Hi Guys, Welcome to Proto Coders Point.
In this Flutter tutorial(Article) we will learn how to check internet connection continuously in flutter by using flutter connectivity package & implement network change state management using Flutter GetX package.
About flutter connectivity package
This plugin is very useful if your app need internet connection to run the application perfectly, This Library allows your flutter Application to Discover Network Connectivity. This Flutter Library will also check if your mobile is currently using cellular mobile data or is using WiFi Connection.
This Flutter Plugin Perfectly works for Both Android and iOS devices, So it is been rated with 100 points in Flutter Library Store.
Video Tutorial
Flutter check internet connection using Connectivity and GetX State management package
Project Files Structure of references
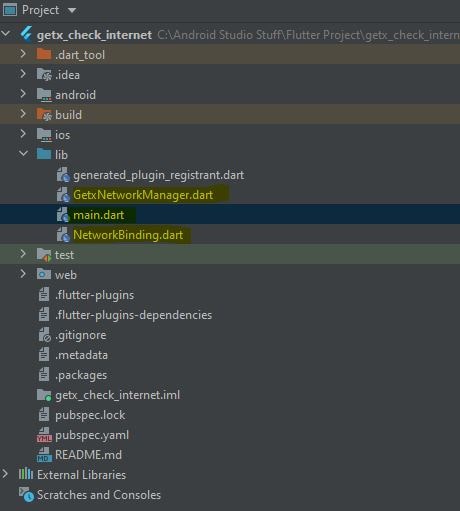
Note: In File Structure, ‘generated_plugin_registrant.dart’ get automatically created when you add below dependencies. You no need to create it.
1. Add Dependencies
dependencies: get: connectivity:
As we know, we need 2 packages i.e. Flutter GetX and Flutter connectivity.
Flutter GetX: To implement State Management, suppose if Internet Connection changed, to update the state of app and tell about the network change by updating UI screen of users.
Flutter Connectivity: This package plugin helps to check connectivity, if you are connected to WIFI or Mobile Data or if flutter no internet connection. Note: Connectivity plugin has stopped further updated, so Kindly use Connectivity_plus. Don’t worry code is same as it is in Connectivity plugin.
2. GetX State management- flutter check internet connection
Now, Create a dart file in lib directory of your flutter project.
I have created the file and named it as ‘GetXNetworkManager.dart’
GetXNetworkManager.dart
This Class manager all the network related task such as checkConnectivity, ConnectivityResult weather your mobile is connected to WIFI, Mobile Data, or no Internet connection.
import 'dart:async'; import 'package:connectivity/connectivity.dart'; import 'package:flutter/services.dart'; import 'package:get/get.dart'; class GetXNetworkManager extends GetxController { //this variable 0 = No Internet, 1 = connected to WIFI ,2 = connected to Mobile Data. int connectionType = 0; //Instance of Flutter Connectivity final Connectivity _connectivity = Connectivity(); //Stream to keep listening to network change state late StreamSubscription _streamSubscription ; @override void onInit() { GetConnectionType(); _streamSubscription = _connectivity.onConnectivityChanged.listen(_updateState); } // a method to get which connection result, if you we connected to internet or no if yes then which network Future<void>GetConnectionType() async{ var connectivityResult; try{ connectivityResult = await (_connectivity.checkConnectivity()); }on PlatformException catch(e){ print(e); } return _updateState(connectivityResult); } // state update, of network, if you are connected to WIFI connectionType will get set to 1, // and update the state to the consumer of that variable. _updateState(ConnectivityResult result) { switch(result) { case ConnectivityResult.wifi: connectionType=1; update(); break; case ConnectivityResult.mobile: connectionType=2; update(); break; case ConnectivityResult.none: connectionType=0; update(); break; default: Get.snackbar('Network Error', 'Failed to get Network Status'); break; } } @override void onClose() { //stop listening to network state when app is closed _streamSubscription.cancel(); } }
3. Getx initial Binding
Now, create one more dart file for bindings between GetXNetworkManager.dart and main.dart.
I have create it and named it as ‘NetworkBinding.dart’
This dart file, class extends Bindings & must have a @override method i.e. Dependenies(), which will then load our GetXNetworkManager class by using Get.LazyPut().
import 'package:get/get.dart'; import 'package:getx_check_internet/GetxNetworkManager.dart'; class NetworkBinding extends Bindings{ // dependence injection attach our class. @override void dependencies() { // TODO: implement dependencies Get.lazyPut<GetXNetworkManager>(() => GetXNetworkManager()); } }
4. main.dart file
In main.dart file , instead of simple MaterialApp, we are using GetMaterialApp, inside it we are using a property called as initialBinding that then invoke GetXNetworkManager class.
Then in stateful widget, we create an Instance of GetXNetworkManager(GetXController) class so that we can use properties of GetXNetworkManager from main.dart.
main.dart
import 'package:flutter/material.dart'; import 'package:get/get.dart'; import 'package:getx_check_internet/GetxNetworkManager.dart'; import 'package:getx_check_internet/NetworkBinding.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return GetMaterialApp( //Initiate Bindings we have created with GETX initialBinding: NetworkBinding() , title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { // create an instance final GetXNetworkManager _networkManager = Get.find<GetXNetworkManager>(); @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text('Network Status',style: TextStyle(fontSize: 20),), //update the Network State GetBuilder<GetXNetworkManager>(builder: (builder)=>Text((_networkManager.connectionType == 0 )? 'No Internet' : (_networkManager.connectionType == 1) ? 'You are Connected to Wifi' : 'You are Connected to Mobile Internet',style: TextStyle(fontSize: 30),)), ], ), ), ); } }
Output
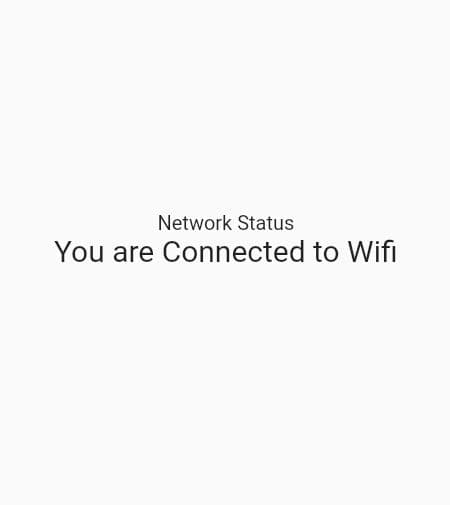