Hi Guys, Welcome to Proto Coders Point, In this Flutter Article let’s check how to become a professional flutter developer by just following this coding rules in flutter.
How to become a professional flutter developer
1. Always use a const keyword for static data.
When any widget using static data which will never change in runtime , it’s better to use const on those widget or dataType.
return Scaffold( body: Container( child: Column( children: const [ Text("This is static Text"), Text("so use const keyword ") // Always use a const for static type data ], ), ) );
2. Use right widget as right place.
Below Example is not the right way to give padding.
Container( padding: EdgeInsets.all(10), child: Text("This is static Text"), )
Flutter app is app an widget, but using right widget matters. In Above Example, I am using Container widget just to give padding to a child does not make sense right, Flutter has padding widget use that instead.
Padding( padding: const EdgeInsets.all(10.0), child: Text("This is static Text"), )
3. Make use of setState() properly for rebuild update.
If you are not using any state management plugin like flutter bloc, provider, GetX. They you might be using setState to rebuild the widget tree to update the date update.
Basically setState() will revuild the whole widget treee, just to make a small change in textField.
Instead create a seperate widget and use StatefulBuilder so that on setState only the particular widget tree get rebuild instead of whole widget tree.
Example:
class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { int _counter = 0; // Do @override Widget build(BuildContext context) { return Scaffold( body: Column( children: [ Text('${_counter}'), ElevatedButton( onPressed: () => { setState(() { _counter++; // this will rebuild whole widget tree }) }, child: Text("Click")), NewWidget() ], )); } Widget NewWidget() { return StatefulBuilder( builder: (BuildContext context, StateSetter setState) { return Column( children: [ Text('${_counter}'), ElevatedButton( onPressed: () => { setState(() { _counter++; // on button click only this widget will get updated }) }, child: Text("Click")) ], ); }, ); } }
4. Use private variable/method.
Keep habit of using private variable. In flutter Dart doesn’t have the keywords like public, protected, and private. If an identifier starts with an underscore _
, it’s private variable in dart.
This is not the right way
class Registration{ String name,email,phone; Registration( { required this.name, required this.email, required this.phone }); }
The Right way to use provide variable
class Registration{ String _name; String _email; String _phone; Registration( { required String name, required String email, required String phone }) : _name = name, _email = email, _phone = phone; }
5. Create seperate Method for event.
Avoid using implementing method or any event inside a widget itself, It’s not a good practice in flutter application development For Eg: Performing any event or action when user press a button, It Ok if event is a small task, if it a long code on event like calling a API to get data from server then it better create a seperate method outside a widget.
Example:
class _MyHomePageState extends State<MyHomePage> { int _counter = 0; void _doSomeThing(){ print("perform your button press action here"); } @override Widget build(BuildContext context) { return Scaffold( body: Column( children: [ Text('${_counter}'), ElevatedButton( onPressed: ()=> _doSomeThing(), child: Text("Click")), ], )); } }
6. Use Nullable the right way.
THe best feature of dart language is null safety I wrote a complete article on flutter null safety check it out to learn in detail
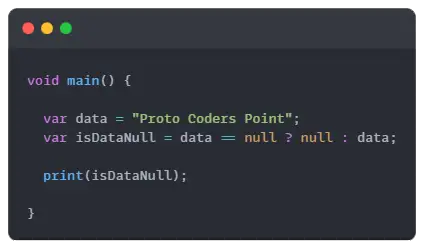
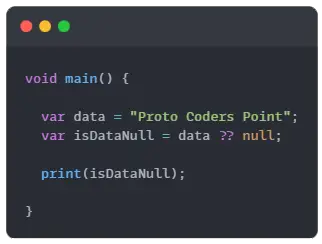
7. Internalization your flutter app.
Avoid using Hardcoded string in app. By implementing Internationalization in flutter app at the beginning of development is better, because one your app starts to grow, then to update small changed in-app then in one place you can easily search and update/replace the string easily.
Example:
Don’t do
Text("This is hard coded Text")
Text( AppLocalizations.of(context)!.AppName, // )
8. Don’t use var at all declaration.
use exact datatype, when you know it value type.
Exmaple: if a variable is of type number then it better to use int instead of var.
Eg:
String name = 'Rajat';
int age = 26;
9. Keep Flutter Theme & Page Routes in seperate file.
class MyApp extends StatelessWidget { var themeSetting = ThemeData( // Always Create a select variable to device Theme primarySwatch: Colors.blue, textTheme: TextTheme(), primaryColor: Colors.green ); @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter', theme: themeSetting, home: const MyHomePage(), ); } }
10. Use stateless widget the right way.
using StatefulWidget, unnecessarily all over the flutter app is not the right way to build flutter application, Use Stateless Widget where there are not rebuild or update required (widget data is fully static).
11. Don’t forget retrun type declaration in functions
Always give a return type to function
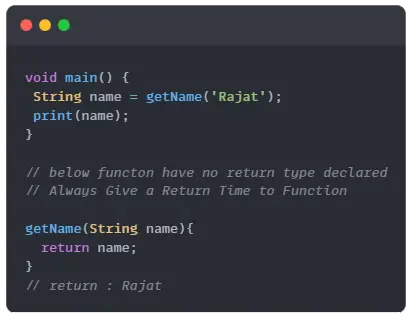
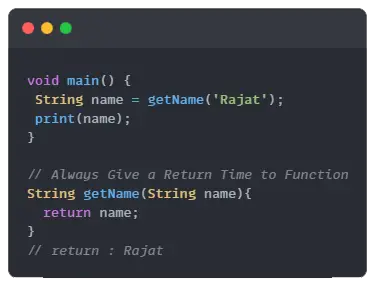
12. To Compose String use Interpolation
With string variable instead of using “+” to concate strings in variable, make use of interpolation in dart language.
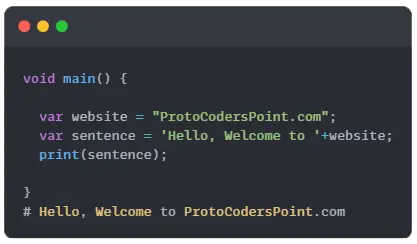
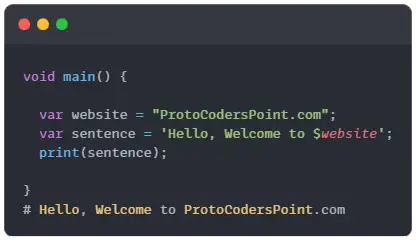
13. using cascade operator in dart
With one object when you run sequence of operation make use of cascade operation (..) double dot operators.
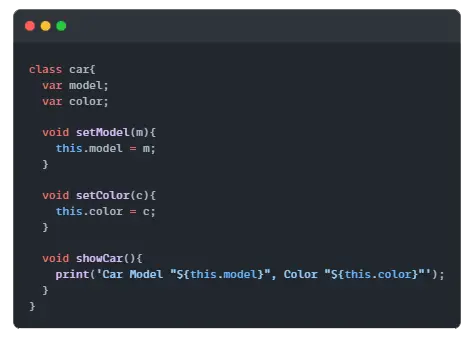
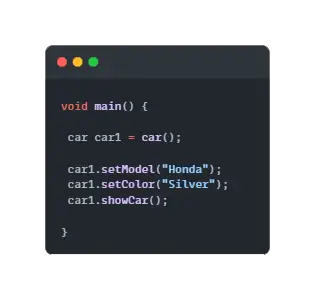
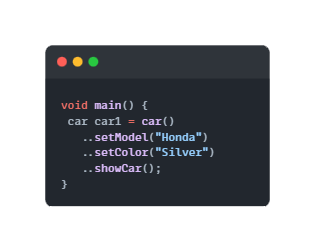
14. Giving naming convention in dart the right way
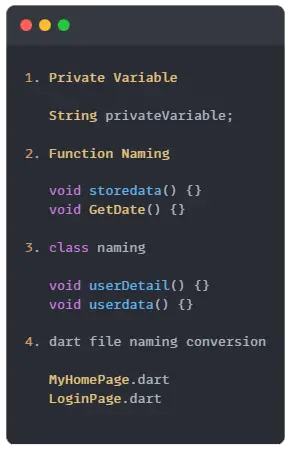
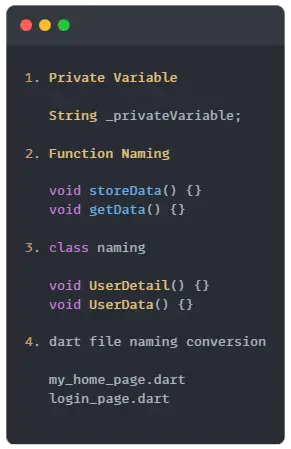