Hi Guys, Welcome to Proto Coders Point. In this article, we’ll look at how to create react custom hooks to fetch APIs in Reactjs using Axios.
Custom hooks in react to fetch APIs help you to make your work easier and rapid instead of importing and using Axios again and again in each file to integrate frontend and backend.
Tons of API calls in your web application result in a slow down of the web app. Due to which it doesn’t ensure user performance. So, using optimized and fewer API calls can help you get better with the scalability of the application.

Introduction to custom hooks in react and Axios React
What is a custom hook
We create a custom hook for reducing code and reusability. A custom hook is a JavaScript function that starts with “use” and that can call other hooks in react functional component. There are already inbuilt react hooks such as useEffect, useState, useHistory, etc. Here we’ll be optimizing API calls in the frontend by creating our own hook.
Now, What is Axios actually
Axios in react is an HTTP client for browser and Node.js based on JavaScript Promises. Axios react makes it easy to send asynchronous HTTP requests to REST endpoints and perform CRUD operations. It can be used with any framework or regular pure JavaScript.
What we are going to build
We’ll be building our custom react hook function named “useAxios”. You can name it anything related such as “useApi” or “useFetch” to create custom hook in react. This will make the usual repetitive API fetch code reusable.
We’ll be using this https://jsonplaceholder.typicode.com/users to Fetch users. This is just a fake and free API for testing.
You’ll see this once you click on the URL. This is written in JSON format.
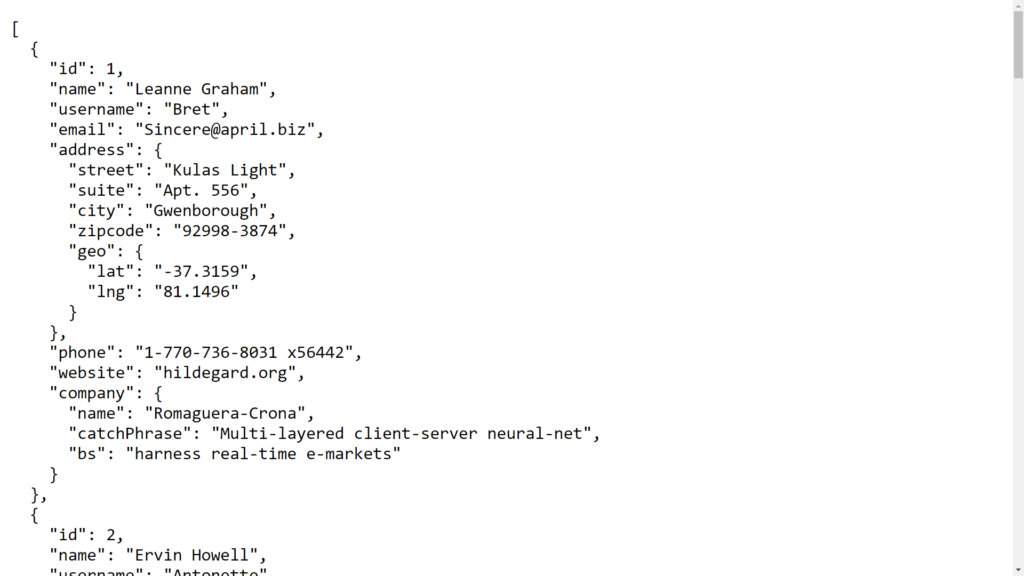
Let’s go with useAxios Approach!
That’s how you fetch API using Axios but this time we’re doing the same but in another custom file.
import { useState, useEffect } from 'react'; import axios from 'axios'; axios.defaults.baseURL = 'https://jsonplaceholder.typicode.com'; const useAxios = () => { const [response, setResponse] = useState(null); const [error, setError] = useState(null); const [loading, setLoading] = useState(false); const fetchData = async () => { setLoading(true); try { const res = await axios.get('/users'); setResponse(res.data); setError(null); } catch (err) { setError(err); } finally { setLoading(false); } }; useEffect(() => { fetchData(); }, []); return { response, error, loading }; }; export default useAxios;
If you’ll console.log the same stuff that we did above. You’ll see this result.
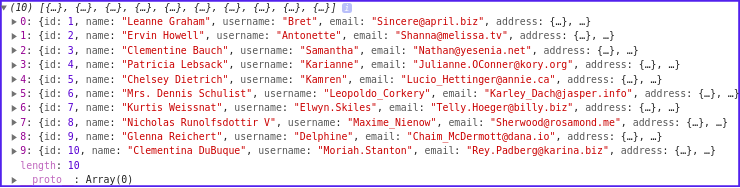
Then, App.js file will be written like this. For all the files that we’ll be fetching APIs in, we can use useAxios and make it reusable and worthy.
import useAxios from './useAxios'; const App = () => { const { response, error, loading } = useAxios(); return ( <div className="app"> {loading ? ( <div>Loading...</div> ) : ( <div> {error && error.message} {response && response?.map((item) => <div>{item.title}</div>)} </div> )} </div> ); }; export default App;
Now we have super clean and easy-to-understand optimized code with fewer lines. That’s the power of making custom hooks. You can also go ahead and make this hook more customizable by adding parameters or functions that will let you work even more faster. For now, I’ve kept it simple and understandable.
That’s all!
Make sure you share it with your developer friends and connections.
learn more about react
Tools and Resources to learn react.js