Hi Guys, Welcome to Proto Coders Point.
In this dart programming article, we will learn what is a palindrome, A dart Programs on “how to check if a number is palindrome”, “check if string is palindrome or not”.
What is a Palindrome – number, string
Palindrome meaning(defination), A Number or a string when reversed looks same and pronounced same as it was before reversing.
For Example: 656 when reversed will be same, ( it’s a palindome number), 657 when reversed is 756 so ( it’s not a palindrome number). like wise 252,25252,49694.
String Example for palindrome: NITIN, MADAM, ABBA, MAM, STRAW WARTS, DAMMIT IM MAD.
Palindrome Number algorithm – Code Work flow
- Get a number to check if palindrome or not.
- Store the same number, in temp variable.
- Reverse number using mathematical operation or string methods.
- Now compare temp variable with the reversed number value.
- If both number match ‘Then it’s a palindrome number’
- Else ‘Not a Palindrome Number’
Now Let’s see a palindrome number program in dart language.
void main(){ int reminder, sum =0, temp; int number = 54545; temp = number; // a loop to reverse a number while(number>0) { reminder = number % 10; //get remainder sum = (sum*10)+reminder; number = number~/10; } if(sum == temp) { print('Its A Palindrome number'); }else{ print('Its A Not Palindrome number'); } // StringNumber(); }


Palindrome program in dart programming language (Another way)
Here in below dart program, A user can input either a number or string to check for palindrome or not.
Here we are accepting a input from a user and then reverse the string and then matching them.
How to reverse a string in dart
stringValue!.split('').reversed.join('')
import 'dart:io'; void main(){ print('Enter Words or number'); // User enter a string or a number String? original = stdin.readLineSync(); // then we will reverse the input String? reverse = original!.split('').reversed.join(''); // then we will compare if(original == reverse) { print('Its A Palindrome'); }else{ print('Its A Not Palindrome'); } }
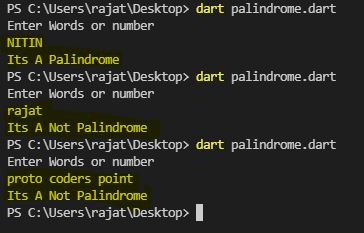
Recommended dart basic programs
Generate random number in dart