Hi Guys, welcome to Proto Coders Point in this Tutorial will we Learn How to Implement Flutter Slider with show Value.
What is Flutter Slider
A Slider in flutter app development is a Material Design , that is basically used in selecting a range of values.
A slider can be used to select from either a continuous or a discrete set of values. The default is to use a continuous range of values from min to max. To use discrete values, use a non-null value for divisions, which indicates the number of discrete intervals. For example, if min is 0.0 and max is 50.0 and divisions is 5, then the slider can take on the discrete values 0.0, 10.0, 20.0, 30.0, 40.0, and 50.0.
Here are the terms for the parts of a Flutter slider are:
- A “thumb”, is a Round shape that help the user to slide horizontally when user what to change in some values.
- “track”, is a horizontal line where the user can easily slide the thumb.
- The “value indicator”, which is a shape that pops up when the user is dragging the thumb to indicate the value being selected.
- The “active” side of the slider is the side between the thumb and the minimum value.
- The “inactive” side of the slider is the side between the thumb and the maximum value.
So Let’s Begin the Implementation of Flutter Code with show values.
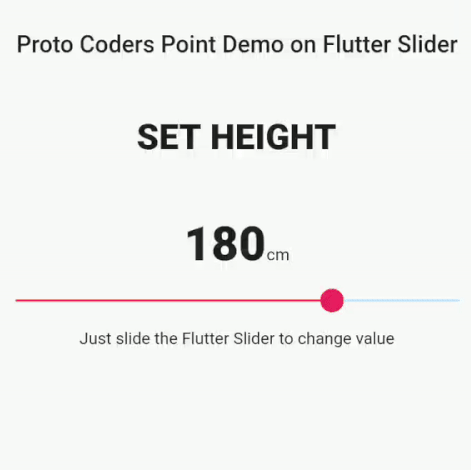
Implement Flutter Slider with show value
First of all with is very much common, your need to create a new Project or Open an existing project where you want to implement Flutter slider. So i am using android-stdio as my Development Toolkit.
New > New Flutter Project > Give a Name to the project and finish
Then, as soon as new Flutter project is been created, Flutter team have already set a default code that simple counts the number of time the floatingbutton is been pressed.
So, I recommend remove all the code persent in lib > main.dart file and copy paste the below code in it.
main.dart
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Slider', home: MyHomePage(title: 'Flutter Slider Demo'), ); } } class MyHomePage extends StatefulWidget { MyHomePage({Key key, this.title}) : super(key: key); final String title; @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( 'Demo on Flutter Slider', style: TextStyle(fontSize: 20.0, fontWeight: FontWeight.w500), ), ], ), ), ); } }
Note: The above code is not the complete working of Flutter project, it’s a way to clean all the default code.
The Final Code of complete working Flutter Slider is at the bottom on this Tutorial, you can just copy paste it in main.dart.
Slider comes with Various Properties
As i said Flutter Slider comes with Various Properties that help Flutter Developer to Customize the slider.
You can learn more about slider class properties navigating to official flutter page.
Slider( value: height.toDouble(), min: 80, max: 220, activeColor: Colors.pink, onChanged: (double value) { setState(() { height = value.round(); }); }, ),
- activeColor → Color The color to use for the portion of the slider track that is active.
- max → double – The maximum value the user can select.
- min → double – The minimum value the user can select.
- onChanged → ValueChanged<
double> Called during a drag when the user is selecting a new value for the slider by dragging. - value → double – The currently selected value for this slider.
Flutter Slider Not Moving
My Flutter app developer who are beginner in flutter are facing some problem while working with flutter slider that’s because :
Flutter Slider will not move if you not used setState method in OnChanged to change value.
onChanged: (double value) { setState(() { height = value.round(); }); },
so, you need to update value as user slide the slider. as shown in above snippet code.
Complete Code of Flutter Slider with show value change
main.dart
So, just copy paste the below complete lines of source code in to main.dart file to make Flutter slider work.
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Slider', home: MyHomePage(title: 'Flutter Slider Demo'), ); } } class MyHomePage extends StatefulWidget { MyHomePage({Key key, this.title}) : super(key: key); final String title; @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { int height = 180; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( 'Proto Coders Point Demo on Flutter Slider', style: TextStyle(fontSize: 20.0, fontWeight: FontWeight.w500), ), SizedBox( height: 50.0, ), Text( "SET HEIGHT", style: TextStyle(fontWeight: FontWeight.w900, fontSize: 30.0), ), SizedBox( height: 50.0, ), Row( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.baseline, textBaseline: TextBaseline.alphabetic, children: <Widget>[ Text( height.toString(), style: TextStyle(fontSize: 40.0, fontWeight: FontWeight.w900), ), Text("cm") ], ), Slider( value: height.toDouble(), min: 80, max: 220, activeColor: Colors.pink, onChanged: (double value) { setState(() { height = value.round(); }); }, ), Text("Just slide the Flutter Slider to change value") ], ), ), ); } }
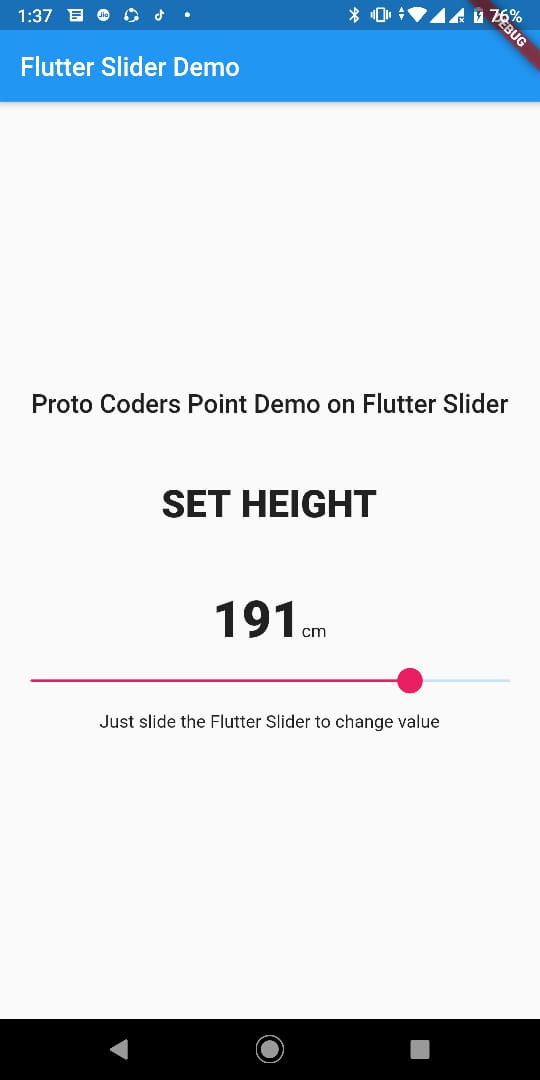
Recommended Articles on flutter
Image slider in flutter using Carousel Pro Flutter Library
flutter swiper – image slider/swiper in flutter
Flutter provider for beginners tutorial with app example
[…] Snippet code of Flutter Slidable […]