Hi Guys, Welcome to Proto Coders Point. In this article, we’ll know about the date-fns JavaScript Library for using Dates.
date-fns is a Modern date utility JavaScript library
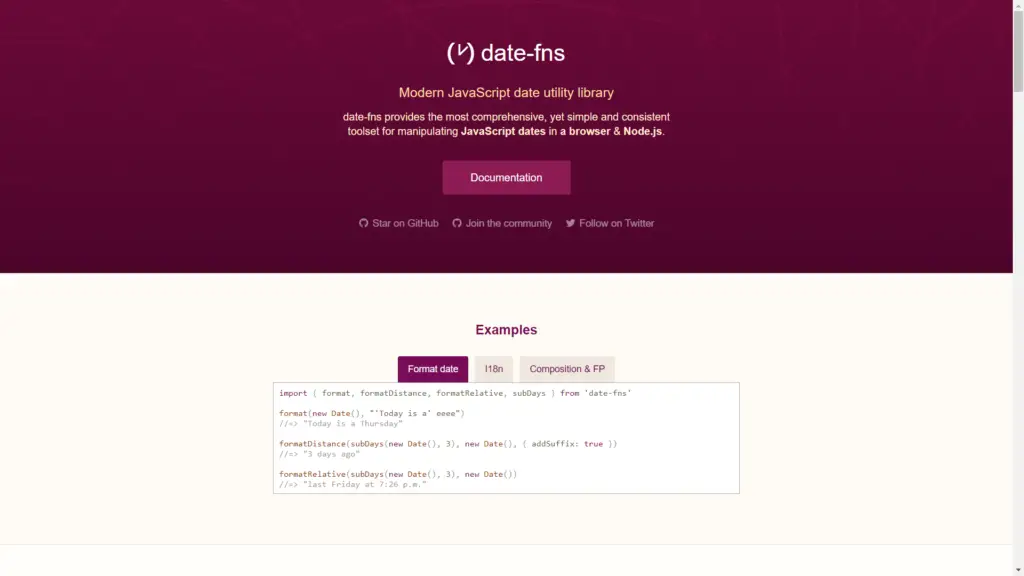
This is so lightweight and makes our life much easier. The first step is to install the package. Make sure you have Nodejs and IDE installed to work and practice this.
On that directory, run the command npm init and get started with the project.
Now let’s install date-fns
npm install date-fns
Create a javascript file naming according to you. I’ll name it Dates.js
Now we’re ready to go ahead into the package and use it effectively.
Let’s start with Formatting Dates
One of the basic usage. We use the format method here.
const { format } = require("date-fns"); console.log(format(new Date(), "dd-MM-yyyy")); console.log(format(new Date(), "dd/MM/yyyy HH:mm:ss")); console.log(format(new Date(), "PPPP"));
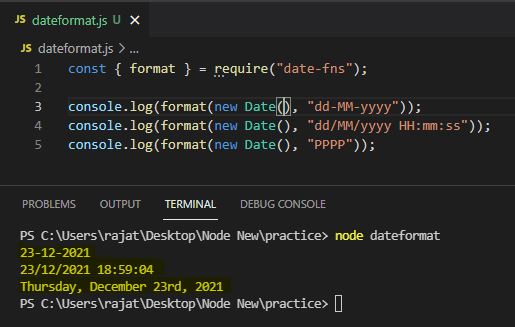
You can find more formats here
formatDistance – get age using dob
This helps in the comparison of dates and returns the gap value between the two dates. The program below will find your age!
How to find my age using date of birth
const { formatDistance } = require("date-fns"); const birthday = new Date("2004, 06, 13"); const presentDay = new Date(); console.log(`Age: ${formatDistance(presentDay, birthday)}`);
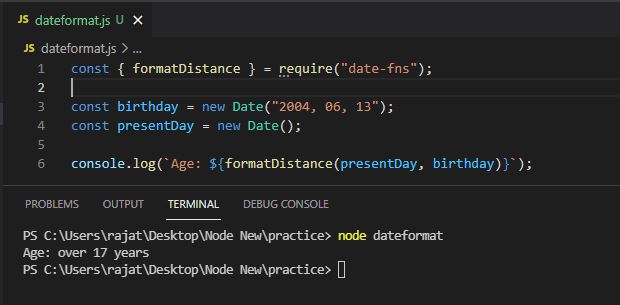
addDays
Add the defined years, months, weeks, days, hours, minutes, and seconds to the given date.
The method addDays is used to set up a deadline that is after a few days.
Simply, we can add days to any date to get the date of the day after some or a few days. It has many applications.
const { format, addDays } = require("date-fns"); const today = new Date(); // birthday after 6 days const birthday = addDays(today, 6); console.log(format(today, "PPPP")); console.log(format(birthday, "PPPP"));
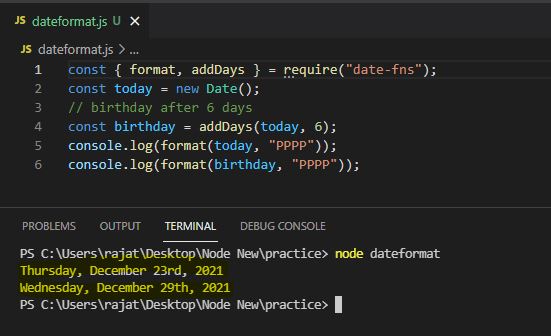
Invalid Dates
Some of the dates aren’t valid when you execute them for eg:- 30th February which is not a date mentioned in any year. So how do we check if the provided date is valid or not? Here we have a method for that too. We use isValid, It returns false if the argument is Invalid Date and true otherwise.
const { isValid } = require("date-fns"); console.log(isValid(new Date("2021, 02, 30")));
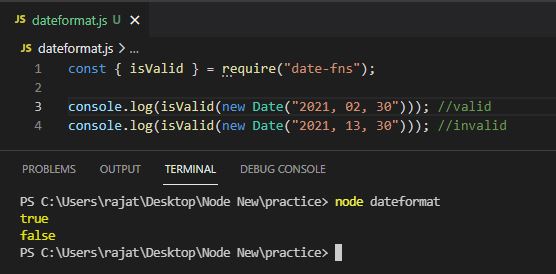
To switch this invalid behavior we have a parse method. This method parses the date that you have given and returns accurate results.
const { isValid, parse } = require("date-fns"); const invalidDate = parse("30.02.2021", "dd.MM.yyyy", new Date()); const validDate = parse("25.03.2021", "dd.MM.yyyy", new Date()); console.log(isValid(invalidDate)); console.log(isValid(validDate));
The above code won’t give us 30th February, it will print 1st March 2021.
Now, Let’s learn about some common helpers
isAfter – Tells you Is the first date after the second one?
// Is 21 January 2022 after 23 December 2021? var result = isAfter(new Date(2022, 1, 21), new Date(2021, 12, 23)) //=> true
isBefore – Is the first date before the second one?
// Is 21 January 2022 after 23 December 2021? var result = isAfter(new Date(2022, 1, 21), new Date(2021, 12, 23)) // result => false
isDate – Returns true if the given value is an instance of Date. The function works for dates transferred across iframes.
// For some value: const result = isDate('2022-02-31') //=> false
isEqual – Are the given dates equal?
// Are 21 Jan 2021 06:30:45.000 and 21 Jan 2021 06:30:45.500 equal? var result = isEqual( new Date(2014, 6, 2, 6, 30, 45, 0), new Date(2014, 6, 2, 6, 30, 45, 500) ) //=> false
Conclusion
We can’t talk about each and every method here, it takes days to learn about. But as you build projects, you can have a look at methods that you find useful in your problem. You’ve learned the essential methods here. Search the specific usage in its documentation.