Hi Guys, Welcome to Proto Coders Point. In this Android tutorial, we will learn how to create a custom dialog box with a floating image app logo.
Sometimes a simple & default android dialop popup is not suitable with our app UI, In that case we need to create a custom alert dialog layout design to show as a popup to a user.
Example: Check the below image, this is what we will achieve at the end.
A android custom popup dialop example with a floating imageview sightly out of dialog as shown in below screenshot.
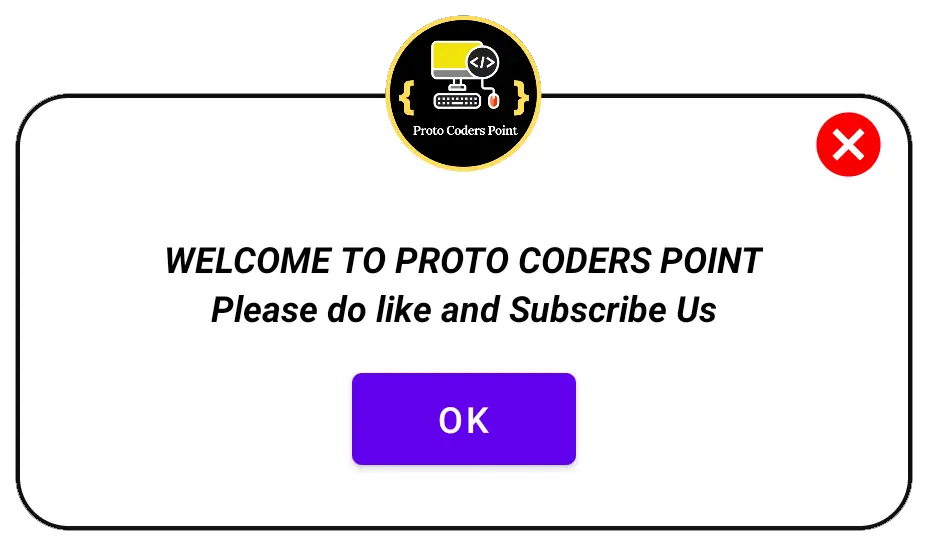
Video Tutorial
Below are steps to achieve above android alert dialog.
Step 1: create a background for custom dialog layout
Create a XML file: dialog_bg.xml in drawable folder and copy paste the below code in it.
<?xml version="1.0" encoding="utf-8"?> <inset xmlns:android="http://schemas.android.com/apk/res/android" android:insetLeft="5dp" android:insetTop="5dp" android:insetBottom="5dp" android:insetRight="5dp" > <shape android:shape="rectangle"> <corners android:radius="20dp"/> <solid android:color="@color/white"/> <stroke android:width="5dp" android:color="#FFF"/> </shape> </inset>
The above code is just to give a corner radius, solid color, and stroke to custom_dialog.xml background.
Step 2: Create a vector image(cancel image) and copy a logo image in drawable
Create vector assets image Right click on drawable -> New -> Vector Assets & choose a cancel button.
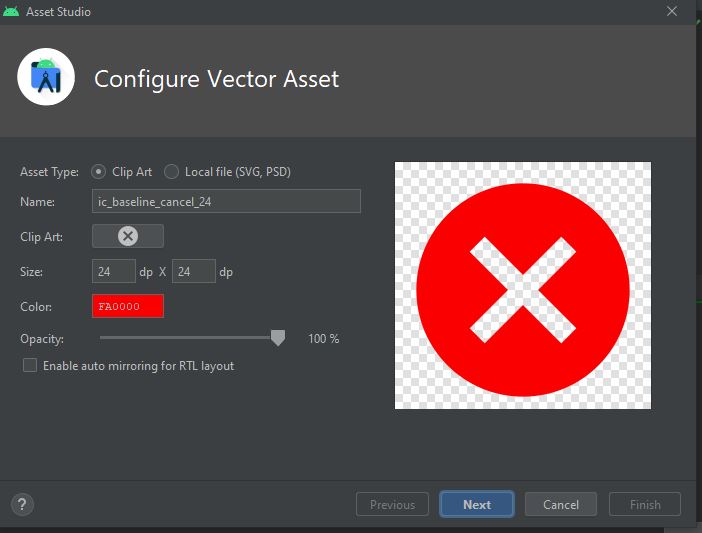
We need a logo image file to show in dialog popup as a floating app logo, So just copy your app logo in drawable file.
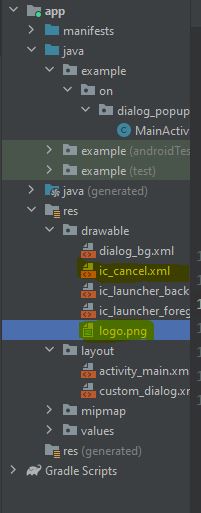
Step 3: Creating a custom_dialog.xml layout file under layout folder
Now a main custom alert dialog layout design custom_dialog.xml, Under layout folder create a XML file custom_dialog.xml and copy below layout code and paste in it.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <LinearLayout android:background="@drawable/dialog_bg" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginLeft="20dp" android:orientation="vertical" android:layout_marginRight="20dp" android:layout_marginTop="30dp"> <ImageView android:id="@+id/cancel_button" android:layout_width="30dp" android:layout_height="30dp" android:background="@drawable/ic_cancel" android:layout_centerHorizontal="true" android:layout_marginRight="10dp" android:layout_marginTop="5dp" android:layout_gravity="right"/> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="20dp" android:layout_marginTop="20dp" android:layout_marginLeft="20dp" android:layout_marginRight="20dp" android:orientation="vertical"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:text="WELCOME TO PROTO CODERS POINT" android:textColor="#000" android:textStyle="bold|italic" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:text="Please do like and Subscribe Us" android:textColor="#000" android:textStyle="bold|italic" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="OK" android:gravity="center" android:layout_gravity="center" android:layout_marginTop="10dp"/> </LinearLayout> </LinearLayout> <ImageView android:layout_width="70dp" android:layout_height="70dp" android:layout_centerHorizontal="true" android:background="@drawable/logo" /> </RelativeLayout>
This code defines different views inside a custom dialog layout, it has 2 imageview, one for App logo and another for cancel vector image and 2 TextView and a ‘OK’ button.
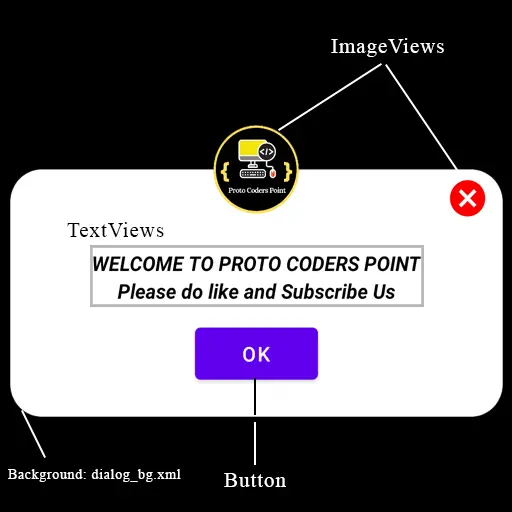
Step 4: activity_main.xml add a button
In activity_main.xml file will add a button, when a user clicks on that button will show android dialog.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" android:gravity="center"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Show Dialog" android:id="@+id/showdialog" /> </LinearLayout>
Step 5: MainActivity.xml on click show alert dialog in android
On Button click create a custom dialog in android and show it to the user.
MainActivity.java
package example.on.dialog_popup; import androidx.appcompat.app.AlertDialog; import androidx.appcompat.app.AppCompatActivity; import android.graphics.Color; import android.graphics.drawable.ColorDrawable; import android.media.Image; import android.os.Bundle; import android.view.LayoutInflater; import android.view.View; import android.widget.Button; import android.widget.ImageView; public class MainActivity extends AppCompatActivity { Button showdialog; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); //find the view showdialog = (Button)findViewById(R.id.showdialog); // when clicked showdialog.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { ImageView cancel; //will create a view of our custom dialog layout View alertCustomdialog = LayoutInflater.from(MainActivity.this).inflate(R.layout.custom_dialog,null); //initialize alert builder. AlertDialog.Builder alert = new AlertDialog.Builder(MainActivity.this); //set our custom alert dialog to tha alertdialog builder alert.setView(alertCustomdialog); cancel = (ImageView)alertCustomdialog.findViewById(R.id.cancel_button); final AlertDialog dialog = alert.create(); //this line removed app bar from dialog and make it transperent and you see the image is like floating outside dialog box. dialog.getWindow().setBackgroundDrawable(new ColorDrawable(Color.TRANSPARENT)); //finally show the dialog box in android all dialog.show(); cancel.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { dialog.dismiss(); } }); } }); } }
Recommended Android and Flutter Posts
Android Alert dialog Box with list of options in it.