Hi Guys, welcome to proto coders point In this Flutter Tutorial will see above flutter photo view package library widget.
Flutter Photo View widget with zoomable image gallery
Photo View widget in flutter is a simple zoomable image or any content widget in flutter application development.
Using PhotoView widget on any widget view enable the widget images to become able to add zoom effect and a pan with user gestures such a rotate,pinch and drag gestures.
It also can show any widget instead of an image, such as Container, Text or a SVG.
This image viewer widget is super simple to use and play, Flutter zoom photo view widget is extremely customizable through the various options it provide and easy controllers.
For more details visit official site HERE
Let’s begin implementing this flutter widget in our project
Step 1: Create new Flutter project
Create a new Flutter Project in android-studio or any other Framework or open your existing flutter project
File > New > New Flutter project
Once your project is ready remove all the default dart code from main.dart file
and just copy paste the below code in main.dart
main.dart
import 'package:flutter/material.dart'; import 'package:photo_view/photo_view.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, //primary theme color ), home: MyHomePage(), //call to homepage class ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Photo View + Zoomable widget"), ), // your body code here ); } }
Step 2: Add Dependencies
Then, you need to add dependencies in your project
open pubspec.yaml file and under dependencies line add below
dependencies: photo_view: ^0.9.1
Step 3: import the photo view widget package
Once you have added photo_view dependencies in your project now you can use those packages into your project by just importing photo_view.dart library
import 'package:photo_view/photo_view.dart';
on the top of main.dart file add this import statement.
This allows you to make user of flutter zoomable image photoViewer library in main.dart file
Step 4: Create a folder(assets) and add path to asset folder in pubspec.yaml file
You need to create a assets folder and assign the path to be able to access images under assets folder.
Right click ( project ) > New > Directory
Name it as assets and then add some images under that folder.
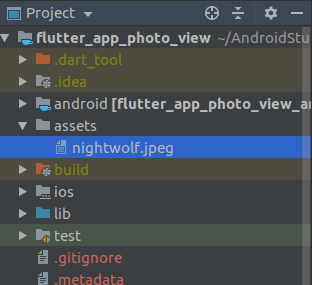
After you add images in assets folder open pubspec.yaml file to assign access path to assets folder for your project, So that your project can easily use that folder.
flutter: # The following line ensures that the Material Icons font is # included with your application, so that you can use the icons in # the material Icons class. uses-material-design: true assets: - assets/ # add the above assets exactly below users-material-----
Then, now the project is ready to make use of Flutter PhotoView zoomable image widget
Zoom image flutter basic code base
import 'package:flutter/material.dart'; import 'package:photo_view/photo_view.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, //primary theme color ), home: MyHomePage(), //call to homepage class ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Photo View + Zoomable widget"), ), // add this body tag with container and photoview widget body: Container( child: PhotoView( imageProvider: AssetImage("assets/nightwolf.jpeg"), )), ); } }
The above code will result you with:
Photo View Gallery
If you want to show more that 1 image in a form of slidable image gallery then you need to user PhotoViewGallery.dart package
import 'package:photo_view/photo_view_gallery.dart';
Copy paste the below code to in main.dart file
Flutter photoview gallery example
import 'package:flutter/material.dart'; import 'package:photo_view/photo_view.dart'; import 'package:photo_view/photo_view_gallery.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, //primary theme color ), home: MyHomePage(), //call to homepage class ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { final imageList = [ 'assets/nightwolf.jpeg', 'assets/lakeview.jpeg', 'assets/redsundet.jpeg', ]; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Photo View + Zoomable widget"), ), // add this body tag with container and photoview widget body: PhotoViewGallery.builder( itemCount: imageList.length, builder: (context, index) { return PhotoViewGalleryPageOptions( imageProvider: AssetImage(imageList[index]), minScale: PhotoViewComputedScale.contained * 0.8, maxScale: PhotoViewComputedScale.covered * 2, ); }, scrollPhysics: BouncingScrollPhysics(), backgroundDecoration: BoxDecoration( color: Theme.of(context).canvasColor, ), loadingChild: Center( child: CircularProgressIndicator(), ), ), ); } }
Result :
Conclusion
In the Flutter Tutorial we learned how can we display an image or photo with customizable, rotatable image, and zoomable image in flutter using the PHOTO VIEW LIBRARY.
The Flutter PhotoView Gallery is one of the best way to show a zoomable image in flutter application.