Hi Guys, Welcome to Proto Coders Point, In this flutter tutorial we will learn how to use font awesome animated Icons class to show/display animated icons in a flutter.
Icons in flutter
In Flutter we have different kinds of Icons that we can use for free to create a beautiful app UI.
- Static Icons: In static there are 2 kinds of those are
Material Icon: Icon(Icons.add,size:100);
Cupertino Icon: Icon(CupertinoIcon.moon_stars,size:100);
So with both this flutter static icons i.e. Material Icon and CupertinoIcon you can make use of 1000’s of icons for your flutter UI design. - Animated Icons:
Then secondly we have our font awesome animated icons that are a pre-defined set of animate Icons that we can use off.
Introducation to flutter animated Icons
In flutter, you can use awesome animated icons that show animation effects in icons while moving/changing from one icon to another icon & also back to the previous one.
The Flutter Animated Icon class is been used to create a simple icon transition between two icons in the flutter.
There are many pre-defined Animated Icons in the flutter class, that you can use to create a very beautiful user interface, so if the user clicks on an icon then it will be a transition to another icon.
A Good Example is a music player app that has a play-pause button.
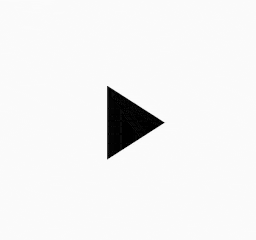
Video Tutorial on Animated Icons In Flutter with Example
1. How to use animated icon widget & controller for it
Widget snippet code
AnimatedIcon( icon: AnimatedIcons.play_pause, progress: iconController, // a animation controller is need to control icon animation size: 150, color: Colors.green, ),
Controller
late AnimationController iconController; // make sure u have flutter SDK > 2.12.0 (null safety) as we are using late keyword @override void initState() { super.initState(); //create an instance of Controller and define a duration of 1 second. iconController = AnimationController(vsync: this,duration: Duration(milliseconds: 1000)); // the below code will perform forword animation then then after 1 sec reverse the animation transition iconController.forward().then((value) async { await Future.delayed(Duration(seconds: 1)); iconController.reverse(); }); }
In the above controller code, we are creating an instance object of AnimationController i.e. ‘iconController & initializing its duration to 1 second.
Then immediatly we are giving a transition animation effect to icon, by using controller.forword() to change icon from play to pause & then after 1 second delay, we are again changing the animation back to normal by using controller.reverse().
2. OnTap on Icon to Play Transition animation
Animated Icon with GestureDetector widget
GestureDetector( onTap: (){ AnimateIcon(); // call below method to start icon animation }, child: AnimatedIcon( icon: AnimatedIcons.play_pause, //pre-defined icon // use any progress: iconController, //attached animation controllder size: 150, color: Colors.green, ), ),
Method & Animation Controller
late AnimationController iconController; // make sure u have flutter sdk > 2.12.0 (null safety) bool isAnimated = false; @override void initState() { super.initState(); iconController = AnimationController(vsync: this,duration: Duration(milliseconds: 1000)); } //method called when user tap on icon void AnimateIcon{ setState(() { isAnimated = !isAnimated; isAnimated ? iconController.forward() : iconController.reverse(); }); } // good practice to dispose controller when not in use @override void dispose() { // TODO: implement dispose iconController.dispose(); super.dispose(); }
In Above snipper code, i have:-
A Variable type boolean ‘isAnimated = false’, used to keep track of Animation Transition state.
In initState(), I am creating an instance object of Animation Controller class with an animation duration of 1000 milliseconds i.e. 1 second, and then the controller object is connected to widget i.e. AnimatedIcon( progress: iconController); as you see above
Then there is a method void AnimateIcon() which is been called when AnimatedIcon is been pressed/onTap. In that method first, we are setting isAnimated to true or false.
Then Performing transition using iconController.forword() & iconController.reverse();
If isAnimated is true then perform forword transition & if isAnimated is false then perform reverse transition.
& finally a good practice is to dispose the controller when the app is been closed, so dispose the controller in void dispose @override method.
Flutter Animated Icons Example – Complete Source Code
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> with SingleTickerProviderStateMixin { late AnimationController iconController; // make sure u have flutter sdk > 2.12.0 (null safety) bool isAnimated = false; @override void initState() { // TODO: implement initState super.initState(); iconController = AnimationController(vsync: this,duration: Duration(milliseconds: 1000)); iconController.forward().then((value) async { await Future.delayed(Duration(seconds: 1)); iconController.reverse(); }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( centerTitle: true, title: Text("App Dropdown Menu"), ), body: Center( child: GestureDetector( onTap: (){ AnimateIcon(); }, child: AnimatedIcon( icon: AnimatedIcons.play_pause, progress: iconController, size: 150, color: Colors.green, ), ), ) ); } void AnimateIcon(){ setState(() { isAnimated = !isAnimated; //if false then change it to true, likewise if true change it to false //if true then forword else reverse isAnimated ? iconController.forward() : iconController.reverse(); }); } @override void dispose() { // implement dispose iconController.dispose(); super.dispose(); } }
Output
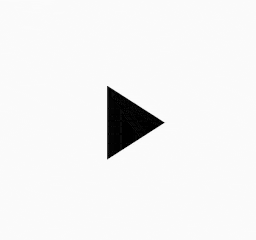