Hi Guys, Welcome to Proto Coders Point, In this Flutter Tutorial we will implement Widget of the week i.e ListWheelScrollView used to build a listview with 3d scrollView effect in flutter.
Brief about Flutter ListWheelScrollView
ListView are great but sometimes ordinary Flutter ListView are boring, Therefore Flutter Team have built a new widget of the week that is List Wheel ScrollView.
This Widget is similar to a ListView but the difference here is that the list will be somewhat like a 3d ListView. It’s a box in which a children is on a wheel can be scrolled.
Here i main thing to understand is When the list at the zero scroll offset,the first child is aligned with the middle on the screen, and when the list is at the final scroll offset, the list child is aligned with the middle viewport.
Here the children are been rendereed as a circular rotation rather then simple scrolling on a plane.
Visit official site to learn in depth Here
So Let’s set Implementing this Flutter Listwheelscrollview widget class in our project.
Widget Class Code for above widget
ListWheelScrollView( controller: _controller, itemExtent: 80, diameterRatio: 2.5, physics: FixedExtentScrollPhysics(), children: <Widget>[], //list of widget Here
ListWheelScrollView have many optional properties, where 2 property of them are mandatory @required children <Widget> [ ], and itemExtent : 80
children <Widget> [ ] : accepts list of widgets
itemExtent : Specifies the pixel Height of each Children.
diameterRatio : you can tune the diameter of the list wheel widget.
offAxixFraction : -1.5 : Here is an Example on how the 3d listview looks when offassexFraction is set to -1.5.
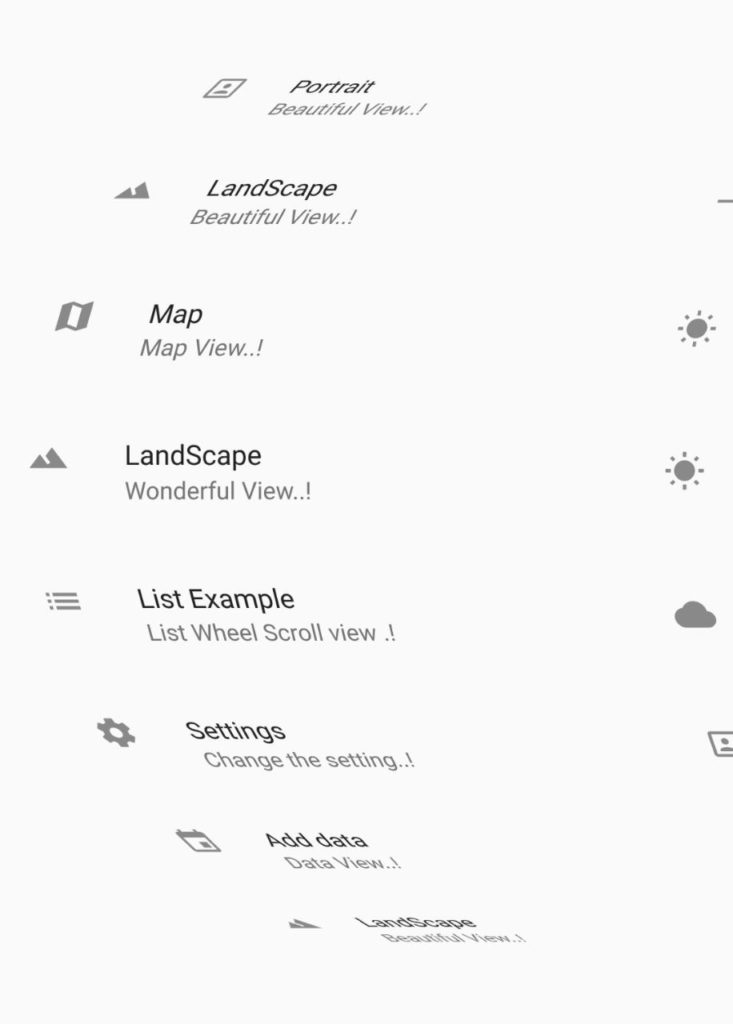
You can add a magnification effect to the flutter List Wheel ScrollView
magnification : used to set the zoomed size.
useMagnifier : true or false.
Here is an example for that
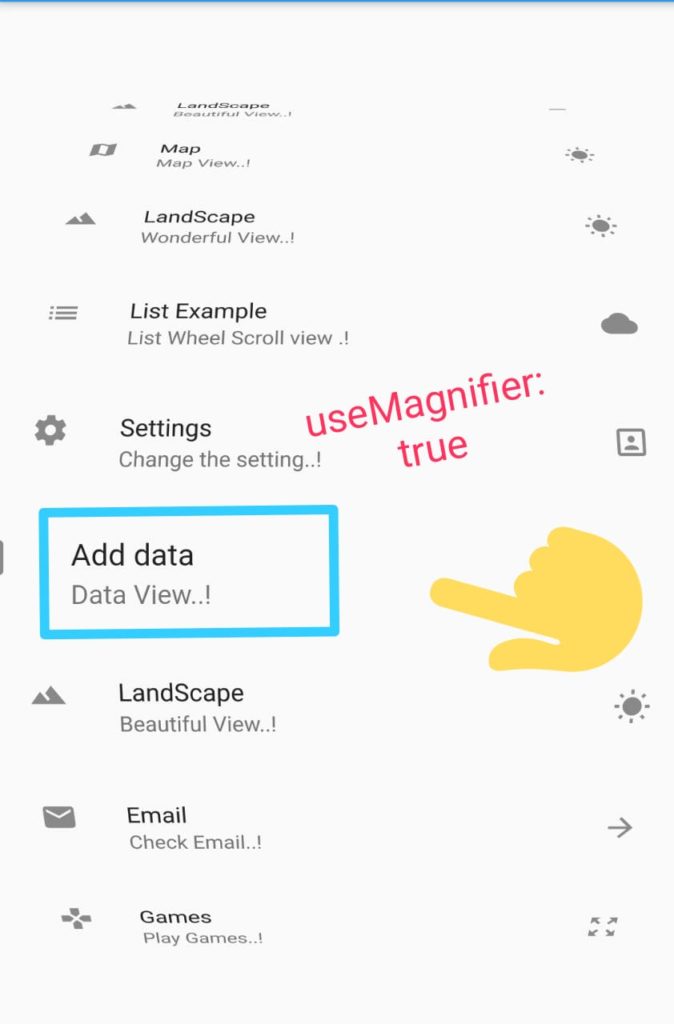
Creating a list of Widget
List<Widget> listtiles = [ ListTile( leading: Icon(Icons.portrait), title: Text("Portrait"), subtitle: Text("Beautiful View..!"), trailing: Icon(Icons.arrow_forward_ios), ), ListTile( leading: Icon(Icons.landscape), title: Text("LandScape"), subtitle: Text("Beautiful View..!"), trailing: Icon(Icons.remove), ), ListTile( leading: Icon(Icons.map), title: Text("Map"), subtitle: Text("Map View..!"), trailing: Icon(Icons.wb_sunny), ), ListTile( leading: Icon(Icons.landscape), title: Text("LandScape"), subtitle: Text("Wonderful View..!"), trailing: Icon(Icons.wb_sunny), ), ListTile( leading: Icon(Icons.list), title: Text("List Example"), subtitle: Text("List Wheel Scroll view .!"), trailing: Icon(Icons.cloud), ), ListTile( leading: Icon(Icons.settings), title: Text("Settings"), subtitle: Text("Change the setting..!"), trailing: Icon(Icons.portrait), ), ListTile( leading: Icon(Icons.event), title: Text("Add data"), subtitle: Text("Data View..!"), trailing: Icon(Icons.add), ), ListTile( leading: Icon(Icons.landscape), title: Text("LandScape"), subtitle: Text("Beautiful View..!"), trailing: Icon(Icons.wb_sunny), ), ListTile( leading: Icon(Icons.email), title: Text("Email"), subtitle: Text("Check Email..!"), trailing: Icon(Icons.arrow_forward), ), ListTile( leading: Icon(Icons.games), title: Text("Games"), subtitle: Text("Play Games..!"), trailing: Icon(Icons.zoom_out_map), ), ];
In the above snippet code i have simply created a list of ListTile widgets .
The ListTile widgets simple have some of it’s property like leading widget, title & subtitle of the listTile and a trailing widget as an Icon widget.
Then i m just using this List of Widgets in ListWheelScrollView Widgets to show the Items as it’s children.
ListWheelScrollView( controller: _controller, itemExtent: 80, magnification: 1.2, useMagnifier: true, physics: FixedExtentScrollPhysics(), children: listtiles, // Here listtiles is the List of Widgets. ),
Flutter List Wheel ScrollView – A 3D ListView in a flutter with Example
Complete Source code
Once you know the Basic of the Widget, Just Copy paste the Below lines of code in main.dart file.
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { final FixedExtentScrollController _controller = FixedExtentScrollController(); List<Widget> listtiles = [ ListTile( leading: Icon(Icons.portrait), title: Text("Portrait"), subtitle: Text("Beautiful View..!"), trailing: Icon(Icons.arrow_forward_ios), ), ListTile( leading: Icon(Icons.landscape), title: Text("LandScape"), subtitle: Text("Beautiful View..!"), trailing: Icon(Icons.remove), ), ListTile( leading: Icon(Icons.map), title: Text("Map"), subtitle: Text("Map View..!"), trailing: Icon(Icons.wb_sunny), ), ListTile( leading: Icon(Icons.landscape), title: Text("LandScape"), subtitle: Text("Wonderful View..!"), trailing: Icon(Icons.wb_sunny), ), ListTile( leading: Icon(Icons.list), title: Text("List Example"), subtitle: Text("List Wheel Scroll view .!"), trailing: Icon(Icons.cloud), ), ListTile( leading: Icon(Icons.settings), title: Text("Settings"), subtitle: Text("Change the setting..!"), trailing: Icon(Icons.portrait), ), ListTile( leading: Icon(Icons.event), title: Text("Add data"), subtitle: Text("Data View..!"), trailing: Icon(Icons.add), ), ListTile( leading: Icon(Icons.landscape), title: Text("LandScape"), subtitle: Text("Beautiful View..!"), trailing: Icon(Icons.wb_sunny), ), ListTile( leading: Icon(Icons.email), title: Text("Email"), subtitle: Text("Check Email..!"), trailing: Icon(Icons.arrow_forward), ), ListTile( leading: Icon(Icons.games), title: Text("Games"), subtitle: Text("Play Games..!"), trailing: Icon(Icons.zoom_out_map), ), ]; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("ListView ScrollView Wheel"), ), body: Center( child: ListWheelScrollView( controller: _controller, itemExtent: 80, magnification: 1.2, useMagnifier: true, physics: FixedExtentScrollPhysics(), children: listtiles, //List of widgets ), )); } }