Picasso android library is – A powerful image loading, image downloading, and image caching library for Android.
This Picasso library allows for hassle-free image loading in your application—often in one line of code!
Picasso dependency is an open-source Android library that is developed and maintained by Square. This library hides all the complexity as it will deal with back-on threading, loading images from the internet, and even caching. Picasso makes memory-efficient to resize and transform images. Picasso Library makes it quite easier for displaying images from remote locations.
Final Output of this Picasso android tutorial.
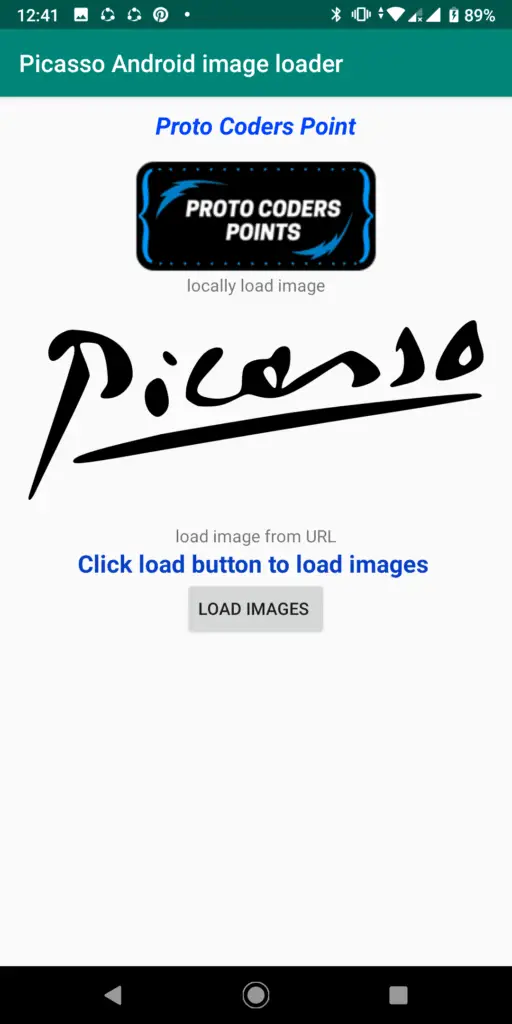
1. Feature of this Universal image loader library
- Handling
ImageView
recycling and download cancelation in an adapter. - Complex image transformations with minimal memory use.
- Automatic memory and disk caching.
2. Callbacks and Targets In Picasso In Android
Picasso library loads an image in two ways that are synchronously and Asynchronously.
.fetch() –this is an asynchronously load the image into a background thread. This method only saves the image into the disk or memory cache. It’s neither going to load the image into image view nor it is going to return any Bitmap. If you want to reduce the reduce image loading times and if you know that the image is needed by you shortly after then it could be used to fill the image cache in the background.
.get() – It returns a Bitmap object and loads an image synchronously. But it will freeze the UI if you will call it from the UI thread.
into(ImageView targetImageView) – It is provided by Picasso where you can Specify your target Imageview in which the image is supposed to get displayed.
In this tutorial, you will learn How to use Picasso android library to load images from the locally drawable drive and even how to load images from a URL.
How to add Picasso library in android studio Picasso dependency?
Navigate to your project file at the left side of your android studio. You will see “Gradle Scripts” open the drop down list, you will see a build.gradle (Module:app) open it.
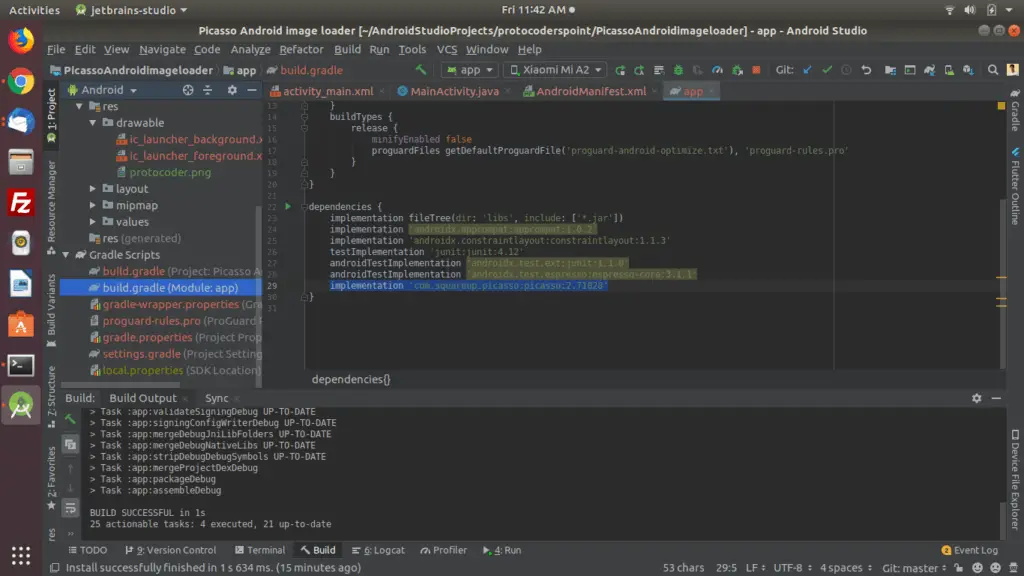
Before using Picasso we have to add its dependency in build.gradle file in module level : app.
Picasso dependency
implementation 'com.squareup.picasso:picasso:2.71828'
NOTE: To load image from URL android you need a internet permission that you need to assign in android manifest.xml file. As shown in below image.
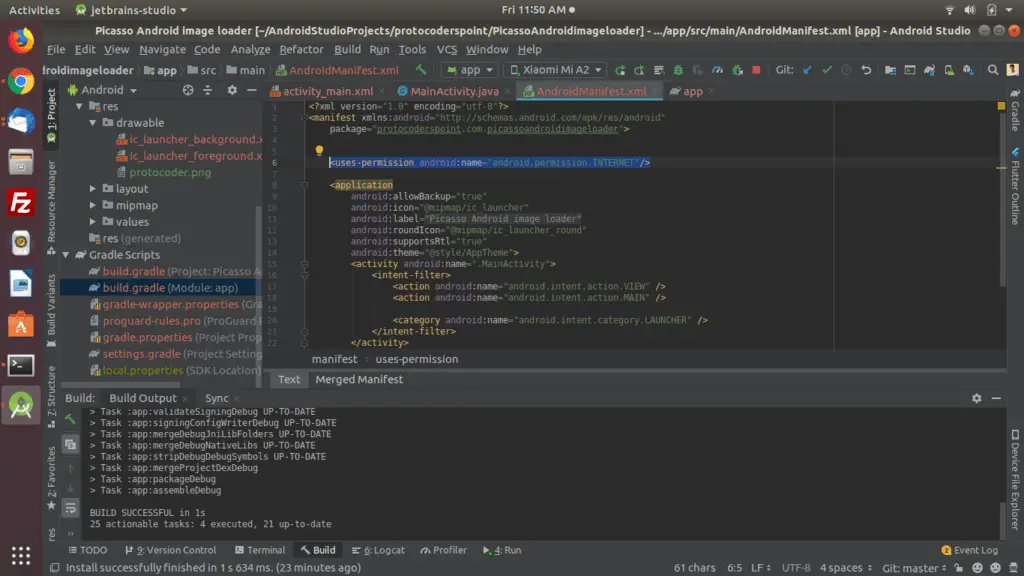
add internet access permission in AndroidMainfest.xml file.
<uses-permission android:name="android.permission.INTERNET"/>
Picasso to load image
To Simply load an image in and imageView
Picasso.get() .load("https://protocoderspoint.com/wp-content/uploads/2019/10/protocoderspoint-rectangle-round-.png") .into(imageView);
show placeholder Picasso android library
Picasso.get() . load("URL PATH") . placeholder(R.drawable.user_placeholder) . error(R.drawable.user_placeholder_error) . into(imageView);
Picasso supports both download and error placeholders as optional features.
We can specify an image as a place holder until the image is being loaded, placeholder are commenly used with we face any technical issue like network failed to load the picasso image, in such a case we make use of placeholder in picasso so that we can show a default image in place of real image.We can also give an image as an error handler if any error occurs while loading the image.
show error image using Picasso library
Picasso.get() .load("URL PATH") .error(R.drawable.user_placeholder_error) .into(imageView);
Image Transformation picasso android studio
Picasso.get() .load(url) .resize(50, 50) .centerCrop() .into(imageView)
As you know picasso is a universal image loader,So we can change image dimensions to fit layouts and reduce memory size utilized by image.
Image Rotation In Picasso
Picasso.with(context) .load("image source here") .rotate(90f) .into(someImageView);
This will rotate the image by 90 degrees.
Picasso Android Image loader library github.
Now let us begin by creating a project in android studio to implement Picasso universal image loader library.
Picasso Android Example
In the Example, i m loading an image from local and from URL when button is clicked OK.
Create a project with and of your wish package name i m using “protocoderspoint.com.picassoandroidimageloader” and add following code in respective files
activity_main.xml
For User interface design.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" android:orientation="vertical"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" android:layout_gravity="center" android:gravity="center" android:layout_margin="10dp"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Proto Coders Point" android:textColor="#0042FF" android:textSize="20sp" android:layout_marginBottom="15dp" android:textStyle="italic|bold"/> <ImageView android:id="@+id/imageView2" android:layout_width="wrap_content" android:layout_height="wrap_content" app:srcCompat="@mipmap/ic_launcher" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="locally load image"/> <ImageView android:id="@+id/imageView3" android:layout_width="wrap_content" android:layout_height="wrap_content" app:srcCompat="@mipmap/ic_launcher" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="load image from URL"> </TextView> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:textStyle="bold" android:textSize="20sp" android:textColor="#093FC4" android:text="Click load button to load images "> </TextView> <Button android:id="@+id/load_images" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Load images " /> </LinearLayout> </LinearLayout>
Main_Activity.java
For back end programming in java
package protocoderspoint.com.picassoandroidimageloader; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.ImageView; import com.squareup.picasso.Picasso; public class MainActivity extends AppCompatActivity { ImageView local_image,url_image; Button load_images; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); local_image=(ImageView)findViewById(R.id.imageView2); url_image=(ImageView)findViewById(R.id.imageView3); load_images=(Button)findViewById(R.id.load_images); load_images.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Picasso.get().load(R.drawable.protocoder).into(local_image); Picasso.get().load("https://cdn.pixabay.com/photo/2012/04/24/11/54/picasso-39592_960_720.png").into(url_image); } }); } }
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="protocoderspoint.com.picassoandroidimageloader"> <!--internet permission to load image from URL--> <uses-permission android:name="android.permission.INTERNET"/> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.VIEW" /> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
also read welcome-screen-github-library
[…] 2 : Adding picasso library into our project […]
[…] picasso library : used to show image fetched from firebase realtime database to imageView. […]