Hi Guys, Welcome to Proto Coders Point.
In this Flutter Tutorial article will learn How to call html page in flutter locally.
Basically load local web pages such as HTML, CSS, JavaScript from assets folder of flutter project.
So to acheive this we are going to make use of flutter package called ‘webview_flutter_plus‘.
Video Tutorial
About webview flutter plus
Flutter Webview plus is a updated version of webview_flutter.
By using Webview Flutter Plus package we can load local webpage into flutter app like ‘HTML‘, ‘CSS‘, ‘JAVASCRIPT‘ content right from Assets folder of your flutter project.
What’s news in this library
- It has all the feature present in webview flutter.
- Load HTML, CSS, JavaScript content from HTML Strings.
- Load HTML, CSS, JavaScript content from Assets folders.
I have already wrote an Article on flutter_webview, check it out or watch below video
Convert Website into Flutter App
Let’s get Started
Installation
Add this package in pubspec.yaml file
dependencies: webview_flutter_plus:
after adding hit ‘put get‘ button or run command flutter pub get, this will add above package library into your flutter project as external Libraries.
Import webview_flutter_plus to use it’s widget
Now once you have added above package, to use it you need to import ‘webview_flutter_plus.dart’ file.
import 'package:webview_flutter_plus/webview_flutter_plus.dart';
Add Internet permission & enable clearTextTraffic
Android
Make sure to add android:usesCleartextTraffic="true"
, In AndroidManifest.xml, under application tag.
<project-directory> / android / app / src / main / AndroidManifext.xml
<application android:label="webview_plus" android:icon="@mipmap/ic_launcher" android:usesCleartextTraffic="true"> ................. ................. ................. </application>
add Internet permission
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
iOS
Add TransportSecurity & embedded views preview.
<project-directory>/ios/Runner/Info.plist
<key>NSAppTransportSecurity</key> <dict> <key>NSAllowsArbitraryLoads</key> <true/> </dict> <key>io.flutter.embedded_views_preview</key> <true/>
Done will basic configuration to load Web pages
How to load Web page in flutter Webview
Snippets Code
1. Loading Website url in flutter app webview
WebViewPlus( initialUrl: 'https://protocoderspoint.com/', javascriptMode: JavascriptMode.unrestricted, ),
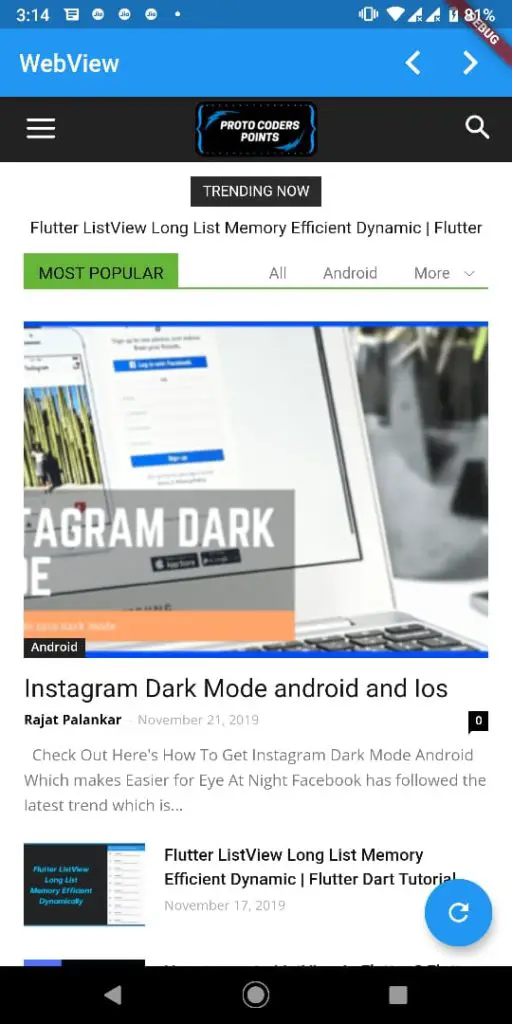
2. Load webpage from html string
Way 1 -> Direct load a html string in webview
WebViewPlus( javascriptMode: JavascriptMode.unrestricted, onWebViewCreated: (controller){ controller.loadString(r""" <html lang="en"> <body>hello world</body> </html> """); }, ),
Way 2 -> Create a String variable that hold complete HTML Code
String htmlpage = ''' <!DOCTYPE html>
<html>
<body>
<h2>Example to load html from string</h2>
<p>This is paragraph 1</p>
<img src="https://thumbs.dreamstime.com/b/sun-rays-mountain-landscape-5721010.jpg" width="250" height="250">
</body>
</html>''';
WebViewPlus( javascriptMode: JavascriptMode.unrestricted, onWebViewCreated: (controller){ controller.loadString(htmlpage); }, ),
Loading Webpage’s from Assets Folder in flutter app
Let’s create a webpages in Assets & Show it in flutter app using webview_flutter_plus.
Create Assets folder & WebPage Folder in your flutter project
<project_directory>/assets/website
Check my project structure for better understanding
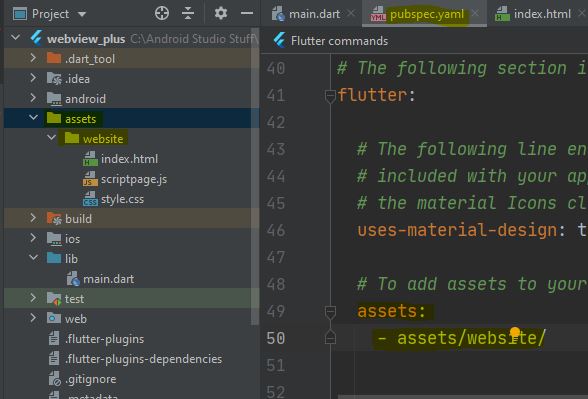
Add permission to access assets folder in pubspec.yaml as shown in above screenshot
flutter: # To add assets to your application, add an assets section, like this: assets: - assets/website/
The Web Page will have radio button when changed will change background color of HTML page.
To Acheive this we need 3 files, so let’s create it under assets/website/....
- HTML – > index.html
- CSS -> style.css
- Javascript -> script.js.
Respective code as below:-
index.html
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="style.css"> </head> <body id="colorback"> <h2>COLOR CHANGE</h2> <p>Select a Color To Change BackGround</p> <input type="radio" id="html" name="fav_language" onclick="submit('red')" value="RED" checked> <label for="html">RED</label><br> <input type="radio" id="css" name="fav_language" onclick="submit('blue')" value="BLUE"> <label for="css">BLUE</label><br> <button type="button" onclick="submit('green')" name="Submit">Submit</button> <script src="scriptpage.js"></script> </body> </html>
style.css
#colorback{ background: red; color:white; }
script.js
function submit(color){ var colorback = document.getElementById('colorback'); colorback.setAttribute('style','background:'+color); }
Flutter – main.dart
import 'package:flutter/material.dart'; import 'package:webview_flutter_plus/webview_flutter_plus.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('WebView Load HTML Locally From assets'), ), body: WebViewPlus( javascriptMode: JavascriptMode.unrestricted, onWebViewCreated: (controller){ controller.loadUrl('assets/website/index.html'); }, ), ); } }
Output – We have a Flutter App that load Webpage from assets folder
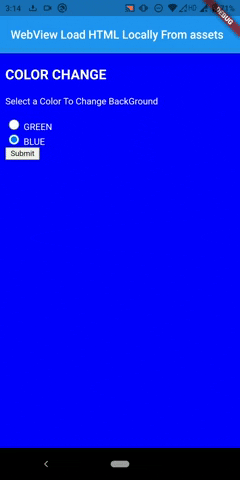