Hi Guy’s Welcome to Proto Coders Point, In this flutter article let’s learn how to blur an image in flutter, Basically will learn how to apply blur filter to a Image Widget.
Blur Background Image in flutter
In order to give blur effect to a Image, We will make use of ImageFiltered
Widget with imageFilter:
property and apply ImageFilter.blur()
with X & Y axis Sigma on top of Image Widget as Parent widget.
ImageFilter.blur constructor
ImageFilter.blur( sigmaX: 10, sigmaY: 10 )
Complete Source Code – Apply Blur Background Image
import 'dart:ui'; import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(), debugShowCheckedModeBanner: false, ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( body: Stack( alignment: Alignment.center, children: [ SizedBox.expand( child: ImageFiltered( imageFilter: ImageFilter.blur( sigmaX: 10, sigmaY: 10 ), child: Image.network( 'https://media.istockphoto.com/id/1146517111/photo/taj-mahal-mausoleum-in-agra.jpg?s=612x612&w=0&k=20&c=vcIjhwUrNyjoKbGbAQ5sOcEzDUgOfCsm9ySmJ8gNeRk=', fit: BoxFit.fill, )), ), Image.network('https://media.istockphoto.com/id/1146517111/photo/taj-mahal-mausoleum-in-agra.jpg?s=612x612&w=0&k=20&c=vcIjhwUrNyjoKbGbAQ5sOcEzDUgOfCsm9ySmJ8gNeRk=') ], ) ); } }
Output
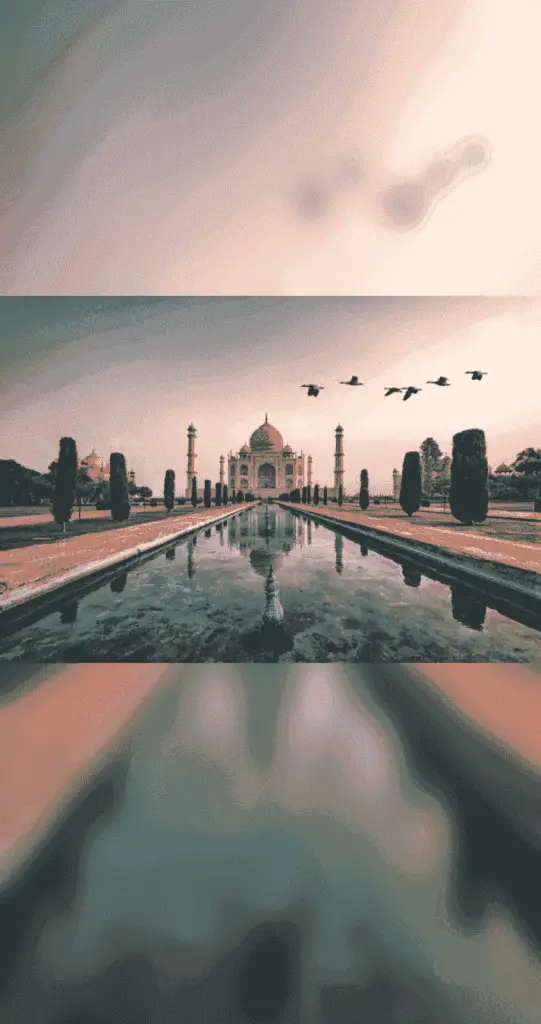