Hi Guys, Welcome to Proto Coders Point In this Android Tutorial we will Learn about Android AsyncTask and Learn How to Fetch JSON data using AsyncTask and Handle them.
Final Project Result
What is AsyncTask ?
In android, AsyncTask is been used to run any process that should be run in background and likewise update the UI(User Interface).
Mainly AsyncTask is used to fetch data from a server and this will not effect our main Thread that is handling UI.
AsyncTask ( Asynchronous Task) Help you n running the background Process which will create a seperate Thread to run in background and then Synchronize again with the main Thread, This process will not disturb the main Thread and UI process will run smoothly.
This class will override at least one method i.e doInBackground(Params) and most often will override second method onPostExecute(Result).
To Execute AsyncTask you need to called Execute() method.
The Flow of AsyncTask Process
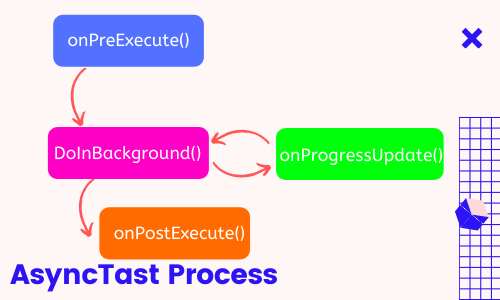
When AsyncTask class is executed onPreExecute() is called then doInBackground() is called to execute background processed and at last onPostExecute() method is called to update the data to UI.
Syntax of AsyncTask:
private class DownloadFilesTask extends AsyncTask<URL, Integer, Long> { @Override protected void onPreExecute() { super.onPreExecute(); // mostly used to show progress dialog // display a progress dialog for good user experiance } @Override protected Long doInBackground(URL... urls) { // code that will run in the background return ; } @Override protected void onProgressUpdate(Integer... progress) { // receive progress updates from doInBackground } @Oveerride protected void onPostExecute(Long result) { // update the UI after background processes completes } }
Here is How to execute AsyncTask class from main Thread
new DownloadFilesTask().execute();
Android AsyncTask Example Handling JSON and parse JSON
In the Below Example of AsyncTask We are performing a network operation, where we gonna fetch data from JSON File and Display them in our UI.
My JSON DATA URL : https://protocoderspoint.com/jsondata/superheros.json
Step 1 : As usual Create a new Android project in android studio.
File > New > New Project
Give relevent name to your project
Step 2 : Adding picasso library into our project Dependencies
Gradle Script > build.gradle(app level)
dependencies { ........ ....... implementation 'com.squareup.picasso:picasso:2.71828' }
Step 3 : UI Design Open res -> layout ->activity_main.xml add following code
Here we have 5 Views,
- Button that Perform Click event.
- Spinner used to select lists.
- 2 TextViews to Display data fetched from server.
- 1 ImageView to Display Image from server.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:padding="30dp" tools:context=".MainActivity"> <Button android:id="@+id/displayData" android:layout_width="200dp" android:layout_height="wrap_content" android:layout_gravity="center" android:layout_marginTop="20dp" android:background="@color/colorPrimary" android:text="Display Hero Data" android:textColor="#fff" android:textSize="20sp" /> <Spinner android:id="@+id/selectHero" android:layout_marginTop="20dp" android:layout_gravity="center" android:layout_width="match_parent" android:layout_height="wrap_content" /> <TextView android:id="@+id/titleTextView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="50dp" android:text="Hero Name: " android:visibility="gone" android:textColor="#000" android:textSize="18sp" /> <TextView android:id="@+id/categoryTextView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="5dp" android:text="His Ability: " android:visibility="gone" android:textColor="#000" android:textSize="18sp" /> <ImageView android:id="@+id/imageView" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_gravity="center" android:visibility="gone" android:fitsSystemWindows="true" android:layout_marginTop="20dp" android:scaleType="centerCrop" /> </LinearLayout>
Step 4: Java Code Open src -> package -> MainActivity.java
In MainActivity.java code.
We first refer the views present in XML File like Buttons, TextView, Spinner and ImageView.
Their after we perform onClick event that execute AsyncTask Class “MyAsyncTasks”.
Then In doInBackground() we fetch all the data from the server link mentioned above.
Then, In onPostExecute method we simply parse the JSON data fetched and Display then in the UI.
and We have used Picasso Library just to show the Fetch URL image in the ImageView.
package com.ui.asynctaskexample; import android.app.ProgressDialog; import android.content.Context; import android.os.AsyncTask; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.AdapterView; import android.widget.ArrayAdapter; import android.widget.Button; import android.widget.ImageView; import android.widget.Spinner; import android.widget.TextView; import android.widget.Toast; import androidx.appcompat.app.AppCompatActivity; import com.squareup.picasso.Picasso; import org.json.JSONArray; import org.json.JSONException; import org.json.JSONObject; import java.io.InputStream; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class MainActivity extends AppCompatActivity { String apiUrl = "https://protocoderspoint.com/jsondata/superheros.json"; String title, image, category; TextView titleTextView, categoryTextView; ProgressDialog progressDialog; Button displayData; ImageView imageView; int index_no; Spinner spinner; String[] HerosNameList = { "Flash", "SuperMan", "Thor", "Hulk", "Venom"}; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // get the reference of View's titleTextView = (TextView) findViewById(R.id.titleTextView); categoryTextView = (TextView) findViewById(R.id.categoryTextView); displayData = (Button) findViewById(R.id.displayData); imageView = (ImageView) findViewById(R.id.imageView); spinner=(Spinner)findViewById(R.id.selectHero); //Creating the ArrayAdapter instance having the country list ArrayAdapter aa = new ArrayAdapter(this,android.R.layout.simple_spinner_item,HerosNameList); aa.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item); //Setting the ArrayAdapter data on the Spinner spinner.setAdapter(aa); spinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() { @Override public void onItemSelected(AdapterView<?> parent, View view, int position, long id) { index_no=position; } @Override public void onNothingSelected(AdapterView<?> parent) { } }); // implement setOnClickListener event on displayData button displayData.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { // create object of MyAsyncTasks class and execute it MyAsyncTasks myAsyncTasks = new MyAsyncTasks(); myAsyncTasks.execute(); } }); } public class MyAsyncTasks extends AsyncTask<String, String, String> { @Override protected void onPreExecute() { super.onPreExecute(); // display a progress dialog for good user experiance progressDialog = new ProgressDialog(MainActivity.this); progressDialog.setMessage("Please Wait"); progressDialog.setCancelable(false); progressDialog.show(); } @Override protected String doInBackground(String... params) { // implement API in background and store the response in current variable String current = ""; try { URL url; HttpURLConnection urlConnection = null; try { url = new URL(apiUrl); urlConnection = (HttpURLConnection) url.openConnection(); InputStream in = urlConnection.getInputStream(); InputStreamReader isw = new InputStreamReader(in); int data = isw.read(); while (data != -1) { current += (char) data; data = isw.read(); System.out.print(current); } Log.d("datalength",""+current.length()); // return the data to onPostExecute method return current; } catch (Exception e) { e.printStackTrace(); } finally { if (urlConnection != null) { urlConnection.disconnect(); } } } catch (Exception e) { e.printStackTrace(); return "Exception: " + e.getMessage(); } return current; } @Override protected void onPostExecute(String s) { Log.d("data", s.toString()); // dismiss the progress dialog after receiving data from API progressDialog.dismiss(); try { JSONObject jsonObject = new JSONObject(s); JSONArray jsonArray1 = jsonObject.getJSONArray("superheros"); JSONObject jsonObject1 =jsonArray1.getJSONObject(index_no); title = jsonObject1.getString("name"); category = jsonObject1.getString("power"); image=jsonObject1.getString("url"); //make all the view Visible when data is ready to show titleTextView.setVisibility(View.VISIBLE); categoryTextView.setVisibility(View.VISIBLE); imageView.setVisibility(View.VISIBLE); titleTextView.setText("Hero Name : "+title); categoryTextView.setText("His Ability : "+category); Picasso.get().load(image).into(imageView); } catch (JSONException e) { e.printStackTrace(); } } } }
Step 5 : Adding a INTERNET PERMISSION in manifest.xml file
<uses-permission android:name="android.permission.INTERNET"/>
And their you go the app is ready to get Tested.
More on: How to Parse JSON data in flutter app development
Flutter Profile Page UI : Flutter Profile Page UI Design