Hi Guy’s Welcome to Proto Coders Point, In this flutter article let’s check out how to use PageView widget in flutter.
Example on PageView Widget you will see in this article:-
- Simple 3 Pageview.
- PageView with dots indicator.
Before going into flutter code, let’s explore more about flutter pageview widget.
What is Flutter PageView? Where & How to use it
A page-by-page scrollable list is build by using flutter pageview widget. It been widely used to create a beautiful carousel or slideshow UI, Which you may have observed in many applications.
Pageview Constructor
PageView({ Key? key, Axis scrollDirection = Axis.horizontal, bool reverse = false, PageController? controller, ScrollPhysics? physics, bool pageSnapping = true, ValueChanged<int>? onPageChanged, List<Widget> children = const <Widget>[], DragStartBehavior dragStartBehavior = DragStartBehavior.start, bool allowImplicitScrolling = false, String? restorationId, Clip clipBehavior = Clip.hardEdge, ScrollBehavior? scrollBehavior, bool padEnds = true })
Dymanically page views building use below constructor, useful when you load content to be shown into PageView from server.
PageView.builder({ Key? key, Axis scrollDirection = Axis.horizontal, bool reverse = false, PageController? controller, ScrollPhysics? physics, bool pageSnapping = true, ValueChanged<int>? onPageChanged, required IndexedWidgetBuilder itemBuilder, ChildIndexGetter? findChildIndexCallback, int? itemCount, DragStartBehavior dragStartBehavior = DragStartBehavior.start, bool allowImplicitScrolling = false, String? restorationId, Clip clipBehavior = Clip.hardEdge, ScrollBehavior? scrollBehavior, bool padEnds = true })
Flutter PageView Example’s
Let’s implement it in below code
Example 1: Simple PageView Flutter Example
In this Example, I will create 3 separate dart file (page1, page2, page3) in lib directory of my flutter project. This 3 pages will be shown in main.dart page by using PageView Widget.
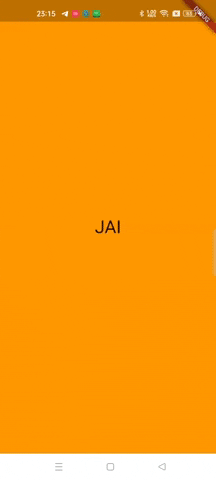
My Project Structure
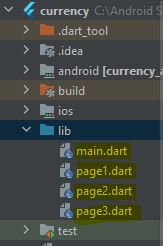
page1.dart
import 'package:flutter/material.dart'; class Page1 extends StatelessWidget { const Page1({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( backgroundColor: Colors.orange, body: Center( child: Text('Page1',style: TextStyle(fontSize: 30),), ), ); } }
Similarly create 2 more pages and design it as per your UI.
main.dart – Complete Code with Explanations
import 'package:flutter/material.dart'; import 'package:intl/intl.dart'; import 'page1.dart'; import 'page2.dart'; import 'page3.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { // Initializing a Controller fore PageView final PageController _pageViewController = PageController(initialPage: 0); // set the initial page you want to show @override void dispose() { // TODO: implement dispose super.dispose(); _pageViewController.dispose(); // dispose the PageController } @override Widget build(BuildContext context) { return Scaffold( //use pageview widget to show pages when user slides body: PageView( controller: _pageViewController, children: [ Page1(),Page2(),Page3() ], ) , ); } }
Example 2 – Pageview with indicator flutter example
Show a Page Slider active page dots, basically show a dots at bottom.
In Below Example, as I said will have dots indicator, which indicate which is the current active page, The App user can simply swipe left, right to switch between pages and also taps on the dot indicator to switch between pages.
Below code output:
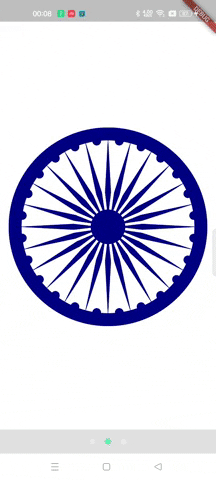
Complete Source Code – main.dart
import 'package:flutter/material.dart'; import 'page1.dart'; import 'page2.dart'; import 'page3.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { // Initializing a Controller fore PageView final PageController _pageViewController = PageController(initialPage: 0); // set the initial page you want to show int _activePage = 0; // will hold current active page index value //Create a List Holding all the Pages final List<Widget> _Pages = [ Page1(), Page2(), Page3() ]; @override void dispose() { // TODO: implement dispose super.dispose(); _pageViewController.dispose(); // dispose the PageController } @override Widget build(BuildContext context) { return Scaffold( // will make use of Stack Widget, so that One Widget can we placed on top body: Stack( children: [ PageView.builder( controller: _pageViewController, onPageChanged: (int index){ setState(() { _activePage = index; }); }, itemCount: _Pages.length, itemBuilder: (BuildContext context, int index){ return _Pages[index]; } ), //creating dots at bottom Positioned( bottom: 0, left: 0, right: 0, height: 40, child: Container( color: Colors.black12, child: Row( mainAxisAlignment: MainAxisAlignment.center, children: List<Widget>.generate( _Pages.length, (index) => Padding( padding: const EdgeInsets.symmetric(horizontal: 8), child: InkWell( onTap: () { _pageViewController.animateToPage(index, duration: const Duration(milliseconds: 300), curve: Curves.easeIn); }, child: CircleAvatar( radius: 5, // check if a dot is connected to the current page // if true, give it a different color backgroundColor: _activePage == index ? Colors.greenAccent : Colors.white30, ), ), )), ), ), ), ], ), ); } }