A java program for student to learn a simple bank account program in java using classes and object. A menu-driven java bank account code where a user can log in, Deposit Amount, Withdraw amount & check account balance, with proper customized Exception Handling.
Bank Account Java Program – Menu Driven Program
Here is my Java Project Structure, for better understanding the Process
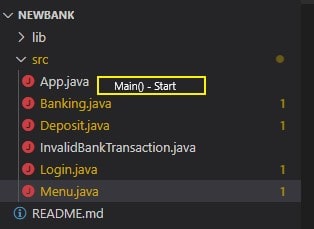
So as we are going to develop a project for bank transaction,( a bank account program in java using classes & object).
Here is source code on java bank account program
App.java
This is starting point of your java code i.e. main(). From here we are just creating an object of Banking class and by using the object i.e. b we are calling initiate() method of Banking class.
public class App { public static void main(String[] args) throws Exception { System.out.println("Hello, World!"); Banking b = new Banking(); b.initiate(); } }
Banking.java
In Banking class we have a int varible amount that is set to 1000 initially,
Banking class can perform various task such a Login, Get Balance, Deposit (add amount), Withdrawal available money, with proper exception handling, So for all this task, i have created the method as below.
- initiate() – used to login, it calls Login class for further process.
- getBalance() – it return available bank account balance.
- add(amt) – deposit the amount or update the balance(amount)
- withdrw(amt) – remove the amount from balance amount from bank account.
import java.util.Scanner; class Banking{ int amount = 1000; public void initiate() { Login login = new Login(); try{ login.acceptInput(); login.verify(); }catch(Exception e) { try{ login.acceptInput(); login.verify(); }catch(Exception f) { } } } public int getBalance(){ return amount; } public void add(int amt){ amount = amount + amt; System.out.println("Amount deposited Successfully"); System.out.println(" "); System.out.println("Total Balance: " +amount); System.out.println(" "); } public void withdrw(int amt){ System.out.println(" "); if(amount < amt) { InvalidBankTransaction invalidWithDraw = new InvalidBankTransaction("InValid Withdrawal Amount"); System.out.println(invalidWithDraw.getMessage()); }else{ amount = (amount - amt); System.out.println("Please Collect your " + amt +" Rupees"); System.out.println(" "); System.out.println("Total Balance: " +amount); System.out.println(" "); } } }
Login.java
To get Logged in i have a fixed ac number =1234 and ac password=9999, using which a use can login,
Login class has 2 method:
acceptInput() – used to ask n take input from user.
verify() – used to check if the login was successful or not successful. if successful then use the banking class to fetch balance and then show a menu-driven option to the user to select the menu.
if login do failed then show a proper message to a user by using the InvalidBankTransaction Customized Exception class.
import java.util.Scanner; class Login{ int ac_number = 1234; int ac_pass = 9999; int ac; int pw; public void acceptInput(){ Scanner scanner = new Scanner(System.in); System.out.println("Enter the account number:"); ac = scanner.nextInt(); System.out.println("Enter the Password:"); pw = scanner.nextInt(); } public void verify() throws Exception{ if(ac == ac_number && pw == ac_pass) { System.out.println("Login Successfull!"); Banking banking = new Banking(); System.out.println(" "); System.out.println("Your Balance is: "+banking.getBalance()+" Rupees"); System.out.println(" "); Menu menu = new Menu(); menu.showMenu(); }else{ InvalidBankTransaction loginfailed = new InvalidBankTransaction("Incorrect login credentials"); System.out.println(loginfailed.getMessage()); throw loginfailed; } } }
Menu.java
The java program is an example of a menu-driven program, using Menu class we are showing the menu option to the user.
Here we are showing menu item to the user and there is a swtich statement to go with the option selected by the user
For Example, we have 1 for Deposit, so when the user select 1 option then the deposit process executes likewise 2 and 3 are for withdrawal & check balance respectively.
import java.util.Scanner; class Menu{ int selectedOption; Banking banking = new Banking(); public void showMenu() { System.out.println("Please Select an option below:"); System.out.println("Press 1 to Deposit Amount."); System.out.println("Press 2 to Withdraw Amount."); System.out.println("Press 3 to View Balance"); System.out.println("Press any key to Exit"); System.out.println(" "); Scanner scanner = new Scanner(System.in); System.out.println ("Press any key:"); selectedOption = scanner.nextInt(); switch (selectedOption) { case 1: Deposit d = new Deposit(); int depamt = d.userInput(); banking.add(depamt); showMenu(); break; case 2: System.out.println("Please Enter the amount to withdraw:"); int withamt=scanner.nextInt(); banking.withdrw(withamt); showMenu(); break; case 3: System.out.println("Your Account Balance is "+banking.getBalance()+" Rupees"); System.out.println(" "); showMenu(); break; default: System.out.println("Transaction Ended"); System.exit(0); break; } } }
Deposit.java
When user select option 1 from menu Deposit class is been called where user is asked to enter the amount to be deposited.
Here is a check statement where if user enter negative amount then show a proper message using Exception Class.
If user enter currect amount then userInput() method will return the amt back to it’s object from where it was called.
import java.util.Scanner; class Deposit{ int amt= 0; public int userInput() { Scanner scanner = new Scanner(System.in); System.out.println("Enter the amount to be deposited: "); amt = scanner.nextInt(); if(amt<=0) { InvalidBankTransaction depositnegativeError = new InvalidBankTransaction("Invalid Deposit Amount"); System.out.println(depositnegativeError.getMessage()); userInput(); }else{ return amt; } return amt; } }
InvalidBankTransaction.java
So this is common Customized Exception class used to handle all the user errors.
class InvalidBankTransaction extends Exception { String errorMessage; public InvalidBankTransaction(String message) { errorMessage = message; } public String getMessage() { return errorMessage; } }
Output – Result of above java code for bank operation
Login 👇👇👇
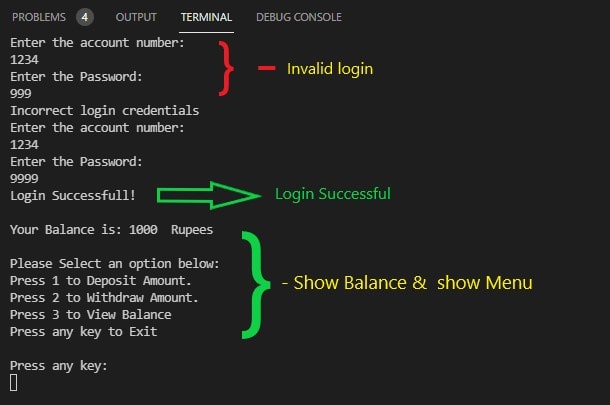
Deposit amount 👇👇👇
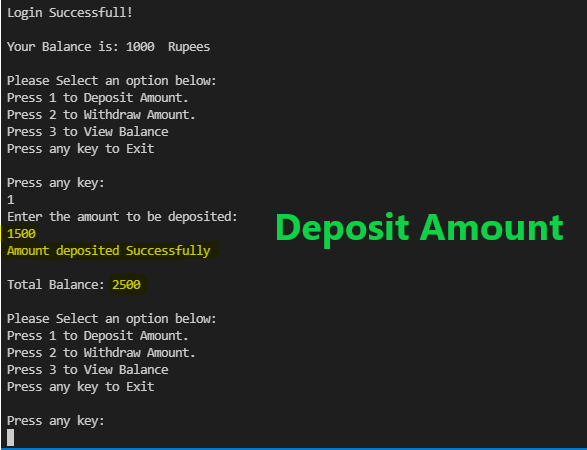
Show Balance 👇👇👇
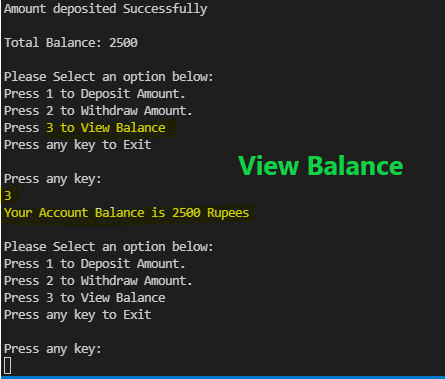
Withdrawal 👇👇👇
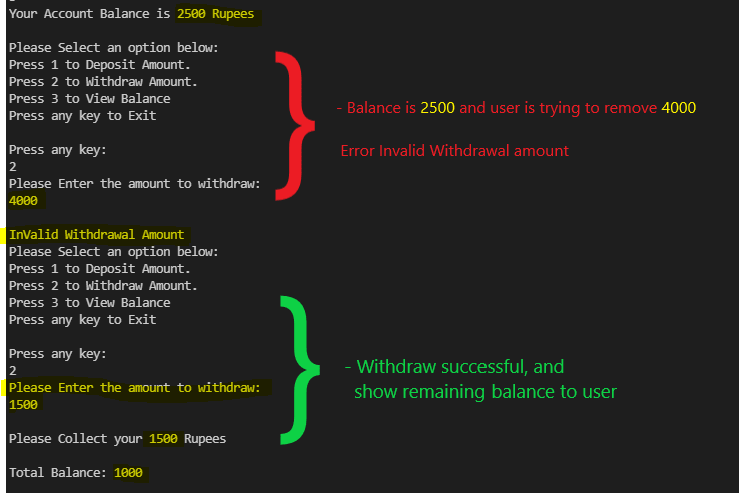