Hi Guys, Welcome to Proto Coders Point. In this article, we’ll learn about how to use Bootstrap in React.
Basically, Bootstrap is an open-source and free CSS framework focused on responsive, mobile-first front-end web development. It contains CSS and JavaScript-based design templates for typography, forms, buttons, navigation, and other interface components.
There are multiple ways of using bootstrap in react:-
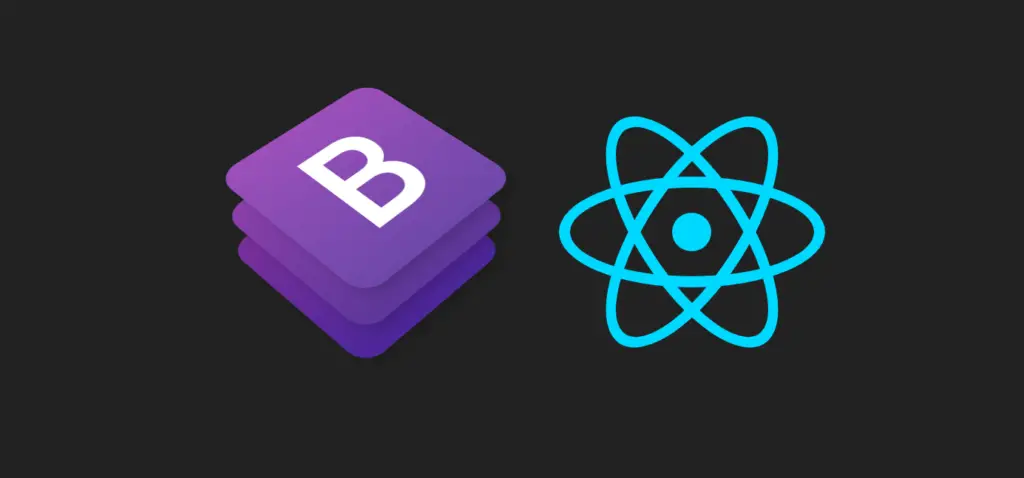
- Downloading Bootstrap and Manually importing as a dependence, Using Bootstrap CDN
- Using React Bootstrap npm package
Downloading Bootstrap and Manually importing as a dependency
You can add BootstrapCDN using as a link to your index.html file in the public folder of your React application.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <meta name="theme-color" content="#000000"> <!-- manifest.json provides metadata used when your web app is added to the homescreen on Android. See https://developers.google.com/web/fundamentals/engage-and-retain/web-app-manifest/ --> <link rel="manifest" href="%PUBLIC_URL%/manifest.json"> <link rel="shortcut icon" href="%PUBLIC_URL%/favicon.ico"> <!-- Notice the use of %PUBLIC_URL% in the tags above. It will be replaced with the URL of the `public` folder during the build. Only files inside the `public` folder can be referenced from the HTML. Unlike "/favicon.ico" or "favicon.ico", "%PUBLIC_URL%/favicon.ico" will work correctly both with client-side routing and a non-root public URL. Learn how to configure a non-root public URL by running `npm run build`. --> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.1.0/css/bootstrap.min.css" integrity="sha384-9gVQ4dYFwwWSjIDZnLEWnxCjeSWFphJiwGPXr1jddIhOegiu1FwO5qRGvFXOdJZ4" crossorigin="anonymous"> <title>React App</title> </head> <body> <noscript> You need to enable JavaScript to run this app. </noscript> <div id="root"></div> <!-- This HTML file is a template. If you open it directly in the browser, you will see an empty page. You can add webfonts, meta tags, or analytics to this file. The build step will place the bundled scripts into the <body> tag. To begin the development, run `npm start` or `yarn start`. To create a production bundle, use `npm run build` or `yarn build`. --> <script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo" crossorigin="anonymous"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.0/umd/popper.min.js" integrity="sha384-cs/chFZiN24E4KMATLdqdvsezGxaGsi4hLGOzlXwp5UZB1LY//20VyM2taTB4QvJ" crossorigin="anonymous"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.1.0/js/bootstrap.min.js" integrity="sha384-uefMccjFJAIv6A+rW+L4AHf99KvxDjWSu1z9VI8SKNVmz4sk7buKt/6v9KI65qnm" crossorigin="anonymous"></script> </body> </html>
Using react-bootstrap npm package
I prefer adding that using the react-bootstrap or reactstrap npm package so we’ll be looking at how to do so?
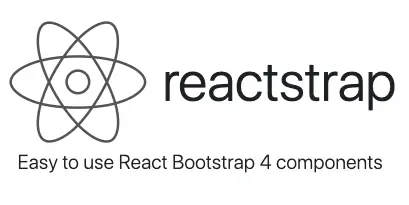
npm install react-bootstrap bootstrap@5.1.3
Now you have the bootstrap installed with this command.
The next step to use Bootstrap is to actually import elements and use them.
You can use Bootstrap directly on elements and components in your React app by applying the in-built classes just like we use in any other programming language. For eg:- Let’s take up a Button Element from Bootstrap.
import Button from 'react-bootstrap/Button'; // or import { Button } from 'react-bootstrap';
BootStrap React Examples
React bootstrap Button Component
Then let’s use this Button Component in jsx, just as we do in HTML file
<Button variant="primary">Primary</Button> <Button variant="secondary">Secondary</Button> <Button variant="success">Success</Button> <Button variant="warning">Warning</Button> <Button variant="danger">Danger</Button> <Button variant="light">Light</Button> <Button variant="info">Info</Button> <Button variant="link">Link</Button> <Button variant="dark">Dark</Button>

React bootstrap Card Component
Let’s see an example of Cards now. The card is a container, Card.Img is an Image element, Card.body takes in content in the card and inside the body, we have Title & More Text. You can give this variant too.
<Card style={{ width: '18rem' }}> <Card.Img variant="top" src="holder.js/100px180" /> <Card.Body> <Card.Title>Card Title</Card.Title> <Card.Text> Some quick example text to build on the card title and make up the bulk of the card's content. </Card.Text> <Button variant="primary">Go somewhere</Button> </Card.Body> </Card>
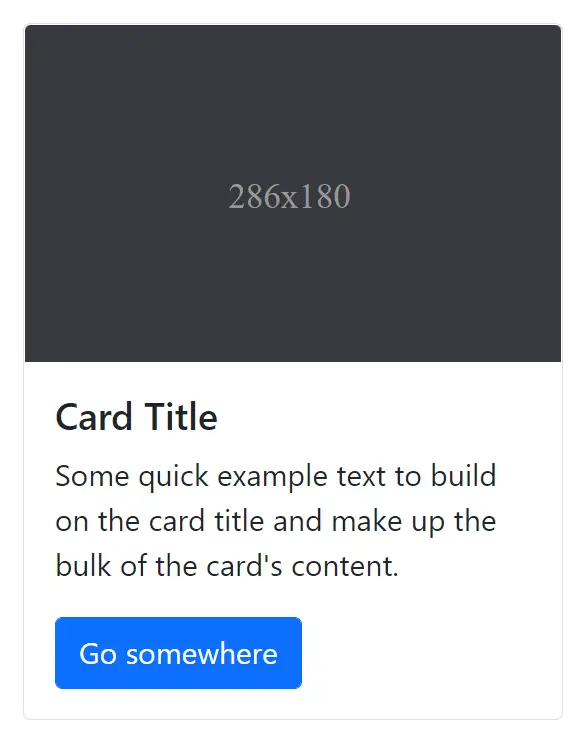
React bootstrap Alert Component
Let’s have a look at Alerts now that provides contextual feedback messages for typical user actions with the handful of available and flexible alert messages. These are the variants.
[ 'primary', 'secondary', 'success', 'danger', 'warning', 'info', 'light', 'dark', ].map((variant, idx) => ( <Alert key={idx} variant={variant}> This is a {variant} alert—check it out! </Alert> ));
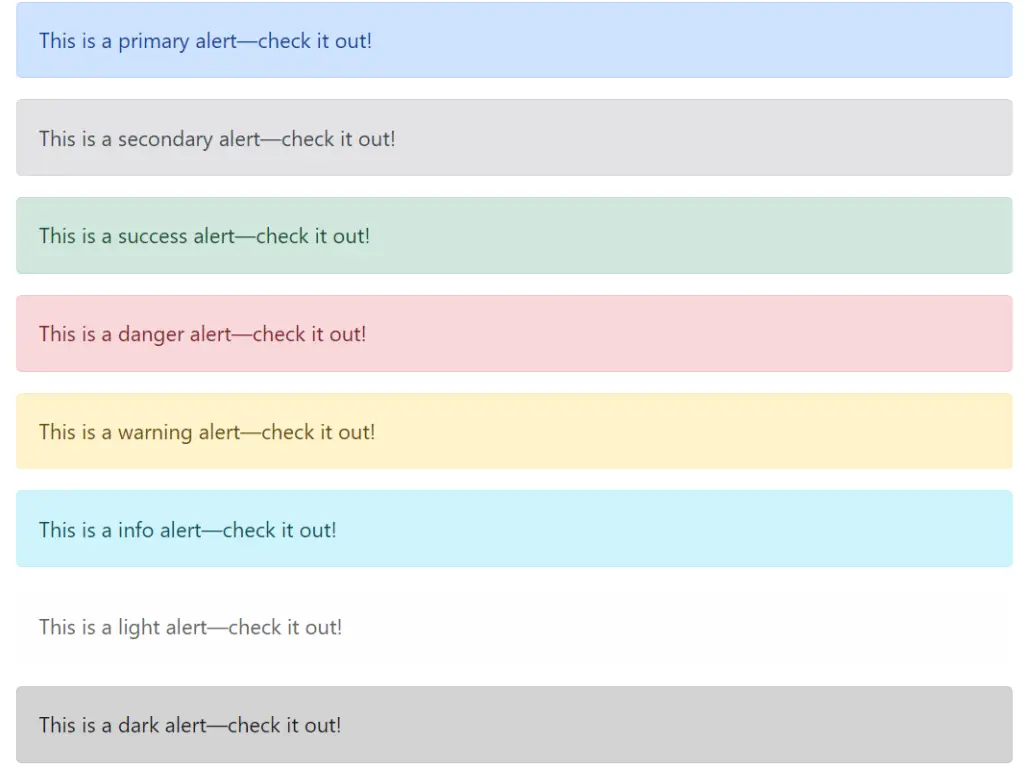
We can use more such components to make our work effective and rapid such as Pagination, Progress Bars, Popovers, Spinners, Breadcrumbs, Dropdowns, Inputs, Lists, Modals, etc. There are many!
In this article, we’ve explored a few different ways in which we can integrate Bootstrap with our React applications. We have also seen how we can use two of the most popular React Bootstrap libraries, namely react-bootstrap
and reactstrap
Don’t forget to share it with your connections 🙂