Hi Guys, Welcome to Proto Coders Point, this Flutter Tutorial is on how to add flutter admob native ads in flutter app.
So to show native admob ads in our flutter project, we will make use of a external library called flutter_native_admob package. Using which you can easily show cusomized native ads in your flutter android & ios application.
Brief about flutter native admob package
A Plugin that makes 70% work of developer to show admob ads into flutter project.
By using this plugin you can integrate firebase native admob ad. This plugin supports both Android & iOS platform.
Please Note: This package is not yet configured with Null safety in flutter, so to use this package your flutter project must use envirnment sdk less then 2.12.0.
environment: sdk: ">=2.8.0 <3.0.0"
What is Native ads? admob
A native advertising, also called as customized sponsored content ads. This are the ads that matches a application contents.
For Example:- A app has a list of items been displayed to the user you can insert a native ads similar look as of your list of items, showing native ads in between listview builder.
Advantage of native ads in app
- Customized ads content – ads look similar to your app UI.
- Eye Catching – App user will not feel irritated by ads.
- Native ads are higher in click-through-rate(CTR) so the earning may be more.
Then, Now let’s start integrating native ads in flutter project.
Video Tutorial on Flutter Native Ads
Integrate Native Admob Ads in Flutter Application
1. Create a new Flutter Project
Just open any existing flutter project or create a new project in your favorite IDE. In my case, i am making use of Android Studio IDE to build flutter projects.
2. Add dependencies
In your project, open pubspec.yaml file & add the native ads dependencies under dependencies section.
dependencies: flutter_native_admob: ^2.1.0+3 # this Line Version may change.
Note: This package is not yet updated with nullsafety feature so kindly check if your project environment sdk version is less then 2.12.0.
3. Platform prerequisite setup needed
- Android Setup
Open Android Studio module in Android Studio
Right click on android folder of your flutter project,
[right click] android > Flutter > Open android module in android studio.
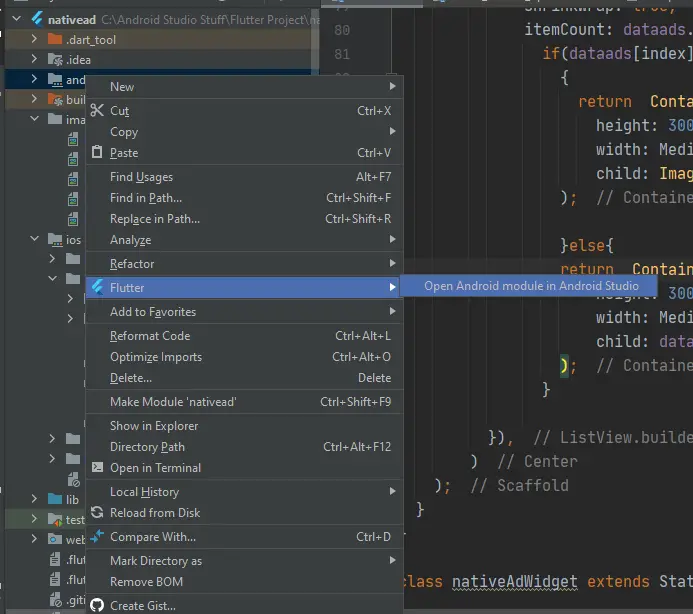
1. Adding google services classpath
open[project]/android/app/build.gradle file.
dependencies { classpath 'com.android.tools.build:gradle:4.1.0' classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version" classpath 'com.google.gms:google-services:4.3.0' // add this google services 4.3.0 }
2. Apply the google services plugin
at the bottom of same build.gradle file add the google services plugin.
apply plugin: 'com.google.gms.google-services'
3. Add meta-data of admob App ID in Android-Manifest.xml
<manifest ....... > <application android:label="nativead" android:icon="@mipmap/ic_launcher"> <!-- Sample AdMob App ID: ca-app-pub-3940256099942544~3347511713 --> <meta-data android:name="com.google.android.gms.ads.APPLICATION_ID" android:value="ca-app-pub-3940256099942544~3347511713"/> <!-- your admob adpp id </application> </manifest>
- iOS Setup
Add Admob App ID key & string value – iOS
Open[project]/ios/Runner/info.plist
you need to add a GADApplicationIdentifier<key> & exactly below key a string value of your admob app id.
and a flutter embedded_view_preview for native ad preview and set it to true.
<key>GADApplicationIdentifier</key> <string>ca-app-pub-3940256099942544~3347511713</string> <key>io.flutter.embedded_views_preview</key> <true/>
properties of flutter native admob package
property | description | |
adUnitID: | String | |
loading: | indicator to show ads loading progress | |
error: | if required, show a toast message to user about failed to load ads. | |
type: | type of ads NativeAdmobType.full. NativeAdmobType.banner. | |
conrolller: | To Control nativeadmobWidget | |
option: | Customize ads styling for appearance. |
In Options, NativeAdmobOptions there are various properties that you can use to customize your native ads appearance as per your flutter app UI. Check out pub.dev page for more.
How to use Native Admob Widget
1. Create a controller using NativeAdmobController class
final _controller = NativeAdmobController();
2. use NativeAdmob Widget to show the flutter admob ads
snippet code of NativeAdmob Widget wrapped with container
Container( height: 250, child: NativeAdmob( adUnitID: "ca-app-pub-3940256099942544/2247696110", //your ad unit id loading: Center(child: CircularProgressIndicator()), error: Text("Failed to load the ad"), controller: _controller, type: NativeAdmobType.full, options: NativeAdmobOptions( ratingColor: Colors.red, showMediaContent: true, callToActionStyle: NativeTextStyle( color: Colors.red, backgroundColor: Colors.black ), headlineTextStyle: NativeTextStyle( color: Colors.blue, ), // Others ... ), ), ),
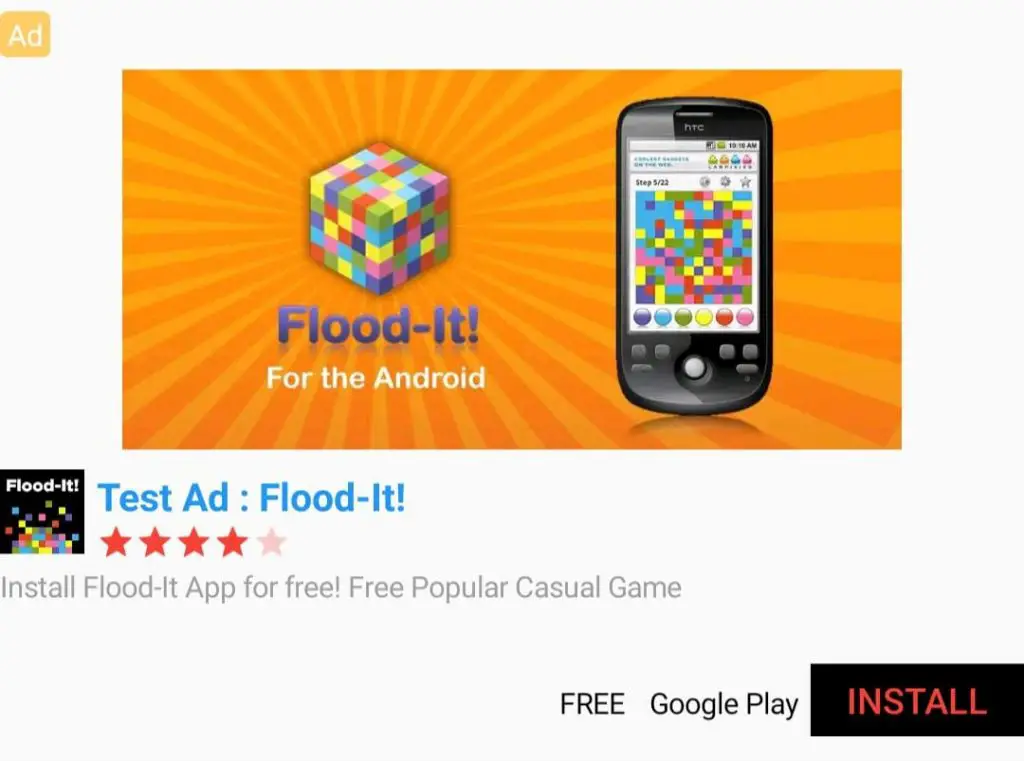
Load/show Admob Native Ads in listview.builder at random position – flutter
Complete Source Code.
main.dart
import 'dart:math'; import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:flutter_native_admob/flutter_native_admob.dart'; import 'package:flutter_native_admob/native_admob_controller.dart'; import 'package:flutter_native_admob/native_admob_options.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', debugShowCheckedModeBanner: false, theme: ThemeData( // This is the theme of your application. // // Try running your application with "flutter run". You'll see the // application has a blue toolbar. Then, without quitting the app, try // changing the primarySwatch below to Colors.green and then invoke // "hot reload" (press "r" in the console where you ran "flutter run", // or simply save your changes to "hot reload" in a Flutter IDE). // Notice that the counter didn't reset back to zero; the application // is not restarted. primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { List<String> images = ['images/img1.jpg','images/img2.jpg','images/img3.jpeg','images/img4.jpg','images/img5.jpg']; List<Object> dataads; @override void initState() { super.initState(); setState(() { dataads = List.from(images); for(int i = 0;i<2;i++){ var min = 1; var rm = new Random(); //generate a random number from 2 to 4 (+ 1) var rannumpos = min + rm.nextInt(4); //and add the banner ad to particular index of arraylist dataads.insert(rannumpos, nativeAdWidget()); } }); } @override Widget build(BuildContext context) { return Scaffold( body: Center( child: ListView.builder( scrollDirection: Axis.vertical, shrinkWrap: true, itemCount: dataads.length,itemBuilder: (context,index){ if(dataads[index] is String) { return Container( height: 300, width: MediaQuery.of(context).size.width, child: Image.asset(dataads[index]) ); }else{ return Container( height: 300, width: MediaQuery.of(context).size.width, child: dataads[index] as nativeAdWidget ); } }), ) ); } } class nativeAdWidget extends StatelessWidget { final _controller = NativeAdmobController(); @override Widget build(BuildContext context) { return NativeAdmob( adUnitID: "ca-app-pub-3940256099942544/2247696110", loading: Center(child: CircularProgressIndicator()), error: Text("Failed to load the ad"), controller: _controller, type: NativeAdmobType.full, options: NativeAdmobOptions( ratingColor: Colors.red, showMediaContent: true, callToActionStyle: NativeTextStyle( color: Colors.red, backgroundColor: Colors.black ), headlineTextStyle: NativeTextStyle( color: Colors.blue, ), // Others ... ), ); } }
output
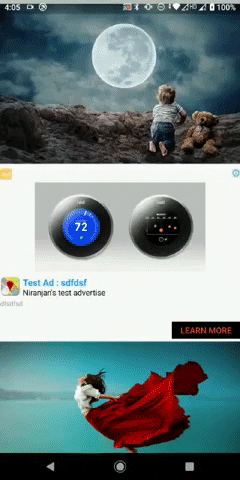