Hi Guys, Welcome to Proto Coders Point. In this Android Article will learn how to implement taking screenshot in your android application, Now every mobile device has a inbuilt feature by which users can easily capture screenshots.
Suppose you want to implement programmatically take screenshot in your android application as a special feature of your app then this article will help you.
Take Screenshot in android programmatically
Final Output of below code implementation
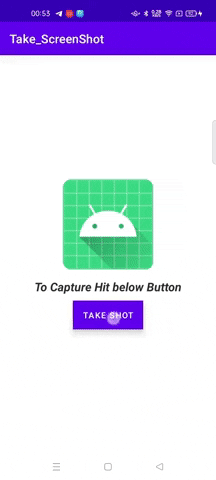
Implementation Step by Step
Step 1: Open or Create new Android Project
Create a new android project in android studio or open any existing android project where you want to implement screenshot taking feature.
Step 2: Add storage read & Write permission
As we are going to take screenshot and store the capture image in storage, we need to add permission to access storage, Therefore open AndroidManifest.xml file
Android > app > Manifest > AndroidManifest.xml
Within Manifest tag add below 3 storage permission
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="example.on.take_screenshot"> //add this 3 permission <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/> <uses-permission android:name="android.permission.MANAGE_EXTERNAL_STORAGE" /> </manifest>
Then next you need to enable requestLegacyExternalStorage
inside <application.> as show below:
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="example.on.take_screenshot"> //add this 3 permission <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/> <uses-permission android:name="android.permission.MANAGE_EXTERNAL_STORAGE" /> <application android:requestLegacyExternalStorage="true" //add this line android:allowBackup="true" ....... ....... ....... > </manifest>
Step 3: Layout design
Now, Let’s create a layout view or page of which our program will take screenshot programmatically.
activity_main.xml
Copy the below code in activty_main.xml file as UI design.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:id="@+id/parent" android:orientation="vertical" tools:context=".MainActivity"> <ImageView android:layout_width="150dp" android:layout_height="150dp" android:src="@mipmap/ic_launcher_round" android:layout_marginBottom="15dp"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="To Capture Hit below Button" android:textColor="#302F2F" android:textSize="20dp" android:textStyle="bold|italic" /> <Button android:id="@+id/takeshot" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="10dp" android:background="#11BFD6" android:text="Take Shot" /> </LinearLayout>
Step 4: Giving Life to UI, Working on MainActivity.java
Refer below MainActivity.java code , Comment are been added for better understanding while code go through.
package example.on.take_screenshot; import static android.content.ContentValues.TAG; import androidx.appcompat.app.AppCompatActivity; import androidx.core.app.ActivityCompat; import android.Manifest; import android.app.Activity; import android.content.pm.PackageManager; import android.graphics.Bitmap; import android.os.Build; import android.os.Bundle; import android.os.Environment; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.LinearLayout; import android.widget.Toast; import java.io.File; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.util.Date; public class MainActivity extends AppCompatActivity { Button take; private static final int REQUEST_EXTERNAL_STORAGE = 1; private static String[] permissionstorage = {Manifest.permission.WRITE_EXTERNAL_STORAGE, Manifest.permission.READ_EXTERNAL_STORAGE}; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); take = findViewById(R.id.takeshot); checkpermissions(this); // check or ask for storage permission take.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { //pass layout view to method to perform capture screenshot and save it. takeScreenshot(getWindow().getDecorView().getRootView()); } }); } protected File takeScreenshot(View view) { Date date = new Date(); try { String dirpath; // Initialising the directory of storage dirpath= MainActivity.this.getExternalFilesDir(Environment.DIRECTORY_PICTURES).toString() ; File file = new File(dirpath); if (!file.exists()) { boolean mkdir = file.mkdir(); } // File name : keeping file name unique using data time. String path = dirpath + "/"+ date.getTime() + ".jpeg"; view.setDrawingCacheEnabled(true); Bitmap bitmap = Bitmap.createBitmap(view.getDrawingCache()); view.setDrawingCacheEnabled(false); File imageurl = new File(path); FileOutputStream outputStream = new FileOutputStream(imageurl); bitmap.compress(Bitmap.CompressFormat.JPEG, 50, outputStream); outputStream.flush(); outputStream.close(); Log.d(TAG, "takeScreenshot Path: "+imageurl); Toast.makeText(MainActivity.this,""+imageurl,Toast.LENGTH_LONG).show(); return imageurl; } catch (FileNotFoundException io) { io.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } return null; } // check weather storage permission is given or not public static void checkpermissions(Activity activity) { int permissions = ActivityCompat.checkSelfPermission(activity, Manifest.permission.WRITE_EXTERNAL_STORAGE); // If storage permission is not given then request for External Storage Permission if (permissions != PackageManager.PERMISSION_GRANTED) { ActivityCompat.requestPermissions(activity, permissionstorage, REQUEST_EXTERNAL_STORAGE); } } }
Result
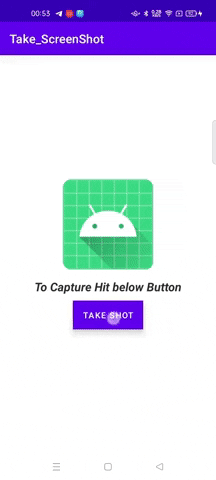