Hi Guys, Welcome to Proto Coders Point. In this dart tutorial we will learn what is Armstrong number & then let’s write a dark program to check if a given number is a armstrong number or not.
This are the basic program been asked in most of the coding interviews so freshers who are applying for job must know armstring program.
What is Armstrong number
A Armstrong number, when each of its digits is raised to the power, number of digits in a number & the sum of it will be same as the number is called as armstrong number.
Eg: Let's take a number '370'. 370, so we have 3 digit number, therefore, each of its digits in 370 will have power of 3. 370 = 33 + 73 + 03 = 27 + 343 + 0 = 370 It's a ArmStrong Number
Let’s Take one more Example(Not an Armstrong)
Let a Number be '1234'
1234, so it is a 4 digit armstrong number to be checked ok. Therefore each digit in 1234 will have power as 4.
1234 = 14 + 24 + 34 + 44
= 1 + 8 + 81 + 256
1234 != 346
It's not a Armstrong number.
Armstrong are also called as plus perfect or Narcissistic number.
Few Example on armstrong number
1: 11 = 1
2: 21 = 2
3: 31 = 3
371: 33 + 73 +13 = 371
407 = 43 + 03 + 73 = 407
1634: 14 + 64 + 34 + 44 = 1 + 1296 + 81 + 256 = 1643
8208 = 84 + 24 + 04 +84 =8208
Armstrong number program in dart
The following program will check if the given number is armstrong number or not.
import 'dart:io'; import 'dart:math'; void main(){ int? num; print('Enter A Number to check ARMSTRONG or NOT ARMSTRONG NUMBER'); // user will enter a number to check num = int.parse(stdin.readLineSync()!); // user entered number is passed to function isArmString(num); } //a function to check arm strong void isArmString(int number){ //initial some variable int temp,digits =0, last = 0, sum = 0; //assign user entered number to temp variable temp = number; // loop execute until temp is 0 and increment digits by 1 each loop while(temp>0){ temp = temp~/10; digits++; } //reset temp to user entered number temp = number; //another loop for getting sum while(temp>0){ last = temp % 10; sum = sum + pow(last, digits) as int; temp = temp~/10; } // now if number and sum are equal its a arm strong number if(number == sum){ print("IT'S A ARMSTRONG NUMBER"); }else{ print("IT'S NOT ARMSTRONG NUMBER"); } }
Result output
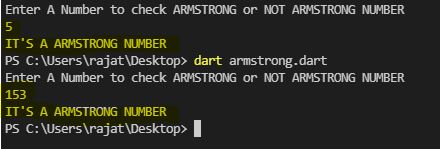
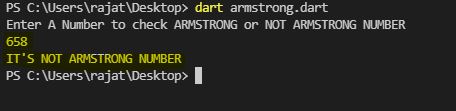
The below Dart Program will print all the ArmString number from 0 to specified input by user.
import 'dart:io'; import 'dart:math'; void main(){ int? num; print('Enter A Number to check ARMSTRONG or NOT ARMSTRONG NUMBER'); // user will enter a number to check num = int.parse(stdin.readLineSync()!); // user entered number is passed to function print('________________________________________-'); for(int i = 0;i<=num;i++) { //if i is arm strong then print it else don't if(isArmString(i)) { print(i); } } } //a function to check arm strong bool isArmString(int number){ //initial some variable int temp,digits =0, last = 0, sum = 0; //assign user entered number to temp variable temp = number; // loop execute until temp is 0 and increment digits by 1 each loop while(temp>0){ temp = temp~/10; digits++; } //reset temp to user entered number temp = number; //another loop for getting sum while(temp>0){ last = temp % 10; sum = sum + pow(last, digits) as int; temp = temp~/10; } // now if number and sum are equal its a arm strong number if(number == sum){ return true; }else{ return false; } }
result output
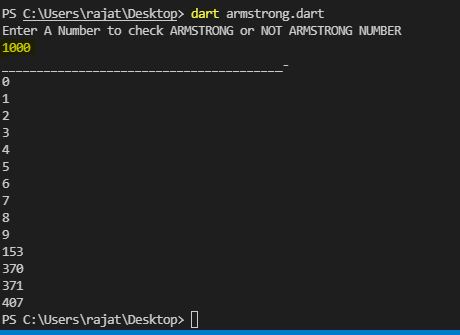
Recommended Dart programs
How to generate random number in dart