(function(result, item, i) { result[i] = item; return result; }, {}); console.log(obj);
At start of function we pass empty object, then augment the object by increment, and at the end of iteration of function, we get result as { '0': 'Rajat', '1': 'Proto', '2': 'Coders' }
.
Object to Array
3. Example 3 – Object to array JavaScript
To convery object to array in js, you can make use on Object.key and map to acheive it.
Let check how
var obj = { '0': 'Rajat','1':'Proto', '2': 'Coders', '3': 'Point' }; var result = Object.keys(obj).map((keys) => obj[keys]); console.log(result);
Output
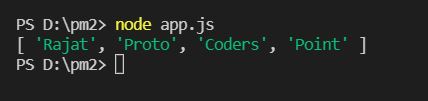