Hi Guys, Welcome to Proto Coders Point , In This Tutorial we gonna implement A Sliver App Bar in Flutter App.
What is Sliver AppBar Class?
A Sliver AppBar in Flutter is similar to any normal AppBar, The Different here is only that Sliver AppBar come with ScrollView effect.
The Sliver App bar should be the first child of a CustomScrollView, Using with we can integrate the appbar with a scroll view so that it can vary in height and other content of the scrollview.
Implementation with Sliver AppBar Widget
Below Examples will show you how appbars can we integrated with different configurations and act with different behaviour when any user Scrolls the View up or down.
Example 1:
When App bar with
- floating: false,
- pinned: false,
- snap: false
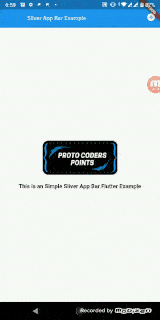
StateFull Class Code
class _NewPageState extends State<NewPage> { @override Widget build(BuildContext context) { return Scaffold( body: CustomScrollView( slivers: <Widget>[ SliverAppBar( expandedHeight: 150.0, floating: false, pinned: false, snap: false, actions: <Widget>[ IconButton( icon: const Icon(Icons.add_circle), onPressed: () {}, ), ], flexibleSpace: FlexibleSpaceBar( title: Text( "Sliver App Bar Example", style: TextStyle(fontSize: 15), ), background: Image.asset( 'images/images.jpeg', fit: BoxFit.cover, ), ), ), SliverFillRemaining( child: new Center( child: new Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Image.asset('images/protocoderspointlogo.png'), SizedBox( height: 20.0, ), Text( "This is an Simple Sliver App Bar Flutter Example", style: TextStyle(fontSize: 15.0, fontWeight: FontWeight.w800), ), ], ), ), ) ], ), ); } }
Example 2 :
When Sliver App bar with
- floating: true,
- pinned: true,
- snap: false
The Code base for this to work is very simply in the above lined of code you just need to change floating to true and pinned to true, this Configuration will simple pin the app bar at the top of the screen when used scroll through the app and other remaining widget will simple scroll to the end.
StateFull Class Code
class _NewPageState extends State<NewPage> { @override Widget build(BuildContext context) { return Scaffold( body: CustomScrollView( slivers: <Widget>[ SliverAppBar( expandedHeight: 150.0, floating: true, //change is here pinned: true, //change si snap: true, actions: <Widget>[ IconButton( icon: const Icon(Icons.add_circle), onPressed: () {}, ), ], flexibleSpace: FlexibleSpaceBar( title: Text( "Sliver App Bar Example", style: TextStyle(fontSize: 15), ), background: Image.asset( 'images/images.jpeg', fit: BoxFit.cover, ), ), ), SliverFillRemaining( child: new Center( child: new Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Image.asset('images/protocoderspointlogo.png'), SizedBox( height: 20.0, ), Text( "This is an Simple Sliver App Bar Flutter Example", style: TextStyle(fontSize: 15.0, fontWeight: FontWeight.w800), ), ], ), ), ) ], ), ); } }
Explaination of above code
1. As i said before that Sliver App bar must be the child of CustomScrollView to get Scrolling effect, The CustomScrollView will make the SliverAppBar into Scrolling.
2. Here i have Used an IconButton just to Show that you can even add IconButton Widget into the Appbar. i’e IconButton, which is used with actions to show buttons on the app bar.
Snippet
actions: <Widget>[ IconButton( icon: const Icon(Icons.add_circle), onPressed: () {}, ), ],
Flexible SpaceBar is used so that we can easily set and maximum expandable size to it when user scroll the app Down-words,
And Even we can set many other properties like background,title etc
SliverFillRemaining that fills the remaining space in the viewport. In Other words The Remaining body should not be null, is the Remaininig body is null then the app bar scrollview will not work as accepted, this value cannot be null.
So i have just Defined some widget in the remaining part in Sliver Fill Remaining
which has a child which is set to center , in turn has a child Column, then This Column have 3 children widget Image,SixedBox,and a Text.
Snippet Code
SliverFillRemaining( child: new Center( child: new Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Image.asset('images/protocoderspointlogo.png'), SizedBox( height: 20.0, ), Text( "This is an Simple Sliver App Bar Flutter Example", style: TextStyle(fontSize: 15.0, fontWeight: FontWeight.w800), ), ], ), ), )
Complete Source code of Flutter Sliver App Bar – An Collapsing ScrollView App Bar
main.dart
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( // This is the theme of your application. // // Try running your application with "flutter run". You'll see the // application has a blue toolbar. Then, without quitting the app, try // changing the primarySwatch below to Colors.green and then invoke // "hot reload" (press "r" in the console where you ran "flutter run", // or simply save your changes to "hot reload" in a Flutter IDE). // Notice that the counter didn't reset back to zero; the application // is not restarted. primarySwatch: Colors.blue, ), home: NewPage(), ); } } class NewPage extends StatefulWidget { @override _NewPageState createState() => _NewPageState(); } class _NewPageState extends State<NewPage> { @override Widget build(BuildContext context) { return Scaffold( body: CustomScrollView( slivers: <Widget>[ SliverAppBar( expandedHeight: 150.0, floating: true, pinned: true, snap: true, actions: <Widget>[ IconButton( icon: const Icon(Icons.add_circle), onPressed: () {}, ), ], flexibleSpace: FlexibleSpaceBar( title: Text( "Sliver App Bar Example", style: TextStyle(fontSize: 15), ), background: Image.asset( 'images/images.jpeg', fit: BoxFit.cover, ), ), ), SliverFillRemaining( child: new Center( child: new Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Image.asset('images/protocoderspointlogo.png'), SizedBox( height: 20.0, ), Text( "This is an Simple Sliver App Bar Flutter Example", style: TextStyle(fontSize: 15.0, fontWeight: FontWeight.w800), ), ], ), ), ) ], ), ); } }