Hi Guys, Welcome to Proto Coders Point, In this dart tutorial we will learn, What is a prime number, check if a given number in prime or not & a dart program to print prime number from 1 to N.
What is a Prime Number?
A Prime Number in math are the numbers that are greater then 1 & are divisible by 1 or itself.
In other Words, A number that can’t be divided by any other number, rather then 1 or itself.
Prime Number Examples
Eg:
5 => 5 can’t be divided by any other numbers.
3 => 3 can’t be divided by any other numbers.
7 => 7 can’t be divided by any other numbers.
13 => 13 can’t be divided by any other numbers.
Not a Prime Number Examples
Eg:
4 => 4 is divisible by 2 so it not a prime number.
6 => 6 is divisible by 2 so it not a prime number.
8 => 8 is divisible by 2 so it not a prime number.
10 => 10 is divisible by 2 so it not a prime number.
If you observe you can see that, all the even number are not a prime number, except 2.
NOTE: 0 and 1 are always said to be not a prime number.
How to check if a number is prime or not in dart
Let’s write dart programs to check if a number is prime or no.
1. Dart Program to check a number prime or no
In this dart program, we will initialize a Integer with a number value & then check if that number is prime or no.
void main(){ int i,m=0,flag=0; int num = 5; m=num~/2; for(i = 2;i<=m;i++){ if(num%i == 0){ print('$num is not a prime number'); flag=1; break; } } if(flag==0){ print('$num is prime number'); } }
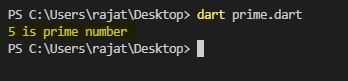
2. check prime number using method in dart program
void main(){ // call method by passing a integer value to it checkPrime(5); checkPrime(8); checkPrime(13); checkPrime(76); checkPrime(7); } // a method that checks prime or not prime void checkPrime(int num){ int i,m=0,flag=0; m=num~/2; for(i = 2;i<=m;i++){ if(num%i == 0){ print('$num is not a prime number'); flag=1; break; } } if(flag==0){ print('$num is prime number'); } }
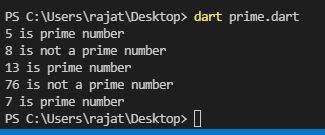
3. Prime number dart program – take input from user
So now in below code, the number to be checked is not predefined, Here the dart code will ask the user to enter a number to be checked whether it a prime or not
import 'dart:io'; import 'dart:math'; void main(){ print('Enter number to check prime or no'); int? num = int.parse(stdin.readLineSync()!); print('$num'); if(CheckPrime(num)){ print('$num is a prime'); }else{ print('$num is not a prime'); } } bool CheckPrime(int num){ if(num<=1){ return false; } for (int i = 2;i<=sqrt(num);i++){ if(num%i == 0){ return false; } } return true; }
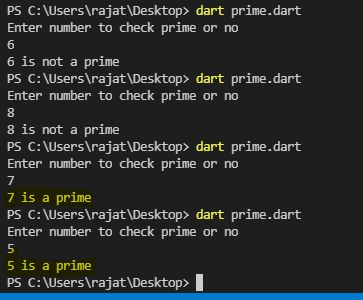
4. Dart program to print prime number from 1 to N
Now let’s write a dart program to print all the prime numbers from 1 to N.
import 'dart:io'; import 'dart:math'; void main(){ print('Enter Nth'); int? N = int.parse(stdin.readLineSync()!); print('----------------------------'); for(int i=2;i<= N;i++){ checkPrime(i); } } void checkPrime(int num){ int i,m=0,flag=0; m=num~/2; for(i = 2;i<=m;i++){ if(num%i == 0){ flag=1; break; } } if(flag==0){ print('$num'); } }
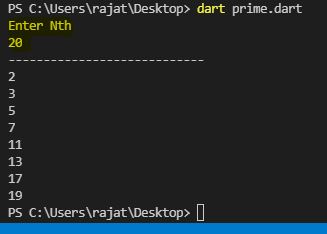
Similar dart programs to learn dart language
Generate Random number in dart
ArmStrong number program in dart