Hi Guys, Welcome to Proto Coders Point, In this Flutter tutorial we will learn what is async & await in flutter.
In order to understand flutter async & await in dart, first we need to learn synchronous vs asynchronous programming in dart & how it works.
synchronous vs asynchronous programming
- synchronous: In simple words, When you execute code synchronously then you need to wait for it to finish task 1 before you move to task 2.
- asynchronous: When you execute code asynchronously, then you can continue to execute the next task, no need to wait for the previous task to complete, but in case if task 1 & task 2 are related like, talk 2 get data from task 1 then you need to make use of async & await in a flutter.
Understanding async & await in flutter
We write code or a lines of code that takes very short amount of time to execute & give result
print(''Hello World');
for example we want to print text on console, as you see that it is a single line statement that takes about fraction of second to complete the task, but there are some code or task that need more time to get executed completly for example: loading a image or File from internet.
Here are some common asynchronous task:-
- Fetching data(image or file) over a network.
- Writing data to a database.
- Reading a huge list of file from internet.
Therefore, to perform the asynchronous operations in a dart programming language, we make use of the Future class & the async & await keyword in a flutter.
synchronous and asynchronous programming code example
synchronous programming
for better understanding lets look into how synochronous code works,
//snippet method void Function(){ //step 1 print('Hello World'); //step 2 loadImage('url of image to load'); //step 3 print('Hello World'); }
When the above method is been called the step 1 gets executed immediately but step 3 executes only when the step 2 process is completely over/executed.
Suppose you have an image to be loaded in step 2 & due to an internet or network issue step 2 never get executed then you code will get stuck at step 2 and step 3 never gets executed until step 2 task is over.
Therefore, in synchronous method every thing works in a sync order/step by step process( The next task will not work until the current task is completed)
asynchronous programming
//snippet method void Function() async{ //step 1 print('Hello World'); //step 2 async_loadImage('URL of image to load'); //if loading image take time then step 3 will execute and simultaneously step 2 will load at same time there is not wait. //step 3 print('Welcome to proto coders point'); }
So, if you have a method that load image & is a async method then, while the image been getting loaded, the step 3 will get executed, it simply means that the step 3 will not wait until step 2 complete the task.
flutter synchronous example
synchronous.dart code example
void main(){ callMethods(); } void callMethods(){ method1(); method2(); method3(); } void method1(){ print('method 1 completed'); } void method2(){ print('method 2 completed'); } void method3(){ print('method 3 completed'); }
Here, its a synchronous code, which execute methods step by step process and the output will be in sequence order.
output
method 1 completed method 2 completed method 3 completed
Ok , then suppose if I add a sleep(duration) in method 2 then until sleep duration is not completed the execution of method 3 is still iin pending.
//snippet void method2(){ Duration wait3sec = Duration(seconds: 3); sleep(wait3sec); // sleep here for 3 second, then go forword print('method 2 completed'); }
Check out the screenshot of output
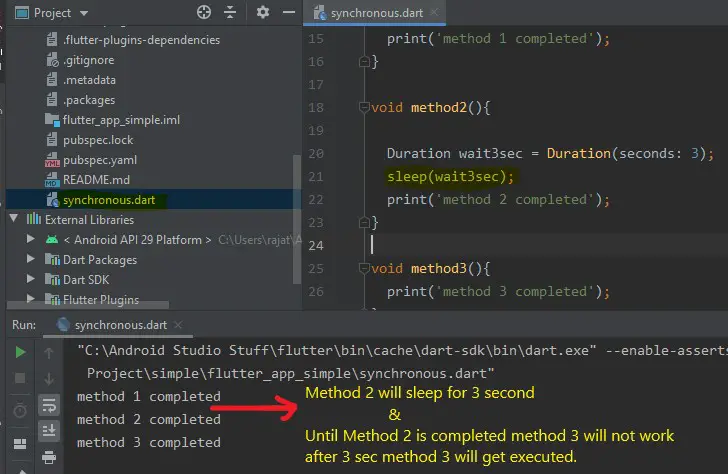
We have created artifically a sleep timer so that method 2 get paused for some time, but in real time application, the case might be different may be method 2 need time to load data or there might be network issue due to which data is loading slowly like downloading Imahe or files through Internet,
Therefore, if we use the synchronous method then until the image or file gets downloaded, method 3 will not execute, so this is the disadvantage of sync methods.
Flutter delayed method in flutter
Suppose you want to execute a piece of code after some duration then you can make use of async method i.e. Future.delayed(duration,(){});
void method2(){ Duration wait3sec = Duration(seconds: 5); Future.delayed(wait3sec,(){ print('Future delayed executes after 5 seconds '); }); print('method 2 completed'); }
Now, if i add future delayed to above method 2() then the output will be something like this:
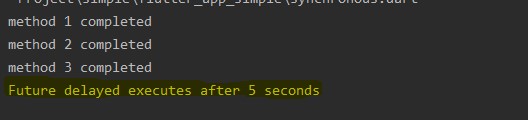
In above example, method 1,2,3 get executed immediatly there is not wait time, but in method 2 i have a future delayed that executed after some duration.
Why & When to use async & await in flutter
Below Example shows you what happens if async & await is not used.
import 'dart:io'; void main(){ callMethods(); } //note: that method 3 get data from the result of method 2, but method 3 get executed before method 2 return the result, due to which null get printed void callMethods(){ method1(); String result = method2(); method3(result); } void method1(){ print('method 1 completed'); } String method2(){ Duration wait3sec = Duration(seconds: 2); String result; Future.delayed(wait3sec,(){ result = 'data from method 2 '; }); return result; } void method3(String data_from_method2){ print('method 3 completed, received ${data_from_method2}'); }
output
method 1 completed method 3 completed, received null
//note: method 3 get data from the result of method 2, but method 3 get executed before method 2 return the result, due to which null get printed.
So, as in above dart code, The method 3 display result as null because method 3 is called immediatly before method 2 return the data therefore string result is null & is passed to method 3 as null.
When to use async & await in flutter dart
Then as you see in the above example method 3 depended of the data that method 2 return, so to prevent null passed to method 3, we make use of async & await in dart.
Snippet Example how async await works in dart
import 'dart:io'; void main(){ callMethods(); } void callMethods() async{ method1(); String result = await method2(); method3(result); } void method1(){ print('method 1 completed'); } Future method2() async{ Duration wait3sec = Duration(seconds: 2); String result; await Future.delayed(wait3sec,(){ result = 'data from method 2 '; }); return result; } void method3(String data_from_method2){ print('method 3 completed, received ${data_from_method2}'); }
Explanation of above code.
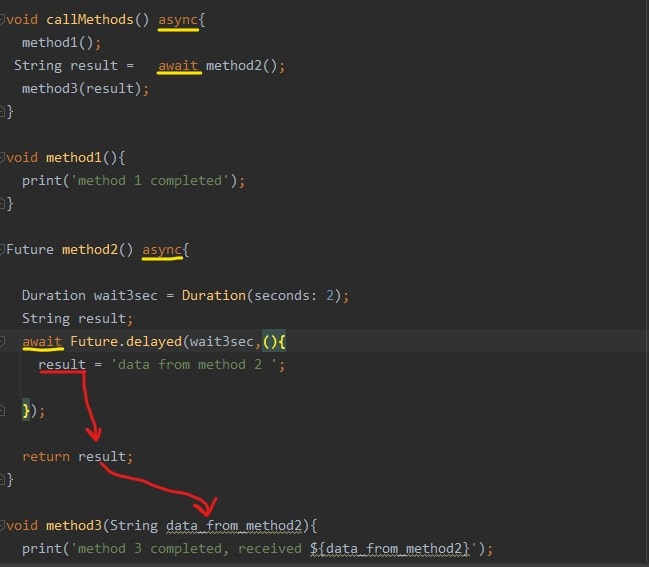
So as you see the method3() needs data that method2 returns, so we are making use of async & await so that method 2 waits until it returns a result, and then once the result is available it is been passed to method 3. therefore method 3 runs successfully with null safety.
Output of above code
method 1 completed method 3 completed, received data from method 2