Hi Guys, Welcome to Proto Coders Point, In this Flutter Tutorial we will discuss & implement 2 different flutter plugin/library, that is been used to ask use to 5 star rating review in our flutter application.
I have found best rating plugin in flutter
- smooth star rating flutter
- rating_dialog – flutter app rating dialog
Video Tutorial
1. Flutter Smooth Star rating ( library 1)
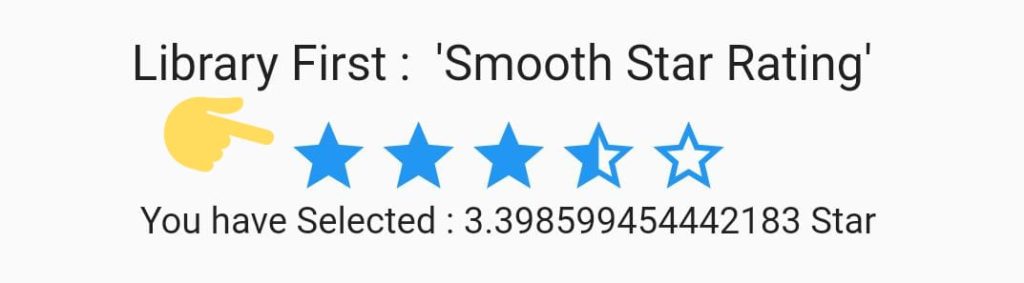
This library is the best library as it comes with a star rating with touch and even it enables to swipe rating to star review. It’s named as smooth star rating flutter because this library will detect this gesture you make on the flutter star rating icon to give the rating.
This are the feature of this library:
Change the default star icons with your desired Icons.
Give user to rate using half rate or full ( Eg : 2.5 rating or 4.5 rating )
swiping on icon will increment/decrement the rating bar star.
and many more feature.
To learn more visit official site here
- Supports replacing default star icons with desired IconData
- Supports half rate and full rate (1.0 or 0.5)
- Swipe for incrementing/decrementing rate amount
- Change star body and boundary colors independently
- Control size of the star rating
- Set your desired total Star count
- Supports click-to-rate
- Spacing between stars
Installation smooth star rating
Add dependencies
open pubspec.yaml and all the following dependencies line.
dependencies: smooth_star_rating: ^1.0.4+2 // add this line
Then, just click on package_get this will download all the required classes in your flutter project.
Import the package class file
Then, after adding the library, you need to import the smooth_star_rating.dart wherever you need to show star review rating bar.
import 'package:smooth_star_rating/smooth_star_rating.dart';
Snippet code of showing star rating widget
SmoothStarRating( rating: rating, size: 35, filledIconData: Icons.star, halfFilledIconData: Icons.star_half, defaultIconData: Icons.star_border, starCount: 5, allowHalfRating: false, spacing: 2.0, onRatingChanged: (value) { setState(() { rating = value; }); }, ),
Different parameters you can use here:
allowHalfRating - Whether to use whole number for rating(1.0 or 0.5) onRatingChanged(int rating) - Rating changed callback starCount - The maximum amount of stars rating - The current value of rating size - The size of a single star color - The body color of star borderColor - The border color of star spacing - Spacing between stars(default is 0.0) filledIconData - Full Rated Icon halfFilledIconData - Half Rated Icon defaultIconData - Default Rated Icon
2. Flutter Rating Dialog ( Library 2 )
This flutter rating dialog is awesome as it provide beautiful and customizable Rating star icon dialog package for flutter application development.
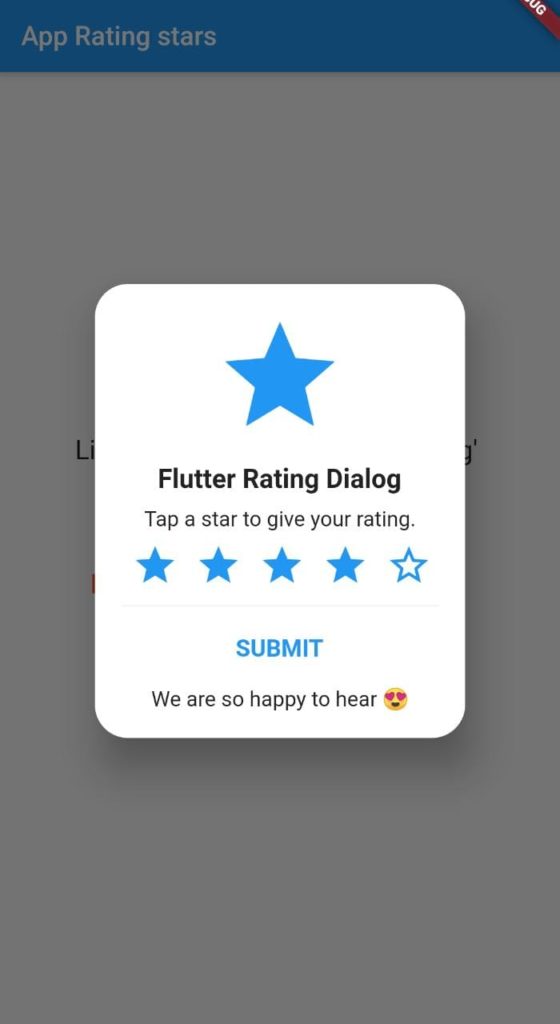
Installation of Flutter rating dialog plugin
Adding depencencies
Open pubspec.yaml file and all the below raiting dialog depencencies
dependencies: rating_dialog: ^1.0.0 // add this line
Then, just click on package_get this will download all the required classes in your flutter project.
Import the package class file
Then, after adding the library, you need to import the rating_dialog.dart whereever you need to show rating dialog box.
import 'package:rating_dialog/rating_dialog.dart';
Snippet Code to show AlertDialogin flutter with rating dialog
void show() { showDialog( context: context, barrierDismissible: true, // set to false if you want to force a rating builder: (context) { return RatingDialog( icon: const Icon( Icons.star, size: 100, color: Colors.blue, ), // set your own image/icon widget title: "Flutter Rating Dialog", description: "Tap a star to give your rating.", submitButton: "SUBMIT", alternativeButton: "Contact us instead?", // optional positiveComment: "We are so happy to hear 😍", // optional negativeComment: "We're sad to hear 😭", // optional accentColor: Colors.blue, // optional onSubmitPressed: (int rating) { print("onSubmitPressed: rating = $rating"); // TODO: open the app's page on Google Play / Apple App Store }, onAlternativePressed: () { print("onAlternativePressed: do something"); // TODO: maybe you want the user to contact you instead of rating a bad review }, ); }); }
The above snippet code has a method show() which have showDialog() widget that will return/display RatingDialog() which is a class of this library.
RatingDialog() widget have various parameters or we can say features.
icon : when you can display your flutter app logo
title: basically to title text
description : text to ask user for there valuable star reviews.
submitButton : will show a simple button for submission of the review.
alternativeButton : you may use this button to navigate user to URL of your company website to know more.
positiveComment : if you select more the 3 star rating then you can show a positive message to user.
negativeComment: if you select 3 or less star rating then you can show different message to user.
onSubmitPressed(){} : what action to be performed when used click of submit review button
onAlternativePressed(){} : where the used should be navigated when user click on more info button.
Complete Source Code with above 2 rating bar dialog example in flutter
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:smooth_star_rating/smooth_star_rating.dart'; import 'package:rating_dialog/rating_dialog.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { double rating = 4.0; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text("App Rating stars")), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( "Library First : 'Smooth Star Rating' ", style: TextStyle(fontSize: 20), ), SizedBox( height: 10, ), SmoothStarRating( rating: rating, size: 35, filledIconData: Icons.star, halfFilledIconData: Icons.star_half, defaultIconData: Icons.star_border, starCount: 5, allowHalfRating: true, spacing: 2.0, onRatingChanged: (value) { setState(() { rating = value; print(rating); }); }, ), Text( "You have Selected : $rating Star", style: TextStyle(fontSize: 15), ), SizedBox( height: 15, ), Text( "Library Second: 'Rating_Dialog ' ", style: TextStyle(fontSize: 20, color: Colors.deepOrange), ), RaisedButton( onPressed: () { show(); }, child: Text("Open Flutter Rating Dialog Box"), ) ], ), )); } void show() { showDialog( context: context, barrierDismissible: true, // set to false if you want to force a rating builder: (context) { return RatingDialog( icon: const Icon( Icons.star, size: 100, color: Colors.blue, ), // set your own image/icon widget title: "Flutter Rating Dialog", description: "Tap a star to give your rating.", submitButton: "SUBMIT", alternativeButton: "Contact us instead?", // optional positiveComment: "We are so happy to hear 😍", // optional negativeComment: "We're sad to hear 😭", // optional accentColor: Colors.blue, // optional onSubmitPressed: (int rating) { print("onSubmitPressed: rating = $rating"); // TODO: open the app's page on Google Play / Apple App Store }, onAlternativePressed: () { print("onAlternativePressed: do something"); // TODO: maybe you want the user to contact you instead of rating a bad review }, ); }); } }
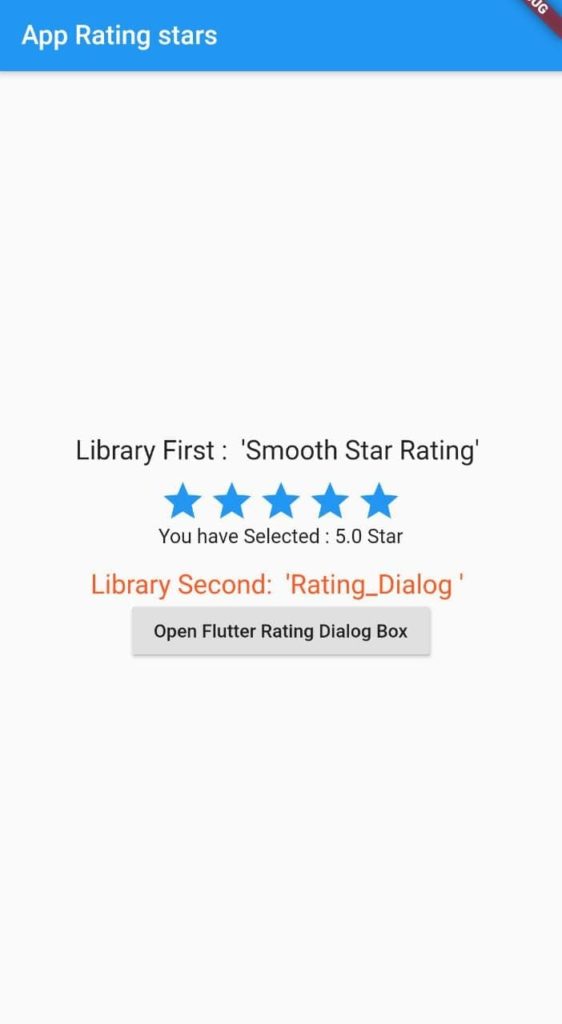
Recommended Articles
bottom popup android – bottom sheet dialog fragment