Hi Guys, Welcome to Proto Coders Point, In this flutter Article let’s learn How to make Get/Post request in flutter app by using GetX package GetConnect() class.
What is GetX in Flutter
In Flutter GetX is an multi functiional powerful library which is open source to use in flutter app.
GetX provides massive high performance in teams of state management in flutter, route management, dependencies injection & basic functionalities such as showing snackbar, dialog Box popup, getStorage for storing data as mini database, Internationalization & much more can be used by just adding one line of code.
Although flutter getx has multiple feature in it, Here GetX library is built in such a way that each feature has it’s separate container & thus only the used once in your flutter app is been compiled.
Therefore, Getx library is very light weight as it does not increase app size much.
GET/POST request in flutter
In flutter app, we can make internet calls i.e GET/POST request by using GETX GetConnect class, you will be suprised by the lines of code your need to write to make Get/Post request.
Step to make GET/POST request using GETX Flutter library
1. Install GetX Dependencies
In your flutter project to use GetX you need to install it , Run below commond in IDE terminal
flutter pub add get
2. Import get.dart
Now, Once getx package is added as dependenies library, to use it, import where require Eg: main.dart
import 'package:get/get.dart';
3. Create Instance of GetConnect Class
Now, you need an instance of GetConnect() class. Let’s create it.
final _getConnect = GetConnect();
now, use this GetConnect instance to make GET/POST requests.
4. A Function that makes GET Request
void _sendGetRequest() async { final response = await _getConnect.get('API URL Here'); if (kDebugMode) { print(response.body); } }
5. A Function That makes POST request
void _sendPostRequest() async { final response = await _getConnect.post( 'http://192.168.29.167:3000/register', // rest api url { 'username': 'rajat123@gmail.com', 'password': '554433#221100', }, ); if (kDebugMode) { print(response.body); } }
Flutter Get/Post Request – Complete Example
I have created a backend NodeJS API, Which simply stores the data in Mongodb database when API Router is been triggered from frontend.
In this Article our frontend is flutter app, which has 2 TextField (username & password) and a Button (basic registration form), when button clicked, data entered in TextField is been sent through POST method of Flutter GetX library to backend NodeJS server which while store the entered data into MongoDB database.
api call using getx flutter
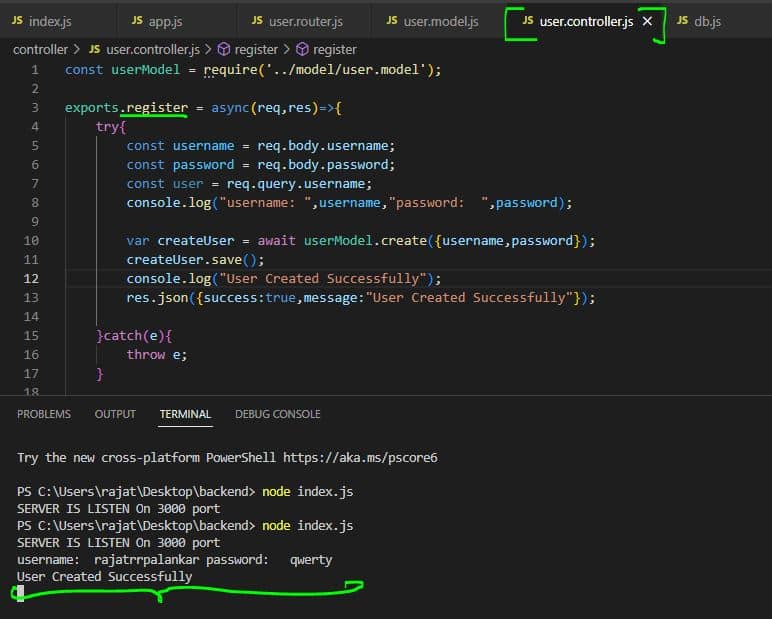
Get NodeJS Backend code from my github repository
MongoDB data stored screenshot
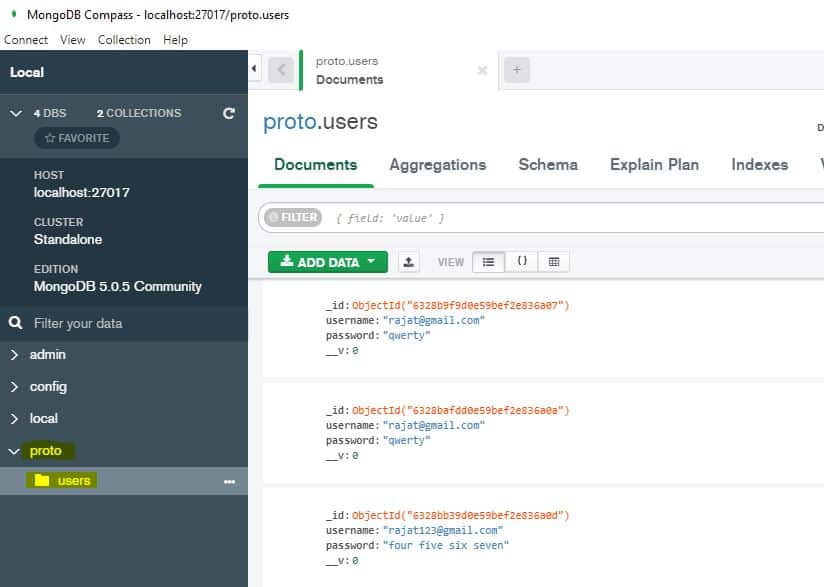
Flutter App Complete Source Code
main.dart
import 'package:flutter/foundation.dart'; import 'package:flutter/material.dart'; import 'package:get/get.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { final _getConnect = GetConnect(); final TextEditingController emailText = TextEditingController(); final TextEditingController passwordText = TextEditingController(); // send Post request void _sendPostRequest(String email,String password) async { final response = await _getConnect.post( 'http://192.168.29.167:3000/register', { 'username': email, 'password': password, }, ); if (kDebugMode) { print(response.body); } } @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Padding( padding: EdgeInsets.all(30), child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ TextField( controller: emailText, decoration: InputDecoration( border: OutlineInputBorder(), labelText: "Enter Email Or Username", ), ), SizedBox(height: 5,), TextField( controller: passwordText, decoration: InputDecoration( border: OutlineInputBorder(), labelText: "Enter Password", ), ), ElevatedButton( onPressed: ()=>_sendPostRequest(emailText.text,passwordText.text), child: const Text('Register')), ], ), ), ), ); } }