Hi Guys, Welcome to Proto Coders Point. In this article will learn how to secure mongodb by creating auth user to remote access mongodb.
As you know, Mongodb is described in NoSQL database, it does not follow any relational DB structure, Instead, it stores data in JSON document.
When you install mongodb on ubuntu server, By default, mongodb remote connection security don’t have any authentication enabled, means anyone with you HOST IP can easily get access to your mongoDB services & perform CRUD operations without any mongodb authentication. which is not a secured way to integrate mongod database globally.
In this tutorial, We will cover how to secure mongodb authentication, so that only the user with mongodb auth credential can access DB.
Topic covered – Auth user creation in Mongodb
- Create MongoDB Super administrative user.
- Create user who can access db with read/write specific database.
- Create a user who has only read access in Mongodb.
Login into server using WINSCP & start putty terminal.
if you have problem, go through below video tutorial
1. Enabling mongodb security Authentication
To enable mongodb security, you need to edit mongod.conf
file.
1. Open mongod.conf
using nano editor
sudo nano /etc/mongod.conf
2. under security section add authorization : enabled
security:
authorization: enabled
Note: Here, security should not have space at start, & authorization should have 2 space at beginning below security.
refer screenshot for clear understanding
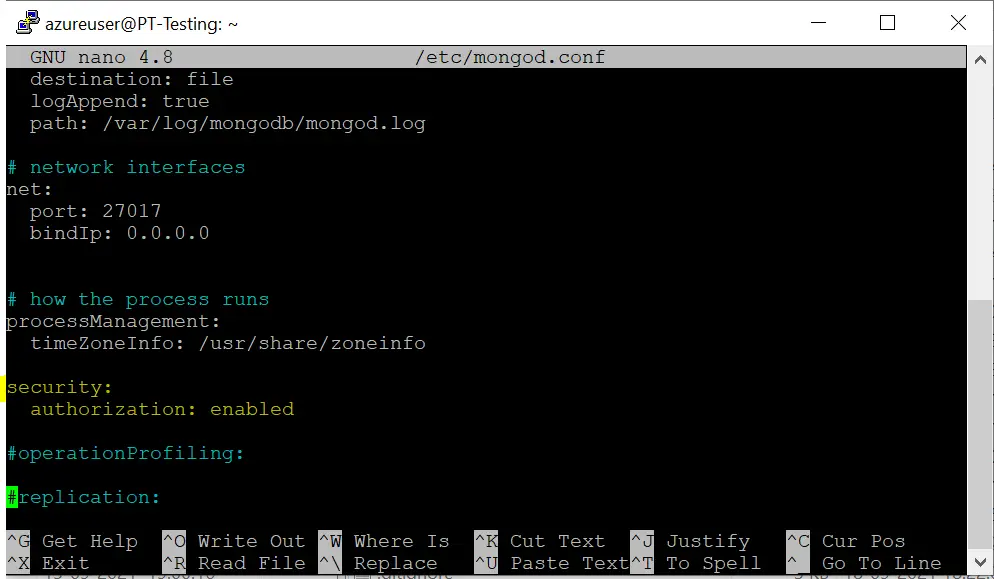
After making mongodb auth enable save mongod.conf file & close it.
2. Restart mongod service & check status
After changing mongodb configuration, you need to restart mongod service, so that auth change get applied.
run below cmd
sudo systemctl restart mongod
then, check the status of mongod service,
sudo systemctl status mongod
If restart was successful, you will see active status as active(running)
Therefore, we have successfully enabled mongodb authorization globally. but we can’t access it right now as auth is been enabled, we need to create users to access database using credential.
MongoDb Auth user Creation
Follow below steps/cmd to create Auth user to access your mongodb database server, So that only the user with auth credential will be able to access DB.
use WinSCP to login into server & start putty terminal and then start mongo shell
mongosh
1. Create Admin User
Let’s create a super admin user, who will have access to read write any database in mongod
run below mongod queries to create users
use admin
mongodb create admin user
db.createUser( { user: "root", pwd: "root1234", roles: [ { role: "root", db: "admin" }, "readWriteAnyDatabase" ] } )
Therefore, super admin user is created with full read write any database access in mongod.
Restart mongod again to apply changed
sudo systemctl restart mongod
Now, goto mongoDB compass & connect using admin user credential
connection URI:
mongodb://root:root1234@< HOST IP >:27017
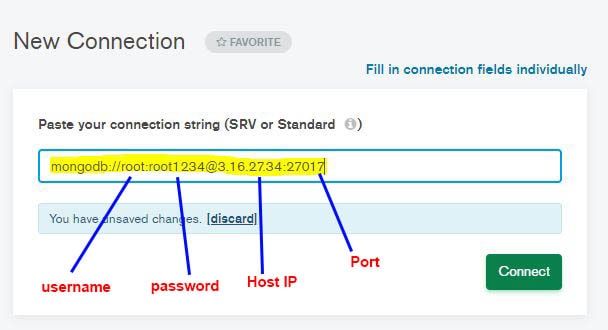
2. Create user to access specific database
Now, let’s create a user who will have access to read/write only specific db assigned to him.
Only super user can assign and create new user to handle db, So first we need to use super admin credential to login, follow steps below
use admin
db.auth("root", "root1234")
now switch to database where you want to create user
use rajatdb
-> replace rajatdb with your dbname.
now create a user to access specific DB.
db.createUser( { user: "rootUser", pwd: "rootUser1234", roles: [ { role: "readWrite", db: "rajatdb" } ] } )
Above user can only access rajatdb, the role assigned to this user is readWrite.
now, run restart mongod cmd.
Now, go back to mongoDB compass & connect using user credential we created.
connection URI: mongodb://rootUser:rootUser1234@< HOST IP >:27017/rajatdb?authSource=rajatdb
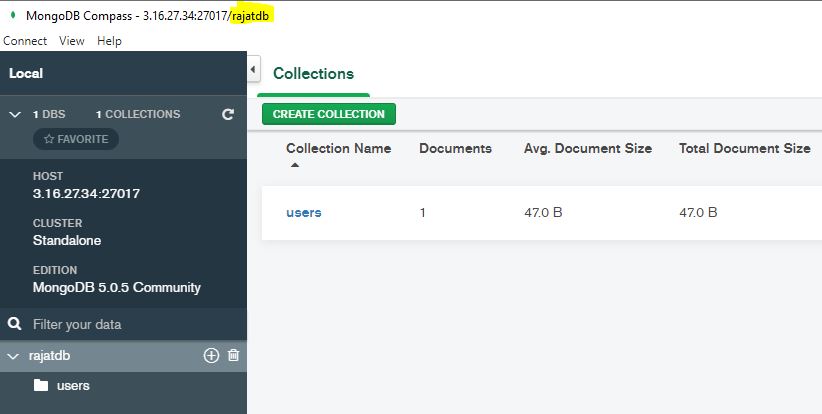
3. Create a user who has only read access to specific database.
Now, let’s create a user which can only read/view the data but can’t make changes.
repeat the same step
auth as super
use admin
db.auth("root", "root1234")
now switch to database where you want to create user
use rajatdb
db.createUser( { user: "ReadUser", pwd: "read1234", roles: [ { role: "read", db: "rajatdb" } ] } )
Here the role assigned to this user is read, so he can only view & read data from database.
now, run restart mongod cmd.
Now, go back to mongoDB compass & connect using user credential we created.
connection URI: mongodb://ReadUser:read1234@< HOST IP >:27017/rajatdb?authSource=rajatdb