Hi Guy’s Welcome to Proto Coders Point. In this flutter dart article let’s have a look into different way to concatenate 2 list in dart(how to join 2 list in flutter).
1. Using dart list class addAll() method
In Flutter dart, list class has a method addAll() using which we can easily combine 2 lists or join it and concat it as a single list.
Example:
void main() { List listOne = [9,8,7]; List listTwo = [6,5,4]; listOne.addAll(listTwo); print('Output: ${listOne}'); }
Here listOne.addAll(listTwo), all the data in listTwo will get added in listOne.
Output:
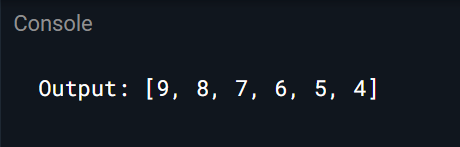
2. Combine 2 list using (+) addition operation in dart
You can simply make use of Addition operator (+) to concat/add 2 list in one.
Example:
void main() { List num1 = [1,4,3]; List num2 = [2,6,9]; final List numList = num1 + num2; print('Output: ${numList}'); }
Output:
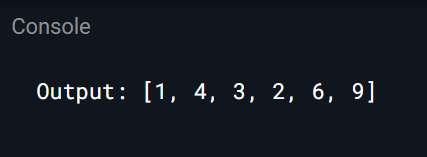
3. Concat 2 list using spread operator in flutter dart
By using spread operator you can merge 2 array list in one, “…” 3 dots indicate spread operator in flutter dart as so called as Cascade Operator. by using “…” you can combine/join 2 list data into one.
Example:
void main() { List pets = ['dog','cat','horse','fish']; List birds = ['crow','pegion','peacock']; final List animalList = [...pets,...birds]; print('Output: ${animalList}'); }
Output:

Recommended dart articles
dart convert list to set or vice versa
dart program to calculate product
Basic of dart program – Learn dart