Hi Guys, Welcome to Proto Coders Point, In this Article we will learn how to create popup menu in flutter appbar.
Final App – Example on Popup dropdown menu item in appbar
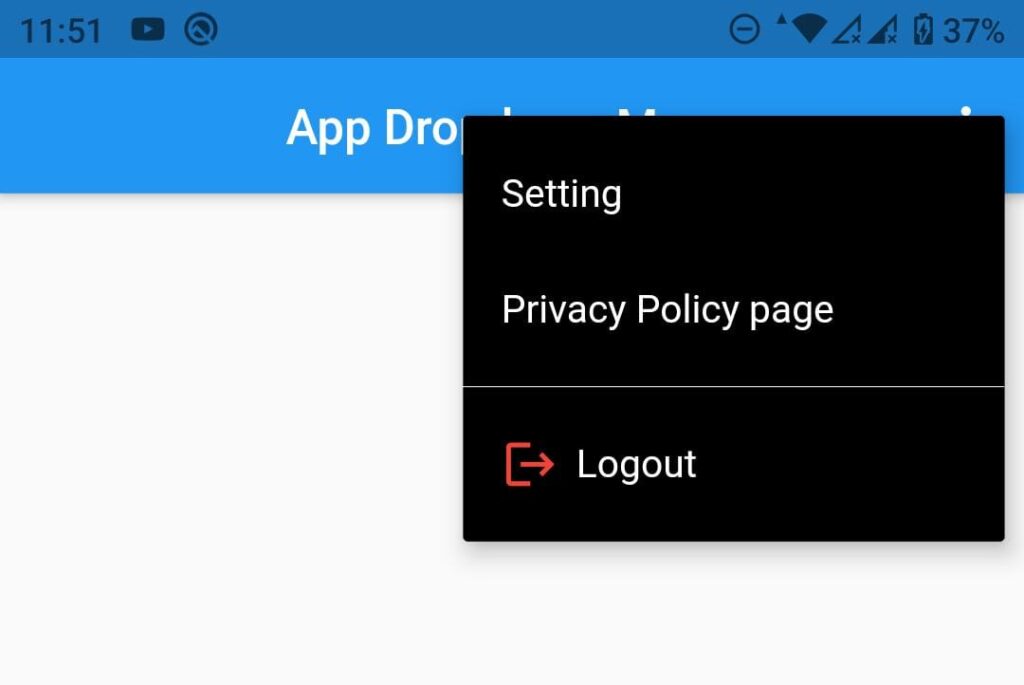
As you can see in the above app screenshot, I have a menu item on the app bar, Which contains 3 menus that a user can select ‘Setting‘, ‘Privacy policy‘, and a ‘Logout‘ menu.
How to create popup menu in flutter appbar
video tutorial
In Flutter we can easily create appbar menu by using ‘actions’ properties of flutter appbar. (To learn more about appbar visit official site here)
appBar: AppBar( centerTitle: true, title: Text("App Dropdown Menu"), actions: [ //list of widget you want to show in appbar ], ),
Easiest way to add 3 dot popup menu in appbar
The simplistic way to add 3 dot popup menu is by using flutter Appbar actions:[] properties.
A Appbar actions properties is a array that accept list of widget, In our case we are using PopupMenuButton to show PopupMenuItem.
Here is an snippet code of PopupMenuButton
appBar: AppBar( centerTitle: true, title: Text("App Dropdown Menu"), actions: [ //list if widget in appbar actions PopupMenuButton( icon: Icon(Icons.menu), //don't specify icon if you want 3 dot menu color: Colors.blue, itemBuilder: (context) => [ PopupMenuItem<int>( value: 0, child: Text("Setting",style: TextStyle(color: Colors.white),), ), ], onSelected: (item) => {print(item)}, ), ], ),//appbar end
Explanation of above snippet code
Above App bar in actions properties which accept widgets, there we are placing PopupMenuButton Widget, In PopMenuButton there is a required property i.e. itemBuilder.
itemBuilder(): is a context that builds list of PopupmenuItem.
PopupMenuItem widget has 2 propeties i.e. value & child
value: is used to set an Id to a menuItem, so that when the user selects the menu item, we can detect which menu item was clicked by the user.
child: this is just to show a widget like Text in the particular menuItem.
How to use onTap or onPressed in PopupMenuItem
In PopupMenuButton there a property called onSelected: (value), which keep track of which item is been selected by the user from menuItem.
Here is an explanation on the same
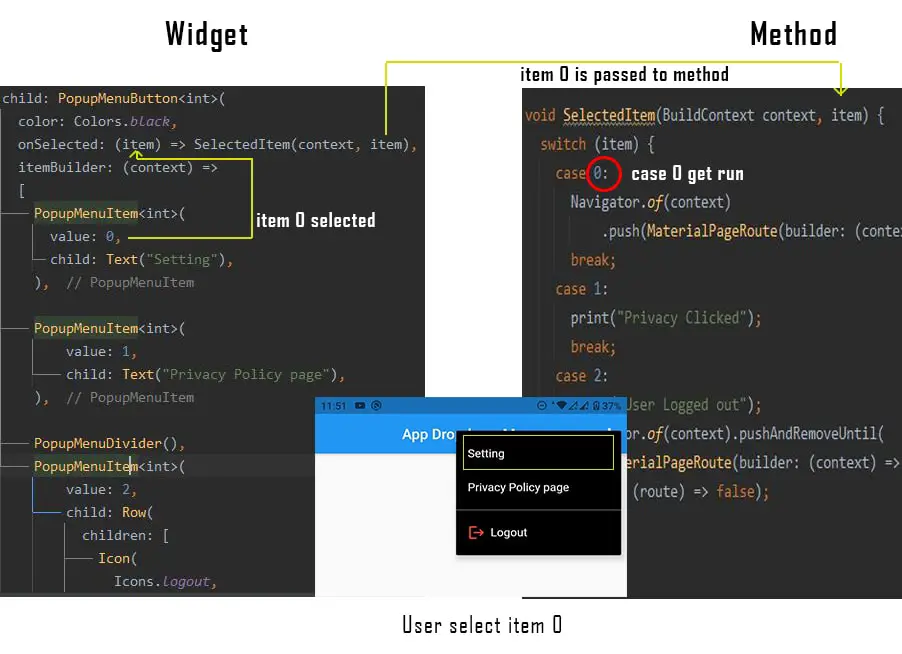
Complete Source Code of Flutter Appbar with Menu Items
LoginPage.dart
import 'package:flutter/material.dart'; class LoginPage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("This is Login Page"), ), ); } }
SettingPage.dart
import 'package:flutter/material.dart'; class SettingPage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("This is Setting Page"), ), ); } }
main.dart
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:flutter_app_simple/LoginPage.dart'; import 'package:flutter_app_simple/SettingPage.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( centerTitle: true, title: Text("App Dropdown Menu"), actions: [ Theme( data: Theme.of(context).copyWith( textTheme: TextTheme().apply(bodyColor: Colors.black), dividerColor: Colors.white, iconTheme: IconThemeData(color: Colors.white)), child: PopupMenuButton<int>( color: Colors.black, itemBuilder: (context) => [ PopupMenuItem<int>(value: 0, child: Text("Setting")), PopupMenuItem<int>( value: 1, child: Text("Privacy Policy page")), PopupMenuDivider(), PopupMenuItem<int>( value: 2, child: Row( children: [ Icon( Icons.logout, color: Colors.red, ), const SizedBox( width: 7, ), Text("Logout") ], )), ], onSelected: (item) => SelectedItem(context, item), ), ), ], ), body: Container(); ); } void SelectedItem(BuildContext context, item) { switch (item) { case 0: Navigator.of(context) .push(MaterialPageRoute(builder: (context) => SettingPage())); break; case 1: print("Privacy Clicked"); break; case 2: print("User Logged out"); Navigator.of(context).pushAndRemoveUntil( MaterialPageRoute(builder: (context) => LoginPage()), (route) => false); break; } } }
Output
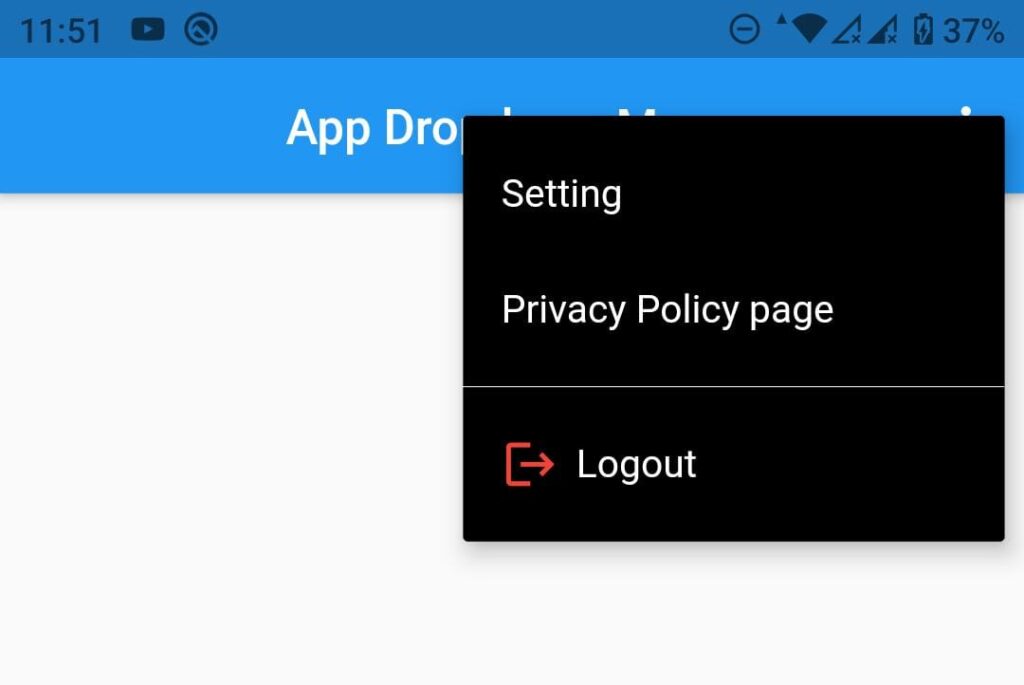