Hi Guys, Welcome to Proto Coders Point. In this flutter tutorial article will learn how to create custom TabBar in flutter.
I have already cover the basic on how to create tabbar in flutter, you can read it & implement yourself.
How to make a custom TabBar in flutter
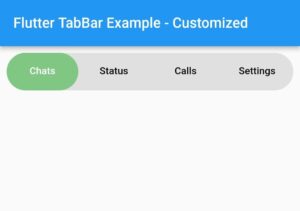
In flutter custom TabBar can be created outside AppBar i.e in body property of Scaffold widget (flutter tabbar without appbar).
To create custom tabbar, we can use tabBar widget to acheive customized flutter tabs designs.
Step to create custom tabBar in flutter app
1. Wrap scaffold widget with DefaultTabController & define length (number of Tabs).
2. In Body property of Scaffold widget will use Column widget.
3. Column widget will contain 2 children widget
1st Child -> Container will hold TabBar with custom design to tabbar using indicator property.
2nd Child -> Expanded widget that will have TabBarView which loads it’s children (i.e. different pages) depending on tabs user has selected.
Complete Source Code – To build custom TabBar in Flutter App
import 'package:flutter/material.dart'; import 'Chat.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', debugShowCheckedModeBanner: false, theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return DefaultTabController( length: 4, child: Scaffold( appBar: AppBar( title: Text("Flutter TabBar Example - Customized "), ), body: Padding( padding: EdgeInsets.all(8.0), child: Column( children: [ Container( height: 45, decoration: BoxDecoration( color: Colors.grey[300], borderRadius: BorderRadius.circular(25.0) ), child: TabBar( indicator: BoxDecoration( color: Colors.green[300], borderRadius: BorderRadius.circular(25.0) ) , labelColor: Colors.white, unselectedLabelColor: Colors.black, tabs: const [ Tab(text: 'Chats',), Tab(text: 'Status',), Tab(text: 'Calls',), Tab(text: 'Settings',) ], ), ), const Expanded( child: TabBarView( children: [ Center(child: Text("Chats Pages"),), Center(child: Text("Status Pages"),), Center(child: Text('Calls Page'),), Center(child: Text('Settings Page'),) ], ) ) ], ), ) ), ); } }