Hi Guys, Welcome to Proto Coders Point, In this Flutter dart article we will walk through How to convert an Object i.e. (Class Instance) into an JSON in Flutter dart.
1. Own Code to convert object to JSON without Library
The below example is manual way to convert object into JSON in flutter dart.
Below Steps:
- Create a class
- The class has a method to convert object into a Map.
- Finally convert the Map object to JSON String using JsonEncode() method.
Code
// main.dart import 'dart:convert'; // Define a class class User { final String userame; final int userage; final bool isMarried; User(this.userame, this.userage, this.isMarried); // Define a toJson method to convert User object to JSON Map<String, dynamic> toJson() { return { 'name': userame, 'age': userage, "isMarried": isMarried, }; } } void main() { User user = User('Shubham', 25, true); // Convert User object to JSON String jsonString = jsonEncode(user.toJson()); print(jsonString); }
{"name":"Shubham","age":25,"isMarried":true}
The object code is manual method is flexiable, It is self written code that can convert object into json string and it don’t use any external library or dependencies.
2. Convert Object to JSON using json_serializable package
We will make use of json_serialiable using which we can generate code from JSON serialization & deserialization.
Steps:
1. Install required dependencies:
we need:
To install all the above 3 package run below command:
flutter pub add json_annotation json_serializable build_runner
then run
flutter pub get
2. Now, In Lib directory let’s create a file by name “user.dart
” & then create class User & define it with @JsonSerializable() annotation example code below
import 'package:json_annotation/json_annotation.dart'; part 'user.g.dart'; @JsonSerializable() class User { String name; int age; // Use JsonKey to change the name of the field in JSON @JsonKey(name: 'is_admin') bool isAdmin; User(this.name, this.age, this.isAdmin); // The generated toJson method Map<String, dynamic> toJson() => _$UserToJson(this); // The generated fromJson method factory User.fromJson(Map<String, dynamic> json) => _$UserFromJson(json); }
3. user build_runner to generate JSON Serialization using below command
dart run build_runner build
By running above cmd a new file will get generated by name User.g.dart in the same directory where your class file exist.
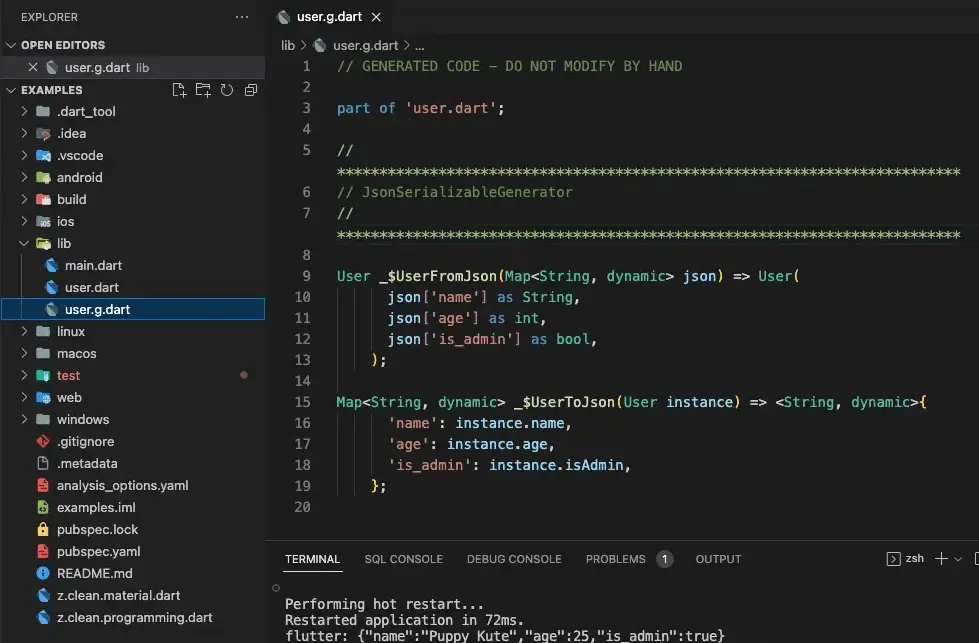
You not need to enter the above code or edit anything in User.g.dart, as the code get automatically ganerated by build_runner.
4. Now simply use the ganeted code function i.e toJson() method that help you convert Object to JSON, if you want you can also use fromJson() to convert JSON data to an Object.
Below Code example
// lib/main.dart import 'dart:convert' show jsonEncode; import 'package:flutter/foundation.dart' show kDebugMode; import 'user.dart'; void main() { // Create a user object User user = User('Puppy Kute', 25, true); // Convert the user object to JSON Map<String, dynamic> jsonUser = user.toJson(); // Print the JSON string if (kDebugMode) { print(jsonEncode(jsonUser)); // Prints: {"name":"Puppy Kute","age":25,"is_admin":true} } // Create a list of user objects List<User> users = [ User('Shubham', 40, false), User('Pavan', 50, true), User('Rajat', 28, false), ]; // Convert the list of user objects to a JSON string List<Map<String, dynamic>> jsonUsers = users.map((user) => user.toJson()).toList(); // Print the JSON string if (kDebugMode) { print(jsonEncode(jsonUsers)); } }
[{"name":"Shubham","age":40,"is_admin":false},{"name":"Pavan","age":50,"is_admin":true},{"name":"Rajat","age":28,"is_admin":false}]
Conclusion
In this article we learn 2 ways by which we can convert Object to JSON. One is by self written code and another is by using external library’s like JSON_Serializable and build_runner.
Similar Articles
How to read json file in flutter & display a listview
Auto Create Model from JSON File in Flutter