Hi Guys, Welcome to Proto Coders Point. In this Article. We will look into the Python Data Structure that help to understand python in deep.
Data structures are containers that organize and group data according to the Data Type
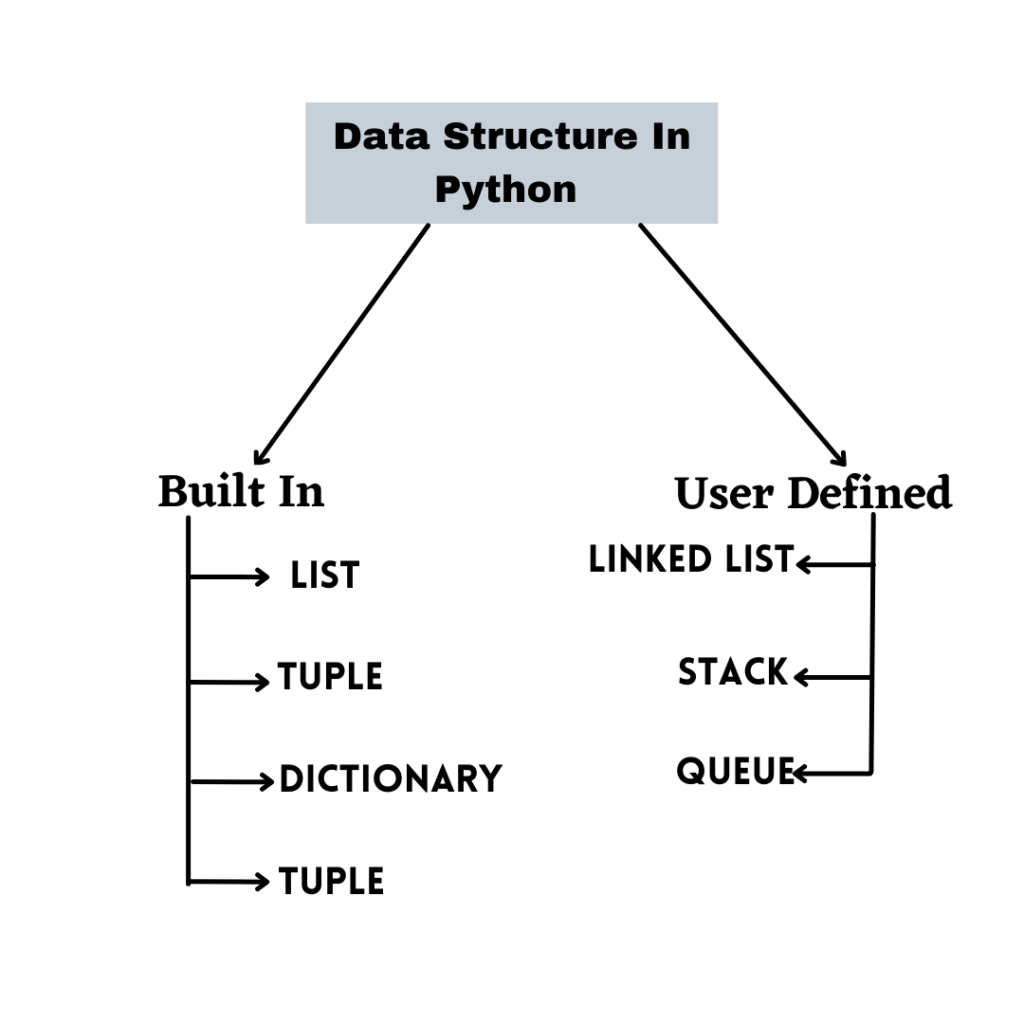
Types of data structure in python
There are 2 Types of Data Structure in Python:-
- Built-in Data Structure
- User Defined data Structure
Python built in data structures
The Python built-in data structures are
- list
- set
- tuples
- and dictionary.
1) List :-
List is sequence Datatypes in Python. Lists are used to store multiple Heterogeneous elements in a single variable. List is Mutable so we can modify and add the elements in the Present List.
List are represented in square Brackets []. It Support Slicing and Positive and Negative indexing.
Now we will see how we can perform operation on list.
Examples :-
#Integer Elements
list1 = [10,20,30]
list1
my_list =[]
#list With Heterogeneous Elements
mix_list = [10, ‘Hello’, 10.5, 20]
mix_list
Access a List Using Slicing and Indexing or Negative Indexing
li = [10,20,30,'a','b',20.5,'c',30.20]
print(li[2]) # Output – 30
print(li[4]) # Output – b
Negative Indexing
print(li[-1]) # Output – 30.20
print(li[-2]) # Output – 10.5
Nested Indexing
nest_list = [5,6,8,[9,7,2],3]
nest_list[2] # Output – 8
nest_list[3] # Output - [9, 7, 2]
nest_list[3][1] #Output - 7
2) Set :-
Set are a collection of elements that can be mutable, iterable. Set is used to store multiple elements in a single element.
Set are mutable.
Cannot accept duplicate Values.
Does not support for indexing
Some Built in Method for Sets
- add ()
- clear ()
- copy ()
- difference ()
- discard ()
- pop ()
- remove ()
- update ()
Examples:-
Set can create by using dictionary, list.
#Dictionary
set_ds = {5,10,15,15}
#Output -> {5, 10, 15}
set_ds = {10, (8, 1.5, 3), 'new'}
#Output -> {(8, 1.5, 3), 10, 'new'}
#List
set_list = [5,9,2,36,4,5]
type(set_list) #Output :- list
set(set_list) #Output :- {2,4,5,9,36} (Remove Duplicate Element and Print Element)
3) Dictionary
Dictionary is collection of items, python Dictionary are used to store the value in the form of key value pair, python Dict are created using curly braces {} separated by commas.
Dictionary Item are represented by key value pair.
Built-in Methods of py dict:-
- clear() –> Use to Clear elements from the dictionary.
- copy() –> Returns a new copy of the dictionary.
- items() –> Return a new object in key value format
- keys() –> Returns a dictionary keys
- values() –> Returns a dictionary’s values
#Examples :-
# dictionary with integer keys
my_dict = {1: 'water', 2: 'Bottle'}
my_dict = {'name': 'John', "age": 20}
print(my_dict[‘name’])
# Output:- John
# using dict()
my_dict = dict({1:'apple', 2:'ball'})
4) Tuple :-
Python Tuples is sequence Data Types that help to store multiple elements in one variable. It is Similar to the List but tuple has certain restriction like :-
Tuple are immutable. So We cannot change or modify elements from tuples.
Tuples are declared using () parenthesis
Accessing Tuples:-
There are certain ways that we can access Tuples .
- Indexing
Used index operator []
to access an element in a tuple,
The index starts from 0.
- Negative Indexing:-
a. Python allows negative indexing for its sequences.
b. The index of -1 refers to the last item, -2 to the second last item and so on.
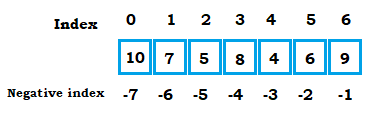
Examples:-
# Tuple having integers
new_tuple = (1, 2, 3)
print(new_tuple)
Output - (1, 2, 3)
# tuple with datatypes
new_tuple = (1, 2, 3, 'new', 10.20)
print(new_tuple)
Output - (1, 2, 3, 'new', 10.20)
# nested tuple
new_tuple = ("bat",(1, 2, 3), [4,5,6])
print(new_tuple)
Output - ("bat",(1, 2, 3), [4,5,6])
Accessing the Tuples:-
new_tuple = (1,6,8,9,10,6,87,9,2,3,6)
print(my_tuple[0]) # 1
print(my_tuple[5]) #6
print(my_tuple[-5]) #87
Python list to tuples example
For Converting list to tuple we have use inbuilt method i.e.tuple()
list_ex = [10,20,30]
print(list_ex)
#output :- [10, 20, 30]
tupl_ex = tuple(list_ex)
print(tupl_ex)
#Output :- (10, 20, 30)
Python User defined Data Structure
- Linked List
- Stack
- Queue
- Linked List
A linked list is a order of data Component, which are connected which each other using links. Each data component available with connection to another data component in form of a pointer.
2. Stack
A stack is a linear data structure that stores elements in LIFO or FILO manner.
In stack, a new elements is add at the end and element remove from end only.
For Adding elements we called PUSH & removing elements called POP.
LIFO :- Last In First Out
FILO :- First In Last Out
3. Queue
A Queue is a linear data structure that stores elements in FIFO(First In First Out) manner.
For Adding elements we called Enqueue & removing elements called Dequeue.
- Operations:-
- Enqueue :- Add element in Queue.
- Dequeue :- Remove the element in Queue.
- Front :- Get the First Element.
- Rear :- Get the Last Element.