In This post as you know we are learning how to implement a scan qr code in android android studio where we are making use of zbar or zxing any of this you can user as both of them are similar.
I am making use of zbar library scan qr code android-studio.
Introduction on QR Code
Each and Every barcode contains unique identity number format which are used to represents the product id based. Using this qr code scanner app, you will be able to find out that id from the barcode/qr code by scanning it.
In the end of this tutorial, our Android app will be able to scan both Barcode as well as QRCode.
qr scanner app for android-studio full source code.
Create a new project in Android Studio.
When you are creating a new qr code project, select empty activity as a default activity in android-studio.
File>new Project > select Empty Activity >Give a Project Name
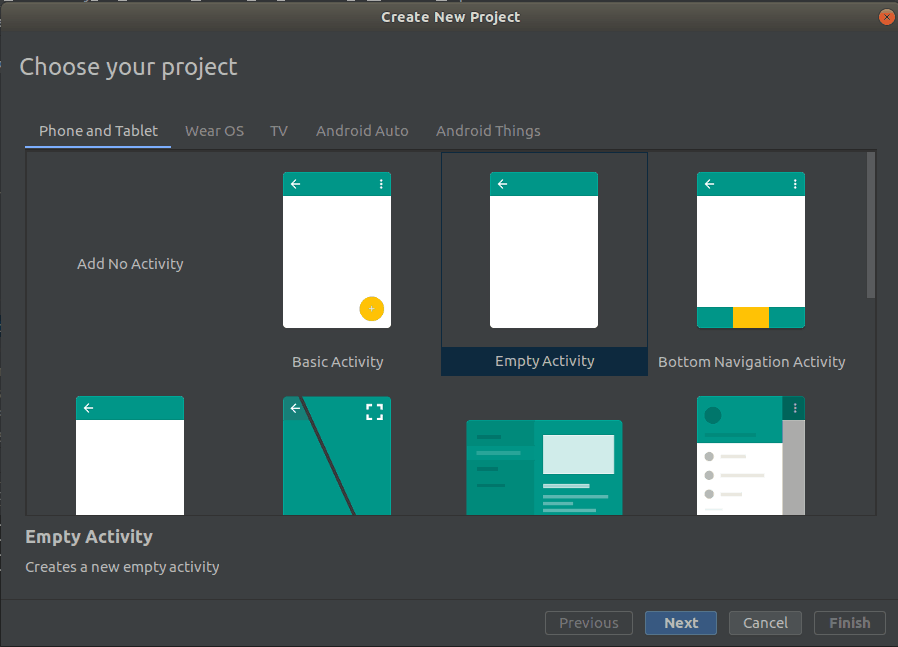
Add dependencies in build.gradle(Module:app) file
Add the following dependency to your build.gradle (Module : app) file.
implementation 'me.dm7.barcodescanner:zbar:1.9.13'
Here i am using ZBar github library , but you can also use ZXing.
Add camera permission to your AndroidManifest.xml file:
You need to add camera permission between <manifest>….</manifest> tag in androidManifest.xml file .
<uses-permission android:name="android.permission.CAMERA" />
For using camera, we have to get permission from the user device.
Note: If you are targeting SDK version above 22 (Above Lollipop) then you need to ask a user for granting runtime permissions.
Updating activity_main.xml file:
Designing a user Interface here i have made use of one button and a text view
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_gravity="center" android:gravity="center"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:text="QR Code Scanner" android:gravity="center" android:textSize="25sp" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/btn" android:text="Scan Barcode or QR code" /> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/tvresult" android:textColor="#000" android:layout_gravity="center" android:gravity="center" android:layout_marginTop="10dp" android:textAppearance="?android:attr/textAppearanceMedium" android:text="Result will be here"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margin="10dp" android:text="For more programming tutorial keep visiting" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="www.protocoderspoint.com" android:textColor="#039BE5" /> </LinearLayout>
Here Button will open a new activity with camara for scanning a bar code or QR code a and After successful scanning, system will enter the value of the barcode or QR code in the textview.
Updating MainActivity.java file:
import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; public class MainActivity extends AppCompatActivity { public static TextView tvresult; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); tvresult = (TextView) findViewById(R.id.tvresult); Button btn = (Button) findViewById(R.id.btn); btn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent intent = new Intent(MainActivity.this, ScanActivity.class); startActivity(intent); } }); } }
In the above MainActivity.java code we have to initialize a textview and button.
On Button click we are loading a new scanActivity.java code using Intent method. In short Here we will open ScanActivity on the button click.
Opening new activity ScanActivity.java in android studio
Now go to File->New->Activity->Empty Activity and give name of activity as ScanActivity.
Add below code to ScanActivity.java
package protocoderspoint.com.qrcodescannerapp; import android.os.Bundle; import android.util.Log; import android.widget.Toast; import androidx.appcompat.app.AppCompatActivity; import me.dm7.barcodescanner.zbar.ZBarScannerView; public class ScanActivity extends AppCompatActivity implements ZBarScannerView.ResultHandler { private ZBarScannerView mScannerView; //camera permission is needed. @Override public void onCreate(Bundle state) { super.onCreate(state); mScannerView = new ZBarScannerView(this); // Programmatically initialize the scanner view setContentView(mScannerView); // Set the scanner view as the content view } @Override public void onResume() { super.onResume(); mScannerView.setResultHandler(this); // Register ourselves as a handler for scan results. mScannerView.startCamera(); // Start camera on resume } @Override public void onPause() { super.onPause(); mScannerView.stopCamera(); // Stop camera on pause } @Override public void handleResult(me.dm7.barcodescanner.zbar.Result result) { // Do something with the result here Log.v("Result 1", result.getContents()); // Prints scan results Log.v("Result 2", result.getBarcodeFormat().getName()); // Prints the scan format (qrcode, pdf417 etc.) MainActivity.tvresult.setText(result.getContents()); onBackPressed(); // If you would like to resume scanning, call this method below: //mScannerView.resumeCameraPreview(this); } }
Here is Brief Description on ScanActivity.java
- In onCreate() method, we have set view as scannerview instead of xml file layout Because we are using Zbar QR Code Library.
- In onResume() method, we have set the resulthandler and started camera preview.
- In onPause() method, we are stopping camera preview.
Explaining handleResult() method of Zbar and zxing QR Code scanner
@Override public void handleResult(me.dm7.barcodescanner.zbar.Result result) { // Do something with the result here Log.v("kkkk", result.getContents()); // Prints scan results Log.v("uuuu", result.getBarcodeFormat().getName()); // Prints the scan format (qrcode, pdf417 etc.) MainActivity.tvresult.setText(result.getContents()); onBackPressed(); // If you would like to resume scanning, call this method below: //mScannerView.resumeCameraPreview(this); }
- As you can see in above code, we get result object which contains Data of Barcode or QRcode after scanning is completed.
- We will get the final result by running result.getContents() method. This method returns the value of barcode in text format.
- we have set this barcode text into TextView of MainActivity.
- onBackPressed() method will return the user to the Main Activity.
So This Tutorial was all about scan barcode and QRcode programmatically in android studio example. Thank you
QR code reader/scanner android source code download
Download a Complete QR coder scanner Source code
Download from DriveOr Refer official Github Zbar / Zxing QR code scanner Library’s
Download from GitHub
[…] Check out : QR scanner app development in android […]